Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
You must perform the following steps to complete this activity: Task 1 : Create an internal structure for list entries, housekeeping variables. Create the structure,
You must perform the following steps to complete this activity: Task : Create an internal structure for list entries, housekeeping variables. Create the structure, internal to the class, that will hold the bid entries. Consider what other variables are needed to help manage the list. Task : Initialize housekeeping variables. Remember to initialize the housekeeping variables in the constructor. Task : Implement append logic. Create code to append a bid to the end of the list. Task : Implement prepend logic. Create code to prepend a bid to the front of the list. Task : Implement print logic. Create code to print all the bid entries in the list to the console. Task : Implement remove logic. Create code to remove the requested bid using the bid ID passed in Task : Implement search logic. Create code to search for the requested bid using the bid ID passed in Return the bid structure if found or an empty bid structure if not found. #include #include #include next; make current the next node delete temp; delete the orphan node Append a new bid to the end of the list void LinkedList::AppendBid bid FIXME : Implement append logic Create new node if there is nothing at the head... new node becomes the head and the tail else make current tail node point to the new node and tail becomes the new node increase size count Prepend a new bid to the start of the list void LinkedList::PrependBid bid FIXME : Implement prepend logic Create new node if there is already something at the head... new node points to current head as its next node head now becomes the new node increase size count Simple output of all bids in the list void LinkedList::PrintList FIXME : Implement print logic start at the head while loop over each node looking for a match output current bidID, title, amount and fund set current equal to next Remove a specified bid @param bidId The bid id to remove from the list void LinkedList::Removestring bidId FIXME : Implement remove logic special case if matching node is the head make head point to the next node in the list decrease size count return start at the head while loop over each node looking for a match if the next node bidID is equal to the current bidID hold onto the next node temporarily make current node point beyond the next node now free up memory held by temp decrease size count return current node is equal to next node Search for the specified bidId @param bidId The bid id to search for Bid LinkedList::Searchstring bidId FIXME : Implement search logic special case if matching bid is the head start at the head of the list keep searching until end reached with while loop current nullptr if the current node matches, return current bid else current node is equal to next node the next two statements will only execute if search item is not found create new empty bid return empty bid Returns the current size number of elements in the list int LinkedList::Size return size;
You must perform the following steps to complete this activity:
Task : Create an internal structure for list entries, housekeeping variables. Create the structure, internal to the class, that will hold the bid
entries. Consider what other variables are needed to help manage the list.
Task : Initialize housekeeping variables. Remember to initialize the housekeeping variables in the constructor.
Task : Implement append logic. Create code to append a bid to the end of the list.
Task : Implement prepend logic. Create code to prepend a bid to the front of the list.
Task : Implement print logic. Create code to print all the bid entries in the list to the console.
Task : Implement remove logic. Create code to remove the requested bid using the bid ID passed in
Task : Implement search logic. Create code to search for the requested bid using the bid ID passed in Return the bid structure if found or an empty bid structure if not found.
#include
#include
#include next; make current the next node
delete temp; delete the orphan node
Append a new bid to the end of the list
void LinkedList::AppendBid bid
FIXME : Implement append logic
Create new node
if there is nothing at the head...
new node becomes the head and the tail
else
make current tail node point to the new node
and tail becomes the new node
increase size count
Prepend a new bid to the start of the list
void LinkedList::PrependBid bid
FIXME : Implement prepend logic
Create new node
if there is already something at the head...
new node points to current head as its next node
head now becomes the new node
increase size count
Simple output of all bids in the list
void LinkedList::PrintList
FIXME : Implement print logic
start at the head
while loop over each node looking for a match
output current bidID, title, amount and fund
set current equal to next
Remove a specified bid
@param bidId The bid id to remove from the list
void LinkedList::Removestring bidId
FIXME : Implement remove logic
special case if matching node is the head
make head point to the next node in the list
decrease size count
return
start at the head
while loop over each node looking for a match
if the next node bidID is equal to the current bidID
hold onto the next node temporarily
make current node point beyond the next node
now free up memory held by temp
decrease size count
return
current node is equal to next node
Search for the specified bidId
@param bidId The bid id to search for
Bid LinkedList::Searchstring bidId
FIXME : Implement search logic
special case if matching bid is the head
start at the head of the list
keep searching until end reached with while loop current nullptr
if the current node matches, return current bid
else current node is equal to next node
the next two statements will only execute if search item is not found
create new empty bid
return empty bid
Returns the current size number of elements in the list
int LinkedList::Size
return size;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
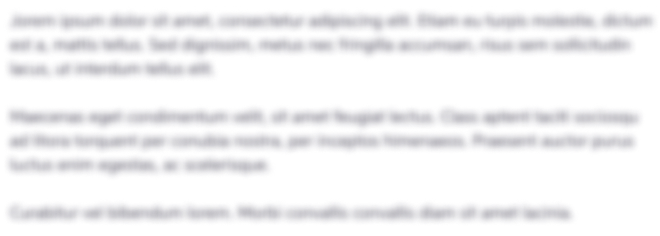
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started