Question
: You may find that this assignment is easier to complete if you think about how you can use variables and fields in your solution.
:
You may find that this assignment is easier to complete if you think about how you can use variables and fields in your solution. Recall that a variable is a name that holds a data value or an object--so think about what values your jeroo will need to remember in order to complete its task. A field is a value stored in the object itself, rather than used locally inside a single method--a field is accessible in all of your methods, and some methods may behave differently depending on the value of the field(s).
Note: this lab does not have a starting scenario to download.
Part 1: Creating Classes
Your goal in this lab is to (by the end) produce a jeroo subclass that begins with a fixed number of flowers in its pouch (enough to plant a perfect square), and is placed somewhere on the island. Suppose the jeroo is going to create a K+1 by K+1 square by planting K flowers on each side (since each corner flower is counted on two sides, only K flowers are needed for each side). To accomplish this task, it will begin with 4 * K flowers in its pouch. The jeroo will plant flowers in a square by beginning at its starting location (whatever direction it is facing), and planting forward, turning right, planting another side, and proceeding in a clockwise planting direction until it arrives back at the very first corner of the square.
Procedure
Create a new scenario
There is no downloadable scenario for you to start with--you will be starting this lab from scratch. Create a new scenario called "lab05".
Select the Jeroo palette from the edit menu.
Right-click the Island class and create an instance of it--a completely "empty" island.
Create a Jeroo subclass
Create a Jeroo subclass called SquarePlanter.
Edit the source code for your jeroo subclass. Complete all of the comments.
Modify the constructor for the class so that it takes one int parameter called flowersPerSide. This parameter value represents the number of flowers to use on one side of the square. Don't forget to add the parameter to the Javadoc description of the method.
The first line of your constructor should be a super() call. Write the super() call so that the total number of flowers is passed to the superclass constructor (the total flowers required is four times the flowersPerSide--remember that Java uses an asterisk (*) to mean multiplication).
Note: the complete list of constructors supported by the Jeroo superclass is provided in the Jeroo class documentation.
Add a field (you pick the name) and initialize it in the constructor to keep the number of flowers per side of the square. Remember that the super() call must be the first line in the constructor, so other actions must come after it.
Devise your jeroo's operations
Brainstorm the kinds of methods you would like your new class to support. For example, you might want to have a plantOneSide() method ...
Write down a list of the method ideas you have had. Ignore myProgram() for now (you'll tackle it in the second part of the lab), but instead think in terms of specific methods that carry out identifiable parts of your solution plan (or even the entire plan). Think about which methods are easiest to implement, which are most important to solving the task at hand, and which require others on your list in order to work. Then prioritize the list so that you have an order in which to tackle these methods.
Test and implement your first method
In this lab, we will start out by writing the "test" for a new method first. That way, you'll have a concrete measure of whether or not the method works the way you want, and you can try out your implementation against this measure as you go along.
Create a test class for your jeroo subclass by right-clicking it and selecting "Create test class". Be sure to name your test class correctly.
Add the necessary imports to your test class (see Lab 04 if you need to review the imports needed).
In this lab, let's try to use the same starting conditions for most of our tests. If the starting situation is shared among all of our test cases, then we need a way to refer to the objects in our starting situation that work everywhere, rather than locally inside a single test method. Add a field of type Island to your test class. Give it a name you pick (like "island").
Edit the setUp() method included in your test case. In this method, initialize your "island" field by assigning to it a newly created island object. Remember that the setUp() method runs once for each test case, so every test method gets a fresh copy of this island.
Add a field called "jeroo" of type SquarePlanter. Modify setUp() to create the jeroo with a value you pick (pick a number between, say, 2 and 8), representing the number of flowers to plant per side of the square. Also add a statement in setUp() to add the jeroo to the island at the position you want, say (1, 1) (you pick the starting position--just be sure there is enough room left on the island for the square to fit!).
Add a test method for the first method you intend to work on. In the test method, you have access to the island and jeroo fields. These fields are automatically reset before every test method for you (the setUp() method gets called automatically each time), so you do not have to create new objects in each test method. Instead, all you have to do is invoke the method you intend to work on, and then make relevant assertion(s) describing what you expect to happen. Add these now. Note that your test probably will not compile correctly, since you haven't written the method that the test method calls yet.
