Question
You are required to model the process of purchase orders in a company. Assume that the company makes only one product. The company receives orders
You are required to model the process of purchase orders in a company. Assume that the company makes only one product. The company receives orders from different customers. Every order has two characteristics: customer and units of product. The orders are processed by order (FIFO). Given below is the class Order:
public class Order {
private String customerName;
private int quantity;
public Order(int qtty, String customer) {
this.customerName = customer;
quantity = qtty;
}
public String toString() {
return "\tCustomer: " + customerName + " " + "\tQuantity: " + quantity + " " + "\t------------";
}
public int getQuantity() {
return quantity;
}
public String getCustomerName() {
return customerName;
}
}
Then, implement the Queue class that implements the following interface:
public interface QueueInterface {
// returns the number of elements in Queue
public int size();
// verifies if the Queue is empty. true if empty | false if not empty.
public boolean isEmpty();
// returns the first element in Queue. The element IS NOT REMOVED from Queue. Returns null if empty.
public Object front();
// adds a new element to the Queue.
public void enqueue(Object info);
// returns the first element in Queue. The element IS REMOVED from Queue. Returns null if empty.
public Object dequeue();
}
To do it, implement also the Node class that represents each node in the linked queue. Then, add a new method such as getQueueDetails() to the Queue class. This method should return the size of the queue and should go from first element to last one and present the data contained inside each order, by using the toString() method from Order class. That is, it should present something similar to:
********* QUEUE OF ORDER*********
Size: 4
** Element 1
Customer: cust1
Quantity: 20
------------
** Element 2
Customer: cust2
Quantity: 30
------------
** Element 3
Customer: cust3
Quantity: 40
------------
** Element 4
Customer: cust3
Quantity: 50
------------
******************************
The program has to traverse the queue in order to show its elements. It begins with the first node, then the second node is reached through the next reference in the first one, then the third node, and so on until the end of the list is reached. The code for that should be something like:
Node current = first;
while (current != null) {
// print here the information of the node
(...)
current = current.getNext();
}
Finally, implement a TestQueue class with a main() method to test the code. Invoke all the methods defined in the Queue class.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
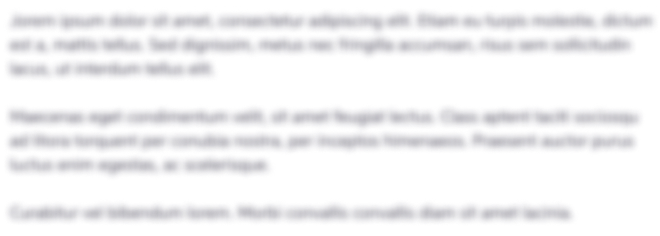
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started