Question
You are to create a new C# project to keep track of truck and car inventories for a car dealership. The user can add vehicles
You are to create a new C# project to keep track of truck and car inventories for a car dealership. The user can add vehicles and view details about each one.
The project will create lists of car and truck objects. The user can add cars and trucks from an interface.
Create a Vehicle class that will contain properties for VIN#, Make, Model, Year, Invoice Price, Mileage, and Date Purchased. The constructor will NOT accept any arguments. This will be the base class. Create two derived classes. One class will contain Truck data and the second will contain Car data. The derived classes will inherit all properties.
The first user interface will contain text boxes for entering the properties for a new vehicle. The program will call a validation method to validate all entries before creating a new vehicle object and adding to the appropriate object list.
To be valid:
VIN#, Make and Model are required entries. Invoice price must be between 0 to 100,000 (inclusive). Mileage must be >= 0. Year (year of vehicle, not year purchased) must be 1900 2018. Date purchased must be a valid date entry today or earlier. You can compare a DateTime field to todays date by accessing the DateTime.Today property. You can validate a date entry by creating a DateTime field and using the DateTime.TryParse method. The user should be able to enter as mm/dd/yyyy.
A truck or car option must be selected from radio buttons.
If all passed validation, add the object to the appropriate list (either car or truck - based on a radio button selection). If any failed, inform the user of the specific problem.
The user will have the option to view detail data about each vehicle in the list. (Use radio button selection on first form). A second form will load all VIN#s in a list box for the user selection (cars or trucks). The user can then select VIN # from the list to view all properties associated with that vehicle.
The form closing event will create two files with all data from each list (Cars.txt and Trucks.txt). The files should be a comma separated file.
The form load event will read the two files and load the two lists with the car and truck data.
Feel free to add any other fun stuff to make it more real-world! (Ex Maybe removing vehicles from the list?... so many things to add!) Just make sure the basics of the program are working first.
Extra credit: 10 points. VIN# must be 10 characters. First two must be numbers, next two must be lower case letters, fifth must be a special character and the rest must be numbers.
Extra credit 5 points. VIN# must be unique.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
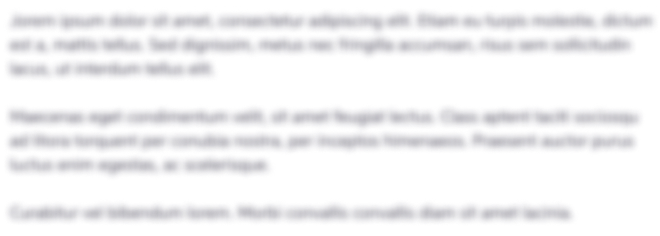
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started