Question
You are to write a maze class. RIGHT CLICK THE IMAGES AND OPEN TO ANIOTHER TAB TO READ THE INSTRUCTIONS. codes: Driver: import java.awt.*; import
You are to write a maze class. RIGHT CLICK THE IMAGES AND OPEN TO ANIOTHER TAB TO READ THE INSTRUCTIONS.
codes:
Driver:
import java.awt.*; import java.awt.event.*; import java.util.*; import java.io.*; import javax.swing.*; //for JOptionPane
public class MazeDisplay extends JFrame implements Runnable, Serializable { //------------ constants private final int START_WIDTH = 700; private final int START_HEIGHT = 500; private final int ANIMATIONDELAY = 0; //Animation display rate (in milliseconds), so 20fps
//------------ data private int cellDim; private int[ ][ ] mazeArray; private int numArrayRows; private int numArrayCols;
private Graphics g; private Thread animationThread; private Insets insets;
//----------- constructor(s) // Parameterized constructor which receives an int that is the landscapeID public MazeDisplay(Maze aMaze) { //if aMaze is null, throw an exception if (aMaze == null) throw new IllegalArgumentException("trying to create a MazeDisplay with a null Maze");
//store the reference to aMaze's mazeArray to be used in the display mazeArray = aMaze.getMazeArray();
//isolate the number of rows and the number of columns in the mazeArray numArrayRows = mazeArray.length; numArrayCols = mazeArray[0].length;
//get the number of "real" rows and cols tht the Maze has int numRealRows = numArrayRows/2; int numRealCols= numArrayCols/2;
//calculate the optimum size of one cell int calcWidth = START_WIDTHumRealCols; int calcHeight = START_HEIGHTumRealRows; cellDim = Math.min(calcWidth, calcHeight);
//but if the cellDim is
//set the JFrame attributes setTitle("CSC205AB Maze"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setSize(numRealCols*cellDim+7,numRealRows*cellDim+1+28); //add one for last border, subtract 38 for title bar, borders center(); setResizable(false); setVisible(true);
// use those values to determine the greeny and the orangey insets = getInsets();
// add a WindowListener addWindowListener(new WindowAdapter() {public void windowClosing(WindowEvent e) { System.exit(0);}});
// get the Graphics object that will be used to write to this JFrame; g = getGraphics();
// Anonymous inner class window listener to terminate the program. this.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent e) { System.exit(0); } } );
// Create and start animation thread animationThread = new Thread(this); animationThread.start();
}
//----------- methods(s)
// run will actually run this Frame public void run() { //Loop, sleep, and update sprite positions once each ANIMATIONDELAY milliseconds long time = System.currentTimeMillis(); while (true) //infinite loop { paint(g); try { time += ANIMATIONDELAY; Thread.sleep(Math.max(0,time - System.currentTimeMillis())); } catch (InterruptedException e) { System.out.println(e); }//end catch }//end while loop }//end run method
// center - will set the x and y of this Frame to the center of the screen private void center() { Dimension ScreenSize = Toolkit.getDefaultToolkit().getScreenSize(); Dimension FrameSize = this.getSize(); int x = (ScreenSize.width - FrameSize.width)/2; int y = (ScreenSize.height - FrameSize.height)/2; this.setLocation(x, y); }
//------------------------------------------------ public void windowGainedFocus(WindowEvent e) { repaint(); }
//------------------------------------------------ public void windowLostFocus(WindowEvent e) { repaint(); }
//------------------------------------------------ public void componentResized(ComponentEvent e) { repaint(); }
//------------------------------------------------ public void componentMoved(ComponentEvent e) { repaint(); }
//------------------------------------------------ public void componentShown(ComponentEvent e) { repaint(); }
