Question
You are to write a MIPS program in MARS that adds, subtracts, and multiply arrays. Given arrays named arr1,arr2, arr3, arr4, arr5, arr6, arr7, and
You are to write a MIPS program in MARS that adds, subtracts, and multiply arrays. Given arrays named arr1,arr2, arr3, arr4, arr5, arr6, arr7, and arr8: arr3 = arr1 + arr2 arr4 = arr1 - arr2 arr5 = arr1 + 4 arr6 = arr2 - 8 arr7 = values enterd by user arr8 = arr1 * arr2
These are element by element operations so arr3 = arr1 + arr2 means arr3[0] = arr1[0] + arr2[0] arr3[1] = arr1[1] + arr2[1] arr3[2] = arr1[2] + arr2[2] Do not use a loop but perform each task using straight line code.
For arr5 = arr1 + 4 do not load the constants into a register, instead use an immediate operation for each sum. Simialrly for the arr6 = arr2 - 8 task.
When you display arr1 to the I/O screen make sure you also output a message describing the output.
The multiplication will require you to use the mul and the mflo instructions
My code so far:
.data
arr1: .word 15,25,35 # arr1 and arr2 are arrays
.space 4
arr2: .word 10,20,30 # of given numbers
.space 4
arr3: .word 3,3,3 # array to hold arr1 + arr2
.space 4
arr4: .word 4,4,4 # array to hold arr1 - arr2
.space 4
arr5: .word 5,5,5 # array to hold arr1 + 4
.space 4
arr6: .word 6,6,6 # array to hold arr2 - 8
.space 4
arr7: .word 7,7,7 # array to hold input values
.space 4
arr8: .word 8,8,8 # array to hold arr1 * arr2
.space 36
str1: .asciiz
.text
# add arr1 to arr2 placing the result in arr3
la $t0, arr3 la $t1, arr1 la $t2, arr2 add $t0, $t1, $t2 syscall
# subtract arr2 from arr1 place result in arr4
# add 4 to each item in arr1 place result in arr5
# subtract 8 from each item in arr2
# place result in arr6
# Prompt user for values, place user values in arr7
# multiply arr1 and arr2 place result in arr8
# Display with appropriate messaging, the values
# for arr1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
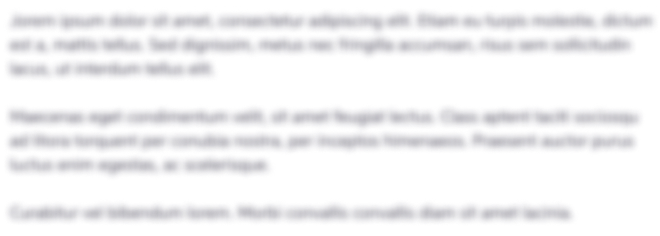
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started