Question
You are given a file named hw02q3.c. All instructions are given in the form of comments in the file. You are to run the file
You are given a file named hw02q3.c. All instructions are given in the form of comments in the file. You are to run the file in both GCC and Visual Studio. Observe the outputs and make changes as asked. Please read all instructions very carefully, then complete and submit the updated file as hw02q3.c.
#include
// CSE 240 Spring 2021 Homework 2 Question 3 (25 points) // Note: You may notice some warnings when you compile in GCC or VS, that is okay.
#define ABS(x) (((x) < 0) ? -(x) : (x))
#define polyMacro(a, b) (a*a + 5*a- 4*a*b + b*b)
int polyFunc(int a, int b) { return (a * a + 5 * a - 4 * a * b + b * b); }
// Part 1: // We want to pass the value of 6 to ABS and expect the result of ABS to be 6, why is the result 7? Correct the error (5 points) void part1(int x) {
int result; result = ABS(++x);
printf("absm(6) = %d ", result);
// Why did this error occur? Please provide the answer in your own words below. printf("Explanation: ________ "); // (2.5 points) }
// Part 2: // We want to pass incremented values of x and y to the macro and function to compare their outputs in VS and GCC. // Run this program in Visual Studio(VS) and then again in GCC. Fill the blanks below with the output values for polyFunc and polyMacro. // Then correct/edit this function so that polyFun and polyMacro produce same correct output of 22. // (5 points) // void part2(int x, int y) { // increment before passing to macro int x_copy = x, y_copy = y;
printf("polyFunc(x, y) = %d polyMacro(x, y) = %d ", polyFunc(++x, ++y), polyMacro(++x_copy, ++y_copy));
// Replace the 4 blank spaces below with the actual output observed when running the code in VS and GCC. // The blanks should have the answers of unedited program. Keep the answers in blanks as they were, after editing the program. printf("In VS : the result of polyFunc = __ and polyMacro = __ "); // (5 points) printf("In GCC: the result of polyFunc = __ and polyMacro = __ "); // (5 points)
// Explain in a short sentence why VS and GCC could possibly produce a different value for the same program and for the same input. printf("Explanation: ___ "); // (2.5 points) }
// Do not edit main() int main() { int x = 5, y = 1;
printf("Part 1: "); part1(x); printf("Part 2: "); part2(x, y);
while (1); // needed to keep console open in VS return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
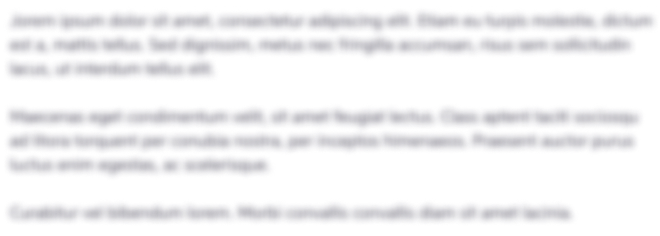
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started