//------------------------------------------------ public void componentHidden(ComponentEvent e) { //repaint(); }
//------------------------------------------------ public void update(Graphics g) { repaint(); }
//------------------------------------------------ public void actionPerformed(ActionEvent e) { }
// paint - repaints the whole Maze. Uses "double-buffering" to eliminate flickering. public void paint(Graphics g) { // create an image - we will "double buffer" (draw to that image first and then // draw the image) to eliminate flickering Image image = createImage(getWidth(), getHeight()); Graphics graphicsBuffer = image.getGraphics();
// fill the image with the background color //graphicsBuffer.setColor(Color.WHITE); //graphicsBuffer.fillRect(12, 12, getWidth()-24, getHeight()-24);
graphicsBuffer.setColor(Color.BLACK);
for (int row=0; row for (int col=0; col { if (row%2==1 && col%2==1 && mazeArray[row][col] == 3) //odd rows, odd cols are the actual cells { graphicsBuffer.setColor(Color.MAGENTA); //goal int startx = insets.left + col/2 * cellDim; int starty = insets.top + row/2 * cellDim; graphicsBuffer.fillRect(startx, starty, cellDim, cellDim); } if (row%2==1 && col%2==1 && mazeArray[row][col] == 2) //odd rows, odd cols are the actual cells { graphicsBuffer.setColor(Color.YELLOW); //current int startx = insets.left + col/2 * cellDim; int starty = insets.top + row/2 * cellDim; graphicsBuffer.fillRect(startx, starty, cellDim, cellDim); } else if (row%2==1 && col%2==1 && mazeArray[row][col] == 0) //odd rows, odd cols are the actual cells { graphicsBuffer.setColor(Color.WHITE); //current int startx = insets.left + col/2 * cellDim; int starty = insets.top + row/2 * cellDim; graphicsBuffer.fillRect(startx, starty, cellDim, cellDim); } }
graphicsBuffer.setColor(Color.BLACK);
for (int row=0; row for (int col=0; col { if (row%2==0 && col%2==1 && mazeArray[row][col] == 1) //even rows, odd cols are the horizontal walls { int startx = insets.left + col/2 * cellDim; int endx = insets.left + col/2 * cellDim + cellDim; int starty = insets.top + row/2 * cellDim; int endy = insets.top + row/2 * cellDim; graphicsBuffer.drawLine(startx, starty, endx, endy); }
else if (row%2==1 && col%2==0 && mazeArray[row][col] == 1) //odd rows, even cols are the vertical walls { int startx = insets.left + col/2 * cellDim; int endx = insets.left + col/2 * cellDim; int starty = insets.top + row/2 * cellDim; int endy = insets.top + row/2 * cellDim + cellDim; graphicsBuffer.drawLine(startx, starty, endx, endy); } }
// copy the image to the actual Frame g.drawImage(image, 0, 0, Color.WHITE, null);
repaint();
}
}
Maze:
import java.awt.*;
import java.io.*;
import java.util.*;
public class Maze
{
//-------data
private int[ ][ ] mazeArray;
private int currentArrayRow;
private int currentArrayCol;
private int goalArrayRow;
private int goalArrayCol;
private int buildAnimationDelay;
private int solveAnimationDelay;
private boolean alreadyBuilt;
//-------constructors
public Maze(int numRealRows, int numRealCols)
{
//since the maze is being created, initialize alreadyBuilt to false
alreadyBuilt = false;
//set the buildAnimationDelay and solveAnimationDelay to 0; they can be reset with methods
buildAnimationDelay = 0;
solveAnimationDelay = 0;
//make sure that the numRealRows and numRealCols are both > 1 (start cannot == goal)
if (numRealRows
throw new IllegalArgumentException("number of rows must be > 1");
if (numRealCols
throw new IllegalArgumentException("number of columns must be > 1");
//create the 2D array to hold the maze (its even rows/cols hold the walls, odd rows/cols hold the paint
mazeArray = new int[2*numRealRows+1][2*numRealCols+1];
//since the even values are the walls, set anything with an even component to 1 (wall exists to start)
for (int row=0; row
for (int col=0; col
if (row%2==0 || col%2==0) //if either dimension is even...
