Question
You are hired as a consultent at Automatic Robot Maintenance (ARM). They have many years of experience in robot technology and cybernetics. Recently ARM landed
Â
You are hired as a consultent at Automatic Robot Maintenance (ARM). They have many years of experience in robot technology and cybernetics. Recently ARM landed a new contract for maintaining a large field of solar panels. Close to the solar panels there is a garbage disposal plant and some trash has a tendency to cover and dirty the solar panels, such that they do not produce any electricity. The company who owns the solar panels has constructed a system to detect if solar panels are recieving less light so that they can check for trash covering them. However, they have become tired of manually cleaning the solar panels and has therefore hired ARM to automate this task. The robots have been programmed to move on the solar panel field and to clean the solar panels, but currently it is taking too long to move the robots to where they need to be.
ARM is in need of an algorithm to choose the correct robots to clean which solar panels and to position the robots so that they can execute a cleaning job as soon as the system detects one. ARM gets payed extra for each solar panel they clean, but lose money for each minute a solar panel is not operational due to being dirty.
Code
You are given a framework which includes:
- Location.java - describes a location using double valuesxandy
- Job.java - describes a job generated by the alert system which monitors if the solar panels are generating electricity. A job object includes:
- Location location- describes where the solar panel is located
- double time- denotes the time when it was discovered that the solar panel stopped producing electricity
- int robotsNeeded- denotes the number of robots required for cleaning the solar panel
- Robot.java - represents a robot for cleaning. Set of methods controlling the robot:
- getLocation()- get last knownLocationof the robot
- getJob- get the currentJobassigned to the robot
- move(Job job)- assigns theJobto the robot. Robot moves towards the location of the job. Aborts any previous commands
- move(Location location)- robots moves towards the givenLocationand awaits further orders. Aborts any previous commands
The packageinf102.h21.systemcontains code for simulating the environment of robots and solar panels.You do not have to look at this code.
The packageinf102.h21.generatorsis used to generate input for the system. Each generator constructs input of robots and solar panels placed around the field. To visualize the input runVisualizer.java. You will see a screen of white and red dots. White dots are robots and red dots are jobs that need to be executed (solar panels that need cleaning). Visualizing the input might help you devise your own strategy in task 3.
Task 1 - RandomStrategy
ImplementRandomStrategy, a strategy which selectskrandomrobots out of the available robots to execute the incoming cleaning jobs. The robot you choose must not be busy with another job.
Analyze the runtime ofassignRobots()inAbstractStrategy. Is there a way to improve the runtime of this method?
randomstrategy i need to implement by fill in selectRobots and getName
package inf102.h21.management; import java.util.List; public class RandomStrategy extends AbstractStrategy { @Override protected List selectRobots(Job job) { return null; } @Override public String getName() { return "Random strategy"; } }
abstractstrategy:
package inf102.h21.management; import java.util.ArrayList; import java.util.LinkedList; import java.util.List; import java.util.Queue; public abstract class AbstractStrategy implements IStrategy { /** * List of all robots, both available and occupied */ protected List robots; protected List available; /** * List of jobs not yet executed */ protected Queue backLog; public AbstractStrategy() { backLog = new LinkedList(); } @Override public void registerRobots(List robots) { this.robots = new ArrayList(robots); available = new ArrayList<>(robots); } @Override public void registerNewJob(Job job) { backLog.add(job); doJobs(); } @Override public void registerJobAsFulfilled(Job job, List robots) { available.addAll(robots); doJobs(); } /** * Finds jobs in backLog and assigns robots */ protected void doJobs() { while (!backLog.isEmpty()) { Job job = selectJob(); List selected = selectRobots(job); if(assignRobots(selected, job)) removeJob(job); else break; } if(backLog.isEmpty()) moveFreeRobots(); } /** * Selects a Job from the list of available jobs * Currently selects the job at the top of the list * * @return most appropriate job */ protected Job selectJob() { return backLog.peek(); } protected void removeJob(Job