Now that your first test is written, switch back to your jeroo subclass and write a stub for the method you decided to test/work on. Then compile and run your test (it should fail).
Now begin implementing your method. Compile and re-run your test as needed to confirm whether or not your method works. Continue until you have it implemented to behave the way you intend.
Remember that to repeat actions, you can use a while loop. You can also use a local variable to keep track of how many times you have done something, or how many you have left, and use an assignment statement to update this count as your loop operates. For example, this loop repeats a basic action a specific number of times:
int hopsToTake = /* some starting value */; while (hopsToTake > 0) { this.hop(); hopsToTake = hopsToTake - 1; // One fewer hops remain now }
You can use this same structure to create loops that count down from a predetermined starting point, or that count up from zero to some limit (you can also use a for loop if you already know how, but we haven't covered them in class yet).
Repeat the testing and implementation of your remaining methods
Repeat Steps 4(f)-(h) for each remaining method in the list you brainstormed. Your test cases should be much less repetitious, since you now have a setUp() method that captures the starting conditions. Remember to re-compile and re-run your tests to double-check your work as you go.
Once you believe you have implemented and tested enough methods to complete the task, you are ready to move on to the next step.
Part 2: Creating a Complete Solution
Procedure
Create a myProgram() method
Edit your jeroo subclass and add a stub for a myProgram() method.
Write a test case in your test class for myProgram(). Its assertions should check that the jeroo plants a square of the desired size, beginning with the first corner where ever the jeroo happens to be located, and planting sides in a clockwise direction. Remember that if the jeroo is created to plant K flowers per side of the square, the square will actually take up K+1 grid cells on each side (because each corner is part of two sides). For example, if the jeroo plants 3 flowers per side, the square itself will be a 4x4 square, the size of the one shown in the figure at the beginning of this lab, which consists of exactly 12 flowers (not 16!).
You may already have a test case that is very similar to this, depending on the methods you designed.
Implement myProgram(). Feel free to run your tests to double-check your progress. If you have chosen your earlier methods well, your implementation of myProgram() should be easy, perhaps even just one line.
For completeness, add one more test case--one that tests myProgram() again, but for a different-sized square. Of course, you have to remember that all test methods in your test class share exactly the same setUp(). Since that method creates the jeroo with a fixed number of flowers (the same for every test case), to test with a different sized square, you must slightly modify the situation for this new test. For example, you can remove the jeroo that is created by setUp() and replace it with a new jeroo with a different number of flowers per side:
// remove the jeroo created by setUp() jeroo.remove(); // create a new jeroo jeroo = new SquarePlanter(/* put your new side choice here */); island.add(jeroo, /* put your desired coordinates here */); // plant the square jeroo.myProgram(); // Add your assertions to check that the square was planted properly
Complete this test to check what you expect, then run it to make sure it confirms what you believe will happen.
Submit to Web-CAT
Make sure your lab05 scenario is the only one you have open, and submit it using the Controls Submit... menu command.
Look carefully at your feedback. In the summary, you will see a table listing each of your source files with a green/red bar by each. If the bar for any file is not completely green, then some code in that file was not exercised by your tests. Click on the file name to view the source code for your file and look for lines that are highlighted in a salmon color. Hover your mouse over highlighted lines for additional information. Add test cases to exercise the code that is highlighted. This may require you to test methods in situations other than those described above.
If you are unsure why points are being deducted in your assignment, or if you are unsure how to address a particular issue, ask a neighbor or a TA.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
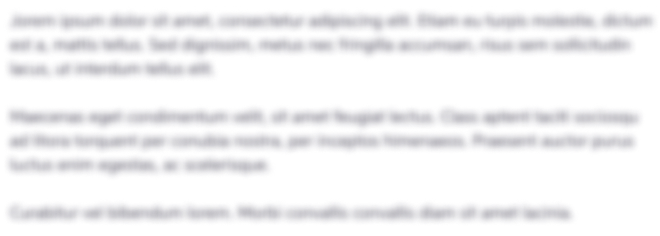
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started