mazeArray[row][col] = 1; //its a wall, so set value to 1
//initialize the currentArrayRow and currentArrayCol to the upper left corner
currentArrayRow = 1;
currentArrayCol= 1;
}
// **************** methods *******************************************
//------- setSolveAnimationDelay - sets the delay (milliseconds) for the maze being solved (in case its animated)
public void setSolveAnimationDelay(int theDelay)
{
solveAnimationDelay = theDelay;
}
//------- buildMaze - builds the Maze; calls other buildMaze method to set buildAnimationDelay to 0
public void buildMaze()
{
buildMaze(0);
}
//------- buildMaze - builds the Maze; receives a delay to slow it down (in case its displayed)
public void buildMaze(int buildAnimationDelay)
{
//if this maze has already been built and it trying to be built again, throw an exception
if (alreadyBuilt)
throw new IllegalStateException("cannot build maze - it has already been built");
else
alreadyBuilt = true; //because we are NOW building it
System.out.println(" beginning to build the maze with " + mazeArray.length/2 + " rows, " + mazeArray[0].length/2 + " cols");
//create a Stack to hold the cells we are visiting as it is built (they will be stored as Points)
//and an ArrayList to hold the "neighbors" in the code below
Stack cellStack = new Stack();
ArrayList neighborAL;
//in the mazeArray, the even rows/cols are the walls, the odd rows/cols are the cells
int numRealRows = mazeArray.length/2;
int numRealCols = mazeArray[0].length/2;
//calculate the total number of (real) cells to visit
int totalCells = numRealRows * numRealCols; //rows x cols
//the odd rows/cols store the actual cells. Choose a random cell to start.
Random gen = new Random();
int currentArrayRow = gen.nextInt(numRealRows)*2 + 1; //ex: if 3 real rows, this is a random from 1,3,5
currentArrayCol = gen.nextInt(numRealCols)*2 + 1; //same for cols...
int lastArrayRow = currentArrayRow;
int lastArrayCol = currentArrayCol;
mazeArray[currentArrayRow][currentArrayCol] = 2;
int numVisitedCells = 1;
//while all cells have not been visited...
while(numVisitedCells
{
//go to sleep to slow down animation (based on its speed)
try{ Thread.sleep(buildAnimationDelay); }
catch(Exception ex) {}
//find all neighbors of currentCell with all walls intact
neighborAL = new ArrayList();
//try cell above it
if (inMaze(currentArrayRow-2, currentArrayCol) && allWallsIntact(currentArrayRow-2, currentArrayCol))
neighborAL.add(new Point(currentArrayRow-2, currentArrayCol));
//try cell below it
if (inMaze(currentArrayRow+2, currentArrayCol) && allWallsIntact(currentArrayRow+2, currentArrayCol))
neighborAL.add(new Point(currentArrayRow+2, currentArrayCol));
//try cell to the left of it
if (inMaze(currentArrayRow, currentArrayCol-2) && allWallsIntact(currentArrayRow, currentArrayCol-2))
neighborAL.add(new Point(currentArrayRow, currentArrayCol-2));
//try cell to the right of it
if (inMaze(currentArrayRow, currentArrayCol+2) && allWallsIntact(currentArrayRow, currentArrayCol+2))
neighborAL.add(new Point(currentArrayRow, currentArrayCol+2));
//if neighbors with intact walls exist...
if (neighborAL.size() > 0)
{
//choose a neighbor at random
int randomInt = gen.nextInt(neighborAL.size());
Point theNeighbor = neighborAL.get(randomInt);
int neighborRow = (int)theNeighbor.getX();
int neighborCol = (int)theNeighbor.getY();
//knock down the wall in between
if (currentArrayRow != neighborRow) /eighbor chosen was above or below
mazeArray[(currentArrayRow+neighborRow)/2][currentArrayCol] = 0; //knock down wall in between
else if (currentArrayCol != neighborCol) /eighbor chosen was to the left or right
mazeArray[currentArrayRow][(currentArrayCol+neighborCol)/2] = 0; //knock down wall in between
//push the current cell onto the cellStack
cellStack.push(new Point(currentArrayRow, currentArrayCol));
//clear the current cell
mazeArray[currentArrayRow][currentArrayCol] = 0;
//make the new cell the current cell
currentArrayRow = neighborRow;
currentArrayCol = neighborCol;
mazeArray[currentArrayRow][currentArrayCol] = 2;
//add 1 to visitedCells
numVisitedCells++;
}
else
{
//clear the current cell
mazeArray[currentArrayRow][currentArrayCol] = 0;
//pop the most recent entry off of cellStack and make it the current cell