job) { if(backLog.peek().equals(job)) backLog.poll(); else backLog.remove(job); } /** * Select robots for the job. Should select robots most appropriate for the job. * * @param job - The job to select robots for * @param available - The Robots to select among * @return return list of selected robots if the job can be executed, else return empty list */ protected abstract List selectRobots(Job job); /** * When a Robot is not assigned to a Job it is just waiting * We can then position these robots such that when a new job comes in * the robots are already close to the job. */ protected void moveFreeRobots() { // TODO: This method could be suited for task 3 } /** * Sends the selected Robots to their Job * * @return true if robots assigned to job, false if not */ boolean assignRobots(List selected, Job job) { if (selected == null) return false; if (selected.isEmpty()) return false; boolean canDo = selected.size() >= job.robotsNeeded; for(Robot r : selected) { if(r.isBusy()) { System.out.println("You selected a robot that was busy."); canDo = false; } } if(canDo) { for(Robot robot : selected) { robot.move(job); available.remove(robot); } } else { for(Robot r : selected) { if(!r.isBusy()) { r.move(job.location); } } } return canDo; } /** * Returns list of free robots * * @return list of all available robots */ public List getAvailableRobots(){ return available; } }
and here is robotinfo:
package inf102.h21.system; import inf102.h21.management.Job; import inf102.h21.management.Location; /** * This class stores information about a robot needed by the Simulation. * Each Robot has a target they are moving towards, if target == null * the robot is awaiting orders. * The simulation is updating Location irregularly and the last update is * stored in lastUpdateTime and lastKnownLocation * * * */ public class RobotInfo { public static final double robotSpeed = 1d; // How far each robot moves in a unit of time public final int robotId; private Job assignedJob; private double lastUpdateTime; private Location lastKnownLocation; private Location target; private int eventId; private boolean waitingToComplete; /** * Constructor creating a RobotInfo with default * @param robotId * @param start */ public RobotInfo(int robotId, Location start) { this.robotId = robotId; this.assignedJob = null; lastUpdateTime = 0d; lastKnownLocation = start; eventId = 0; target = null; } /** * @return the job this Robot is assigned to do, null if robot is free */ public Job getAssignedJob() { return assignedJob; } /** * This method is called to add information that this Robot * has been assigned a Job to do and is moving towards. * If this Robot already was assigned to do another Job that Job is aborted * if assignedJob == null it means the robot is waiting for orders * @param job - The Job this Robot should do * @return The Job this Robot had previously */ public Job assignJob(Job job) { Job previousJob = assignedJob; assignedJob = job; if(job == null) target = null; else target = job.location; eventId++; return previousJob; } /** * Sends robot to a new location. * If input is null it means stop the robot and await new orders * @param location */ public void setLocation(Location location) { target = location; //if input is null it means stop robot and wait if(location == null) { eventId++; } //abandon job and go to new location if(assignedJob != null && !assignedJob.location.equals(location)) { assignedJob = null; eventId++; } } /** * Moves the robot to the position it will be at currentTime * Robot can be standing still waiting, reaching the target * before currentTime or still be moving at currentTime. * If robot already has a job and location sends it to a different location * the robot will abandon the job * * @param currentTime */ public void updatePosition(double currentTime) { //update time double deltaTime = currentTime - lastUpdateTime; if(deltaTime<0) throw new IllegalArgumentException("Can only update to a later time."); lastUpdateTime = currentTime; // No target if (target == null) { return; // Robot is stationary waiting for orders or for job to complete } double distance = lastKnownLocation.dist(target); double deltaDistance = deltaTime*robotSpeed; // Will reach target if (distance-0.0001 <= deltaDistance) { lastKnownLocation = target; target = null; return; } // Move some distance Location newLocation = new Location( lastKnownLocation.x + (target.x-lastKnownLocation.x)*deltaDistance/distance, lastKnownLocation.y + (target.y-lastKnownLocation.y)*deltaDistance/distance); //System.out.println("Moved from "+lastKnownLocation+" to "+newLocation); lastKnownLocation = newLocation; } /** * Last known location will always be the location the robot was at lastUpdateTime */ public Location getLastKnownLocation() { return lastKnownLocation; } /** * Returns the eventId counter for this robot. * Each time this robot changes target this counter is increased * @return */ public int getEventId() { return eventId; } }
