Question
You are to develop a model for robots to save people in a Cartesian plane in case of fire. A robot has a name and
You are to develop a model for robots to save people in a Cartesian plane in case of fire. A robot has a name and (x, y) coordinates, and two robots are considered identical if they have the same name. In order to save people, a robot has to support a method explore. This method takes as parameter a destination (destX, destY) coordinates; and it returns all the possible sequences of moves that will get the robot to that destination starting from its initial coordinates. Suppose that a robot is allowed to repeatedly make one of these three moves:
-move North (abbreviated "N"), which will increase y-coordinate by 1.
-move East (abbreviated "E"), which will increase x-coordinate by 1.
-move Northeast (abbreviated "NE"), which will increase both x-coordinate and y-coordinate by 1.
For example, starting from the origin (0, 0), these three different moves N, E and NE, would leave you in the following locations (0, 1), (1, 0) and (1, 1), respectively.
Write a class Robots that consists of a set of Robot objects. Robots class must support: addRobot method that takes (x, y) coordinates and a name. This method throws a checked exception RobotExistException, if there already exists a robot with the given name; otherwise a robot will be created with the given input and added to the set of robots. Moreover, Robots class must support for-each primitive, where the list of robots is iterated in an increasing alphabetical order with respect to their names.
Note that, performance is a key point in this problem. Your program should run in the most efficient way.
Feel free to use any existing data structure provided by Java.
Provided Robot class as follows:
public class Robot {
private String name;
private double x;
private double y;
public Robot(String name, double x, double y) {
this.name = name;
this.x = x;
this.y = y;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getX() {
return x;
}
public void setX(double x) {
this.x = x;
}
public double getY() {
return y;
}
public void setY(double y) {
this.y = y;
}
}
Below is a sample driver with its corresponding output.
Program Input:
public static void main(String[] args) { Robots robots = new Robots();
try { robots.addRobot(1, 2, "r2"); robots.addRobot(2, 2, "r1"); robots.addRobot(3, 2, "r2");
} catch(RobotExistException e) {
System.out.println("Exception: " + e.getMessage()); }
for(Robot r: robots) { System.out.println("Robot " + r + " starts exploring:"); System.out.println(r.explore(2, 3)); System.out.println("----------------------------------");
} }
Output:
Exception: Robot r2 Already Exists Robot Robot r1 starts exploring: [ N]
----------------------------------
Robot Robot r2 starts exploring:
[NE, EN, NE]
----------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
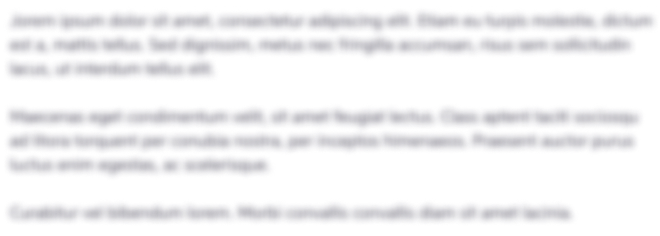
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started