Point popped = cellStack.pop();
currentArrayRow = (int)popped.getX();
currentArrayCol = (int)popped.getY();
mazeArray[currentArrayRow][currentArrayCol] = 2;
}
} //end while
//clear the cell that ended up as the current Cell
mazeArray[currentArrayRow][currentArrayCol] = 0;
//set the current cell to the upper left corner
currentArrayRow = 1;
currentArrayCol = 1;
mazeArray[currentArrayRow][currentArrayCol] = 2; //current
//set the goal to the lower right corner
goalArrayRow = numRealRows*2-1;
goalArrayCol = numRealCols*2-1;
mazeArray[goalArrayRow][goalArrayCol] = 3; //goal
System.out.println("finished building the maze ");
}
//-------- getNumRows - returns the number of rows in the maze (from user's perspective)
private int getNumRows()
{
return mazeArray.length/2; // divide by 2 for real answer
}
//-------- getNumCols - returns the number of columns in the maze (from user's perspective)
private int getNumCols()
{
return mazeArray[0].length/2; // number of columns in row0 (divide by 2 for real answer)
}
//-------- allWallsIntact - returns true if the cell at [aRow][aCol] has all walls around it intact
private boolean allWallsIntact(int aRow, int aCol)
{
return (mazeArray[aRow-1][aCol] == 1 && //wall above it exists
mazeArray[aRow+1][aCol] == 1 && //wall below it exists
mazeArray[aRow][aCol-1] == 1 && //wall to the left exists
mazeArray[aRow][aCol+1] == 1); //wall to the right exists
}
//-------- inMaze - returns true if the cell at [aRow][aCol] is in the maze
private boolean inMaze(int aRow, int aCol)
{
return (aRow > 0 && aRow
aCol > 0 && aCol
}
//-------- getCurrentRow - returns the current (real) row
public int getCurrentRow()
{
return currentArrayRow/2;
}
//-------- getCurrentCol - returns the current (real) col
public int getCurrentCol()
{
return currentArrayCol/2;
}
//-------- isOpen - returns true if there is no wall in the direction that is passed in
public boolean isOpen(Direction direction)
{
boolean result = false;
if (direction == Direction.UP && mazeArray[currentArrayRow-1][currentArrayCol]==0)
result = true;
else if (direction == Direction.DOWN && mazeArray[currentArrayRow+1][currentArrayCol]==0)
result = true;
else if (direction == Direction.LEFT && mazeArray[currentArrayRow][currentArrayCol-1]==0)
result = true;
else if (direction == Direction.RIGHT && mazeArray[currentArrayRow][currentArrayCol+1]==0)
result = true;
return result;
}
//-------- isOpenTo - returns true if the current cell is openTo (no wall) the one passed in
private boolean isOpenTo(int aRow, int aCol)
{
boolean result;
if (!isAdjacentTo(aRow, aCol))
result = false;
else if (currentArrayRow-aRow == 2) //IS adjacent, figure which direction and call other method
result = mazeArray[currentArrayRow-1][currentArrayCol]==0; //UP
else if (currentArrayRow-aRow == -2)
result = mazeArray[currentArrayRow+1][currentArrayCol]==0; //DOWN
else if (currentArrayCol-aCol == 2)
result = mazeArray[currentArrayRow][currentArrayCol-1]==0; //LEFT
else if (currentArrayCol-aCol == -2)
result = mazeArray[currentArrayRow][currentArrayCol+1]==0; //RIGHT
else
result = false;
return result;
}
//-------- adjacentTo - returns true if the current cell is adjacentTo (above/below/left/right) current cell
public boolean isAdjacentTo(int aRow, int aCol)
{
//calculate how far the move is (hopefully row OR col is just +-2)
int arrayRowChange = currentArrayRow - aRow;
int arrayColChange = currentArrayCol - aCol;
//it is adjacent if EITHER the rows or the cols differ by 2
return Math.abs(arrayRowChange)==2 ^ Math.abs(arrayColChange)==2; //checking row xor col
}
// -------- move - receives a Direction and moves there if OK. Calls the other
// arrayMove to do this
public boolean move(Direction direction)
{
boolean success = false;
if (direction == Direction.UP)
success = arrayMove(currentArrayRow-2, currentArrayCol);
else if (direction == Direction.DOWN)
success = arrayMove(currentArrayRow+2, currentArrayCol);
else if (direction == Direction.LEFT)
success = arrayMove(currentArrayRow, currentArrayCol-2);
else if (direction == Direction.RIGHT)
success = arrayMove(currentArrayRow, currentArrayCol+2);
return success;
}
//-------- move - receives the literal (not array) row/col to move to. Calls the
// other ArrayMove to do this.
