Answered step by step
Verified Expert Solution
Question
1 Approved Answer
You are tasked to write a Java program that filters a list of products using Predicate and negate ( ) methods. The program should receive
You are tasked to write a Java program that filters a list of products using Predicate and negate methods. The program should receive a list of products and two predicates. The first predicate should filter out products that are out of stock, and the second predicate should filter out products that have a price greater than $ Your program should return a list of products that match both predicates.
Instructions:
Create a Product class with the following attributes: name String price double and isOutOfStock boolean
Define a List of Product objects with at least elements.
Define a Predicate that returns true if the given product is out of stock.
Define a Predicate that returns true if the given product's price is greater than $
Use the Predicate and negate methods to filter the list of products. First, filter out products that are out of stock, and then filter out products that have a price greater than $
Print the resulting list of products.
Suggested Skeleton:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.function.Predicate;
class Product
private String name;
private double price;
private boolean isOutOfStock;
Constructor, getters, and setters
@Override
public String toString
return "Product
"name name
price price
isOutOfStock isOutOfStock
;
public class PredicateExample
public static void mainString args
List products new ArrayListArraysasList
new ProductiPhone false
new ProductMacBook Pro", false
new ProductiPad Pro", true
new ProductAirPods false
new ProductApple Watch", true
;
Write your first predicate code here
Write your second predicate code here
Research streamfilter and write the code for filtering here
Write the code to print the filtered list code here
Step by Step Solution
There are 3 Steps involved in it
Step: 1
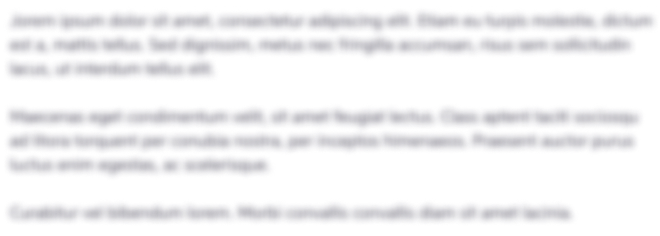
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started