Question
You are hired to determine eligibility of an applicant for auto insurance company. Using JavaScript, you are to makea console-based application as per the specifications
You are hired to determine eligibility of an applicant for auto insurance company. Using JavaScript, you are to makea console-based application as per the specifications below.
At the top of your JavaScript file, make a function called findEligibility(). This function must accept four parameters:
- name - applicant name (string)
- age - applicant age(number)
- ticket - whether the applicant has received the speeding ticket in past or not(Boolean)
- course - whether the applicant has taken driving lessons before or not(Boolean)
Thefunctionmustdisplayappropriatemessageinoutput thatitischeckingtheeligibilityforthegivendetails. For Boolean values, you should display "Yes" in the output if the value is true; "No" otherwise. Check the sampleoutput.
The function should then determine the eligibility of applicant as per the following criteria.
- If the client is 25 or older and has no prior speeding ticket, the premium is$500.
- If the client has a speeding ticket and is 25 or older the premium is$1,000.
- Forclientsunder25withoutaspeedingticketthepremiumis$1,500,unlesstheyhavetaken a driving course, in which case the premium is$1,000.
- If the client is under 25 and has a speeding ticket but has also taken a driving course, the premium is$1,500.
- All other clients are refused insurancecoverage.
If eligible then display eligibility and premium amount; otherwise, display appropriate message.
ThefindEligibility()functionmustcheckifalltheparametersareavailableandarenotundefinedbeforeusing them.Thefunctionshoulduseappropriatemechanismsuchasoptionsordefaultvaluesforparameters.
You must use let keyword to declare variables wherever you think is appropriate. Use template literals (` backquote) when displaying output.
Testing:You should use following code snippet to call your function and testyour task 1.
findEligibility("Tom", 26);
findEligibility("Jerry", 30, true);
findEligibility("Mickey", 22, false); findEligibility("Minnie", 20, false, true); findEligibility("Donald", 21, true, true); findEligibility("Louie", 20, true, false);
Sample Output for task 1: -----------------------------------------------------------------------------
Checking eligibility for Tom age = 26
has speeding ticket = No
has taken driving course = No
Is applicant eligibile ? Yes Premium Amount : 500
-----------------------------------------------------------------------------
Checking eligibility for Jerry age = 30
has speeding ticket = Yes
has taken driving course = No
Is applicant eligibile ? Yes Premium Amount : 1000
-----------------------------------------------------------------------------
Checking eligibility for Mickey age = 22
has speeding ticket = No
has taken driving course = No
Is applicant eligibile ? Yes Premium Amount : 1500
-----------------------------------------------------------------------------
Checking eligibility for Minnie age = 20
has speeding ticket = No
has taken driving course = Yes
Is applicant eligibile ? Yes Premium Amount : 1000
-----------------------------------------------------------------------------
Checking eligibility for Donald age = 21
has speeding ticket = Yes
has taken driving course = Yes
Is applicant eligibile ? Yes Premium Amount : 1500
-----------------------------------------------------------------------------
Checking eligibility for Louie age = 20
has speeding ticket = Yes
has taken driving course = No Is applicant eligibile ? No
-----------------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
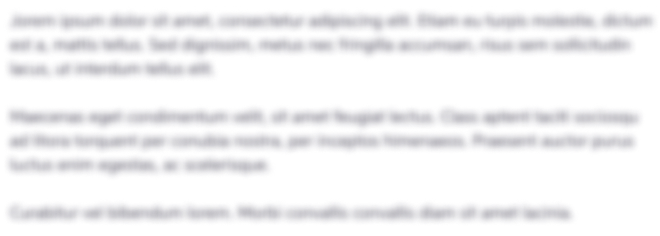
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started