private boolean move(int realRow, int realCol)
{
return arrayMove(2*realRow+1, 2*realCol+1);
}
//-------- arrayMove - moves using the maze array (moves a distance of 2 to get to next cell)
// first checks to see if move is legal. Returns true if successful.
private boolean arrayMove(int newArrayRow, int newArrayCol)
{
boolean success;
//go to sleep to slow down animation (based on its speed)
try{ Thread.sleep(solveAnimationDelay); }
catch(Exception ex) {}
//make sure the new row/col is still in the maze
if (!inMaze(newArrayRow, newArrayCol))
throw new IllegalMazeMoveException("trying to move to cell
newArrayCol/2 + "> which is outside the maze");
//make sure the new row/col is adjacent
else if (!isAdjacentTo(newArrayRow, newArrayCol))
throw new IllegalMazeMoveException("trying to move from cell
"> to non-adjacent cell ");
//make sure there is not a wall in between
else if (!isOpenTo(newArrayRow, newArrayCol))
throw new IllegalMazeMoveException("trying to move from cell
"> to cell and there is a wall in between");
//if OK, move the current cell
else
{
//if new ArrayRow is already in the path, then we are retreating from current location so
//clear current location
if (mazeArray[newArrayRow][newArrayCol] == 2)
mazeArray[currentArrayRow][currentArrayCol] = 0;
currentArrayRow = newArrayRow;
currentArrayCol = newArrayCol; //move current cell
mazeArray[currentArrayRow][currentArrayCol] = 2; //and show it as part of path
success = true;
}
//return
return success;
}
//-------- goalReached - returns true if the maze is solved (current location == goal)
public boolean goalReached()
{
return (currentArrayRow == goalArrayRow && currentArrayCol == goalArrayCol);
}
//-------- getMazeArray - returns the mazeArray
public int[][] getMazeArray()
{
return mazeArray;
}
//***********************************************************************
//This is Maze's enumerated data type: moves can be UP, DOWN, LEFT, RIGHT
public enum Direction implements Serializable
{
UP, DOWN, LEFT, RIGHT
}
//***********************************************************************
//MazeMoveException will be thrown when an illegal move is requested in the maze
public class IllegalMazeMoveException extends IllegalArgumentException implements Serializable
{
/o data is needed - all inherited...
//constructor - just do what the parent class would do if it received the String
public IllegalMazeMoveException(String str)
{
super(str);
}
/o methods are needed - all inherited...
}
}
Maze display:
import java.awt.*; import java.awt.event.*; import java.util.*; import java.io.*; import javax.swing.*; //for JOptionPane
public class MazeDisplay extends JFrame implements Runnable, Serializable { //------------ constants private final int START_WIDTH = 700; private final int START_HEIGHT = 500; private final int ANIMATIONDELAY = 0; //Animation display rate (in milliseconds), so 20fps
//------------ data private int cellDim; private int[ ][ ] mazeArray; private int numArrayRows; private int numArrayCols;
private Graphics g; private Thread animationThread; private Insets insets;
//----------- constructor(s) // Parameterized constructor which receives an int that is the landscapeID public MazeDisplay(Maze aMaze) { //if aMaze is null, throw an exception if (aMaze == null) throw new IllegalArgumentException("trying to create a MazeDisplay with a null Maze");
//store the reference to aMaze's mazeArray to be used in the display mazeArray = aMaze.getMazeArray();
//isolate the number of rows and the number of columns in the mazeArray numArrayRows = mazeArray.length; numArrayCols = mazeArray[0].length;
//get the number of "real" rows and cols tht the Maze has int numRealRows = numArrayRows/2; int numRealCols= numArrayCols/2;
//calculate the optimum size of one cell int calcWidth = START_WIDTHumRealCols; int calcHeight = START_HEIGHTumRealRows; cellDim = Math.min(calcWidth, calcHeight);
//but if the cellDim is
//set the JFrame attributes setTitle("CSC205AB Maze"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setSize(numRealCols*cellDim+7,numRealRows*cellDim+1+28); //add one for last border, subtract 38 for title bar, borders center(); setResizable(false); setVisible(true);
// use those values to determine the greeny and the orangey insets = getInsets();
// add a WindowListener addWindowListener(new WindowAdapter() {public void windowClosing(WindowEvent e) { System.exit(0);}});
