Question
You are provided with a zip file attached below, which contains a solution of a console app, with a single cs file (Program.cs) It has
You are provided with a zip file attached below, which contains a solution of a console app, with a single cs file (Program.cs)
It has two functions, the main function is where you will be writing your code.
The second function is provided to you, this function is called Decrypt, it takes an encrypted string as a parameter, and returns the decrypted string back.
You are also provided with a text file with secret 50 lines of text, each of these lines is an encrypted string that can be decrypted by the function provided in the program.
You are required to decrypt those 50 lines using the function provided to you.
You are required to write program where 10 threads are created and each thread is responsible to decrypt only 5 lines. You are not allowed to use the same thread to decrypt more than 5 lines.
You are not allowed to change anything in the Decrypt function.
Your mission, is to create 10 concurrent threads. Each thread should access the file, choose 5 lines, and use the decrypt function 5 times to decrypt one line at a time, and write back the results to a new file.
If you are successful, the new file would have all text in readable English.
Here is the backbone of the program:
namespace Spies { internal class Program {
static void Main(string[] args) {
//Each thread can only use the Decrypt function 5 times only, it will throw an exception otherwise.
//Distribute the task accross multiple threads, each thread should read 5 lines, decrypt them, and write them back to the original file in their original place.
// Success is when you have a file with 50 lines of readable english text.
// Below is an example of how to use the decrypt function.
Console.WriteLine(Decrypt("YqKdDLNnFJt+MNPkUHVCJSPzzDQcv6f7oiiacLBEnakXDCOminnBdnZS+oMNa4C/goPi8VJ1XRS7DIjMvsuhMvMMKpGZQpIP180zMT8H//9hMbzMvLzY+YtQiARF5Q7RBaoYhRutkcAhSAi3W2pAKQ==", out string error));
}
// Please don't change anything in the below helper function, but please do use it :)
public static string Decrypt(string value, out string exception) {
string password = "password"; string salt = "salt";
bool exist = callers.TryGetValue(System.Threading.Thread.CurrentThread.ManagedThreadId, out int count);
if (!exist) { callers.Add(System.Threading.Thread.CurrentThread.ManagedThreadId, 1); } else
{ if (count == 5) throw new Exception("Cannot be used by same thread more than 5 times!"); else callers[System.Threading.Thread.CurrentThread.ManagedThreadId] = count + 1; }
string plaintext = ""; exception = ""; try { string localpassword = password + DateTime.UtcNow.ToString("ddMMyyyy");
DeriveBytes rgb = new Rfc2898DeriveBytes(localpassword, Encoding.Unicode.GetBytes(salt));
SymmetricAlgorithm algorithm = new AesManaged();
byte[] encrypted = Convert.FromBase64String(value); byte[] rgbKey = rgb.GetBytes(algorithm.KeySize >> 3); byte[] rgbIV = rgb.GetBytes(algorithm.BlockSize >> 3);
string key = Convert.ToBase64String(rgbKey); string IV = Convert.ToBase64String(rgbIV); ICryptoTransform transform = algorithm.CreateDecryptor(rgbKey, rgbIV);
using(MemoryStream buffer = new MemoryStream(encrypted)) { using(CryptoStream stream = new CryptoStream(buffer, transform, CryptoStreamMode.Read)) {
using(StreamReader reader = new StreamReader(stream)) plaintext = reader.ReadToEnd(); }
} } catch (Exception ex) { exception = ex.ToString(); }
return plaintext; }
static Dictionary < int, int > callers = new Dictionary < int, int > (); // Please ignore this, don't remove it :)
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
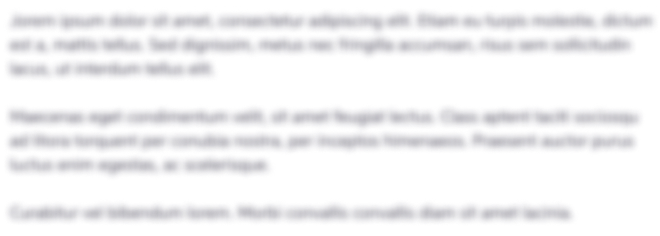
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started