Answered step by step
Verified Expert Solution
Question
1 Approved Answer
You are to write a program that allows a user purchase tickets to a Broadway musical. The program asks the user how much money they
You are to write a program that allows a user purchase tickets to a Broadway musical. The
program asks the user how much money they have to spend on tickets and then asks them how
many tickets they would like to purchase. The program must implement the following function
that is called once the user has entered all the information:
Function: PurchaseTickets
Parameters:
pRemainingCash points to a variable containing the
amount of cash the user has
adultTickets specifies the number of adult tickets
the user wants to purchase.
childTickets specifies the number of child tickets
the user wants to purchase.
Description:
The function will determine if the user has enough
money to purchase the specified number of tickets.
If they do then the function deducts the proper
funds from their remaining cash and returns
the total number of tickets purchased. If they do not
the function returns
int PurchaseTicketsdouble pRemainingCash int adultTickets,
int childTickets;
You must also use the following two constants to represent the ticket prices:
#define ADULTTICKETPRICE
#define CHILDTICKETPRICE
The program must prompt the user for the following information and in the following order:
Amount of cash they have
Number of child tickets to purchase
Number of adult tickets to purchase
If the user has enough money to purchase the requested tickets, display the number of tickets
they purchased and the remaining cash they have after the purchase. If the user does not have
enough money to purchase the tickets, tell them and exit the program.
Here are two sample interactions:
Sample :
How much money do you have to purchase tickets?
How many child tickets would you like to purchase?
How many adult tickets would you like to purchase?
You have purchased tickets, and you have $ remaining
Sample :
How much money do you have to purchase tickets?
How many child tickets would you like to purchase?
How many adult tickets would you like to purchase?
You do not have enough money to purchase the tickets
Here is what I have so far:
#include
#define ADULTTICKETPRICE
#define CHILDTICKETPRICE
int PurchaseTicketsdouble pRemainingCash int adultTickets, int childTickets;
int mainvoid
double cash;
int childTickets;
int adultTickets;
printf to ask for the amount of cash
printfHow much money do you have to purchase tickets?:
;
scanf and error checking
scanfd cash;
ask for number of child tickets
printfHow many child tickets would you like to purchase?:
;
scanf and error checking
scanfd childTickets;
ask for number of adult tickets
printfHow many adult tickets would you like to purchase?:
;
scanf and error checking
scanfd adultTickets;
if PurchaseTickets&cash, adultTickets, childTickets
printfYou have purchased d tickets, and you have $f remaining.", adultTickets childTickets, cash;
else
printfYou did not purchase any tickets.
;
return ;
int PurchaseTicketsdouble pRemainingCash int adultTickets, int childTickets
int numTicketsPurchased ;
return numTicketsPurchased; if user does not have enough money for the tickets
Step by Step Solution
There are 3 Steps involved in it
Step: 1
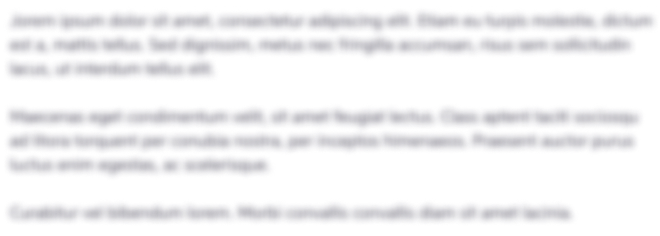
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started