// get the Graphics object that will be used to write to this JFrame; g = getGraphics();
// Anonymous inner class window listener to terminate the program. this.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent e) { System.exit(0); } } );
// Create and start animation thread animationThread = new Thread(this); animationThread.start();
}
//----------- methods(s)
// run will actually run this Frame public void run() { //Loop, sleep, and update sprite positions once each ANIMATIONDELAY milliseconds long time = System.currentTimeMillis(); while (true) //infinite loop { paint(g); try { time += ANIMATIONDELAY; Thread.sleep(Math.max(0,time - System.currentTimeMillis())); } catch (InterruptedException e) { System.out.println(e); }//end catch }//end while loop }//end run method
// center - will set the x and y of this Frame to the center of the screen private void center() { Dimension ScreenSize = Toolkit.getDefaultToolkit().getScreenSize(); Dimension FrameSize = this.getSize(); int x = (ScreenSize.width - FrameSize.width)/2; int y = (ScreenSize.height - FrameSize.height)/2; this.setLocation(x, y); }
//------------------------------------------------ public void windowGainedFocus(WindowEvent e) { repaint(); }
//------------------------------------------------ public void windowLostFocus(WindowEvent e) { repaint(); }
//------------------------------------------------ public void componentResized(ComponentEvent e) { repaint(); }
//------------------------------------------------ public void componentMoved(ComponentEvent e) { repaint(); }
//------------------------------------------------ public void componentShown(ComponentEvent e) { repaint(); }
//------------------------------------------------ public void componentHidden(ComponentEvent e) { //repaint(); }
//------------------------------------------------ public void update(Graphics g) { repaint(); }
//------------------------------------------------ public void actionPerformed(ActionEvent e) { }
// paint - repaints the whole Maze. Uses "double-buffering" to eliminate flickering. public void paint(Graphics g) { // create an image - we will "double buffer" (draw to that image first and then // draw the image) to eliminate flickering Image image = createImage(getWidth(), getHeight()); Graphics graphicsBuffer = image.getGraphics();
// fill the image with the background color //graphicsBuffer.setColor(Color.WHITE); //graphicsBuffer.fillRect(12, 12, getWidth()-24, getHeight()-24);
graphicsBuffer.setColor(Color.BLACK);
for (int row=0; row for (int col=0; col { if (row%2==1 && col%2==1 && mazeArray[row][col] == 3) //odd rows, odd cols are the actual cells { graphicsBuffer.setColor(Color.MAGENTA); //goal int startx = insets.left + col/2 * cellDim; int starty = insets.top + row/2 * cellDim; graphicsBuffer.fillRect(startx, starty, cellDim, cellDim); } if (row%2==1 && col%2==1 && mazeArray[row][col] == 2) //odd rows, odd cols are the actual cells { graphicsBuffer.setColor(Color.YELLOW); //current int startx = insets.left + col/2 * cellDim; int starty = insets.top + row/2 * cellDim; graphicsBuffer.fillRect(startx, starty, cellDim, cellDim); } else if (row%2==1 && col%2==1 && mazeArray[row][col] == 0) //odd rows, odd cols are the actual cells { graphicsBuffer.setColor(Color.WHITE); //current int startx = insets.left + col/2 * cellDim; int starty = insets.top + row/2 * cellDim; graphicsBuffer.fillRect(startx, starty, cellDim, cellDim); } }
graphicsBuffer.setColor(Color.BLACK);
for (int row=0; row for (int col=0; col { if (row%2==0 && col%2==1 && mazeArray[row][col] == 1) //even rows, odd cols are the horizontal walls { int startx = insets.left + col/2 * cellDim; int endx = insets.left + col/2 * cellDim + cellDim; int starty = insets.top + row/2 * cellDim; int endy = insets.top + row/2 * cellDim; graphicsBuffer.drawLine(startx, starty, endx, endy); }
else if (row%2==1 && col%2==0 && mazeArray[row][col] == 1) //odd rows, even cols are the vertical walls { int startx = insets.left + col/2 * cellDim; int endx = insets.left + col/2 * cellDim; int starty = insets.top + row/2 * cellDim; int endy = insets.top + row/2 * cellDim + cellDim; graphicsBuffer.drawLine(startx, starty, endx, endy); } }
// copy the image to the actual Frame g.drawImage(image, 0, 0, Color.WHITE, null);
repaint();
}
}
