Answered step by step
Verified Expert Solution
Question
1 Approved Answer
You are to write simple MSP 4 3 0 assembly functions for a program that will take a decimal number and a base as input
You are to write simple MSP assembly functions for a program that will take a decimal number and a base as input and output the value in that specified base. For example:
E
LQ
SZ
Sa
T
xM
This is just example output, you should test against as many possible inputs as you can.
Your program should be able to handle all bases from to and
It must have completed assembly functions:
unsigned convertunsigned decimal, unsigned base
unsigned otherbaseunsigned decimal, unsigned base
unsigned powerbaseunsigned decimal, unsigned base
unsigned baseunsigned decimal, unsigned base
unsigned logunsigned value
unsigned rshiftunsigned value, unsigned amount
The call graph of a possible solution program looks like:
convert
powerbase
log
rshift
otherbase
umod
udiv
base
log
otherbase
Submit only the modifications to the files in the func folder
When unexpected input is given, a should be output to minicom see above
Don't worry about overflow
Converting Integers to Characters
Think about how to use this character array:
char ascii ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz;
The character is not the same as the value If you need to know which numeric values correspond to which on screen characters, look at man ascii in your terminal.
Division & Modulo
This algorithm is suitable for any base that is not a power of two and is not base otherbase function
Let's see an example of converting the decimal number into base How many different values are there for base : and So you should expect all of our digits to be one of those numbers. Get the first digit by calculating the remainder of :
The result of is with a remainder of
There is a special operator for this in almost all programming languages: modulo operator. This will give the remainder instead of the quotient.
This is the first digit ones digit of the base number. To continue, you start from the quotient of this operation. Division in C is integer division just like in Python using so the remainder is discarded when you do:
Now, continue with the algorithm:
The result of is with a remainder of
This is the second digit fives digit of the base number.
The algorithm continue until this division results in :
Now we have a remainder of and the result of the integer division is
The number in base is
Mask & Shift
This algorithm is suitable for any base that is a power of two and is not base powerbase function
What if you are trying to convert the number into base How many bit patterns are there for base
which is and Let's see how masking works:
&
You've "masked off" the first digit, which is by using the & bitwise AND operator. Now you can shift those bits to the right using the bitwise RIGHT SHIFT operator like so:
And continue with the algorithm:
&
The number in base is
Base
This algorithm will only be used for base base function
It will involve using both previous algorithms. Since this is a power of mask & shift must be utilized. However, the values you get will be numbers between and The ascii character array doesn't have that many different characters in it so instead you will represent base numbers in the same format as IP addresses:
Each place value is separated by a character. The division & modulo algorithm will be used to calculate each digit of the decimal number for each place value.
In the above example, the ones place shows the number which would have been calculated using mask & shift from the input number. Then that value needs to be placed in the buffer as a three digit decimal number by using the division & modulo algorithm.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
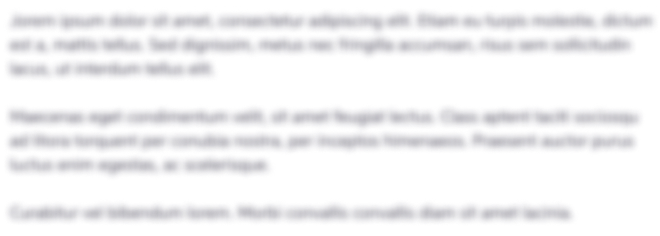
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started