Question
You are tasked with the problem of writing a program to help the doctor at an after hours clinic manage the patients waiting to see
You are tasked with the problem of writing a program to help the doctor at an after hours clinic manage the patients waiting to see him.
During the check-in process, each patient is given a priority ranking from 1-5 by the receptionist regarding how urgent it is for the patient to see the doctor (with 1 being the highest priority).
The patient is also given a unique waiting number. The waiting number is determined like this:
The first person with priority 1 is given waiting number 1000, the second 1001, etc. The first person with priority 2 is given waiting number 2000, the second 2001, etc. The first person with priority 3 is given waiting number 3000, the second 3001, etc. The first person with priority 4 is given waiting number 4000, the second 4001, etc. The first person with priority 5 is given waiting number 5000, the second 5001, etc.
When the doctor becomes free they will consult the priority queue and see the patient with the highest priority first. Patients with the same priority will be seen in a FIFO manner (Hint: the waiting number can help you determine FIFO).
Project Composition
Your solution must include the following components:
Patient class must implement the Comparable interface o Attributes: first name, last name, and waiting number. o Constructor o Get/set methods o compareTo method ensures patients are seen in the correct order o toString method prints the waiting number
PQMinHeap class o Must use Generics x Hint: Add > after class name o Attributes: the array to function as a minheap and number of items in the array o Constructor o Add method x This will add an item to the heap (array) and make any swaps necessary x Note: this can have an additional helper method o Remove method x This will remove an item from the heap (array) and make any swaps necessary x Can also have additional helper method PQTest class o This is the driver class o When turned in, it must contain the following code:
PQMinHeap mp = new PQMinHeap(); mp.add(new Patient("Bob", "Jones", 3000)); mp.add(new Patient("Dave", "Smith", 2000)); mp.add(new Patient("Susie", "Smith", 1000)); mp.add(new Patient("Jessica", "Montgomery", 2001));
System.out.println("Processing patient number: " + mp.remove()); System.out.println("Processing patient number: " + mp.remove());
mp.add(new Patient("Billy", "Joel", 1001)); mp.add(new Patient("Dawn", "Angelo", 3001)); mp.add(new Patient("Sally", "Atwater", 3002)); mp.add(new Patient("Michael", "Jordan", 5000));
System.out.println("Processing patient number: " + mp.remove()); System.out.println("Processing patient number: " + mp.remove()); System.out.println("Processing patient number: " + mp.remove());
mp.add(new Patient("Nikki", "Last", 5001)); mp.add(new Patient("Mike", "Powers", 2002));
for (int i=0; i<5; ++i) { System.out.println("Processing patient number: " + mp.remove()); }
You must use a min-heap to implement the priority queue. You may NOT use Javas built in PriorityQueue class.
Minimum Test When tested with the sample driver provided above, you should get the following output: Processing patient number: 1000 Processing patient number: 2000 Processing patient number: 1001 Processing patient number: 2001 Processing patient number: 3000 Processing patient number: 2002 Processing patient number: 3001 Processing patient number: 3002 Processing patient number: 5000 Processing patient number: 5001
*In Java please
Step by Step Solution
There are 3 Steps involved in it
Step: 1
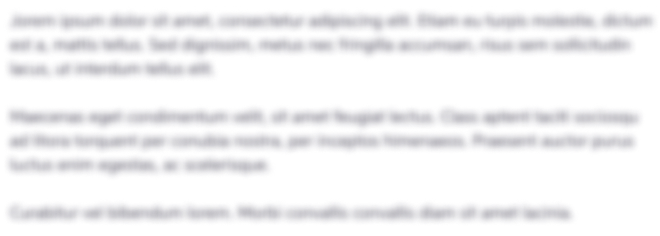
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started