D You are to write the fol x Ask me anything Program 3-solving a Maze In this program, you will use the Stack class that you wrote in MinilabStack. If you do not have it, you can use the built-in class java.util Stack instead. Then you will wile: a class called MazeSolver, which will be able to create a Maze, set up a MazeDisplay, and then solve it. You are given 3 other classes to work with and possibly change slightly when it is time to implement Scrialization and File input. Program3Driver-which starts the program Maze which is a maze Maze Display which graphically displays the maze it is given. After everything is working, you will add a loop and Serialization in so you can cither quit at any time or save your Mazesolver at any time and read it back in later. Please read all parts carefully: Part (just background, but very important so you will know how to call the Maze's methods Look at the Maze java file, it contains the code for the Maze. You do not have to know or understand all the code for the Maze class, but it uses a feature called enumerated data types that you will use extensively. At the very end of the Maze class is defined an enum called Direction. When working with a Maze, the directions that you can use are UP, DOWN, LEFT, and RIGHT. Since Direction is defined inside Maze, you have to refer to it as Maze.Direction and refer to the various directions as Maze.Direction.UPetc. You can use enumerated data types as a type in the same way that you would use other types or classes. For example, you could have o Maze. Direction whichWay, //which Way is a Maze. Direction, can have a value of //Maze.Maze Direction, LEFT for example. o Stack Maze Direction myStack //Generics o whichWay Maze. Direction.UP /ote This will be extremely important, as the Maze methods that you have available are expecting Maze, Directions... For background only (not needed to know or use is a class that is defined at the very end of Maze java. It of exception calle nting it out because cgalMazeMowcEx s a custom ty tion ctivate Windows o Settings to activate Windows. 2.46 PM 3/26/2017 D You are to write the fol x Ask me anything Program 3-solving a Maze In this program, you will use the Stack class that you wrote in MinilabStack. If you do not have it, you can use the built-in class java.util Stack instead. Then you will wile: a class called MazeSolver, which will be able to create a Maze, set up a MazeDisplay, and then solve it. You are given 3 other classes to work with and possibly change slightly when it is time to implement Scrialization and File input. Program3Driver-which starts the program Maze which is a maze Maze Display which graphically displays the maze it is given. After everything is working, you will add a loop and Serialization in so you can cither quit at any time or save your Mazesolver at any time and read it back in later. Please read all parts carefully: Part (just background, but very important so you will know how to call the Maze's methods Look at the Maze java file, it contains the code for the Maze. You do not have to know or understand all the code for the Maze class, but it uses a feature called enumerated data types that you will use extensively. At the very end of the Maze class is defined an enum called Direction. When working with a Maze, the directions that you can use are UP, DOWN, LEFT, and RIGHT. Since Direction is defined inside Maze, you have to refer to it as Maze.Direction and refer to the various directions as Maze.Direction.UPetc. You can use enumerated data types as a type in the same way that you would use other types or classes. For example, you could have o Maze. Direction whichWay, //which Way is a Maze. Direction, can have a value of //Maze.Maze Direction, LEFT for example. o Stack Maze Direction myStack //Generics o whichWay Maze. Direction.UP /ote This will be extremely important, as the Maze methods that you have available are expecting Maze, Directions... For background only (not needed to know or use is a class that is defined at the very end of Maze java. It of exception calle nting it out because cgalMazeMowcEx s a custom ty tion ctivate Windows o Settings to activate Windows. 2.46 PM 3/26/2017Step by Step Solution
There are 3 Steps involved in it
Step: 1
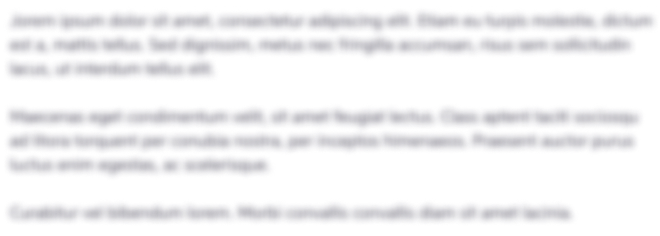
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started