and robot:
package inf102.h21.management; import inf102.h21.system.RobotStateManager; /** * Robot able to move around performing jobs. * This class keeps track of the position and activity of a Robot. * It also works as a communcation interface where Strategy implementations * can send commands through the robot to the system modelling the Simulation. * * For the runtime information N: numberOfRobots M: numberOfJobs * * * */ public class Robot { private RobotStateManager state; private final int id; /** * Contructor for creating a new robot. * All robots are created by the system, * there should be no need to create new robots * * @param id * @param state */ public Robot(int id, RobotStateManager state){ if (state == null) throw new NullPointerException(); this.id = id; this.state = state; } /** * This method tells the robot to move towards a Job location * If the same robot has received previous move commands that are not completed * these commands will be cancelled. * Once a move command is given the Robot will immediately find the optimal route to the job location. * Robots always move in a straight line between the current location and their target. * * runtime: O(log M) * * @param job - the job you want the robot to focus on */ public void move(Job job) { // System.out.println(this+" moving to "+job); state.move(id, job); } /** * This method tells the robot to start moving towards a location * The Robot will continue to move towards this location until * either it reaches the location or it gets stopped by another command. * * If the robot had previously received commands that were not yet complete * these commands will be cancelled. * * runtime: O(log M) * * @param location */ public void move(Location location) { //System.out.println(this+" moving to "+location); state.move(id, location); } /** * Gets the last known position of the robot * * runtime: O(1) * * @return */ public Location getLocation() { return state.getPosition(id); } /** * The Robot is only able to keep orders for one Job at the time. * The Robot is continuously moving towards the location of that Job * and once reached there it waits until the job is done. * When the job is done the system sets the job to null indicating * that the robot is free to move. * * If robot is moving towards a job or is waiting for a job to complete that job will be returned, * if robot is either moving to a point not related to a job or waiting for orders null is returned * * runtime: O(1) * * @return null if robot is free, otherwise the job this robot is working towards. * */ public Job getJob() { return state.getTarget(id); } /** * Check if the robot is busy with a job * * runtime: O(1) * * @return true if the robot is busy, false otherwise */ public boolean isBusy() { return getJob()!=null; } @Override public String toString() { return "Robot "+ id; } }
location:
package inf102.h21.management; /** * Location represents a point of interest where a robot might go to do work. * All fields of Location are finite meaning a Location can not be changed once it is created * * * */ public class Location{ public final double x, y; public Location(double x, double y){ this.x = x; this.y = y; } /** * Computes the Eucleadian distance between this location and the given location. * @param l - given location * @return - The distance between the points as a double */ public double dist(Location l) { return Math.sqrt((l.x -x)*(l.x -x) + (l.y - y)*(l.y -y)); } @Override public int hashCode() { final int prime = 31; int result = 1; long temp; temp = Double.doubleToLongBits(x); result = prime * result + (int) (temp ^ (temp >>> 32)); temp = Double.doubleToLongBits(y); result = prime * result + (int) (temp ^ (temp >>> 32)); return result; } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null) return false; if (getClass() != obj.getClass()) return false; Location other = (Location) obj; if (Double.doubleToLongBits(x) != Double.doubleToLongBits(other.x)) return false; if (Double.doubleToLongBits(y) != Double.doubleToLongBits(other.y)) return false; return true; } @Override public String toString() { return "("+x+","+y+")"; } }
please let me know if you need other classes to help me
Step by Step Solution
3.45 Rating (168 Votes )
There are 3 Steps involved in it
Step: 1
Sure Heres an implementation of the RandomStrategy that fills in the missing methods package inf102h...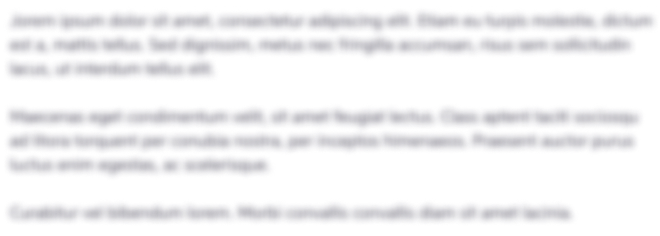
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started