Question
you need to implement a c program that manages rooms in a small hotel that has 5 single rooms (no kids allowed), 10 double rooms
you need to implement a c program that manages rooms in a small hotel that has 5 single rooms (no kids allowed), 10 double rooms (a max of 1 kid is allowed) and 3 studio rooms (a max of 3 kids allowed).
The price for a single room is 350 MAD
o Single rooms are numbered oddly from 1 to 9
The price of a double room 500 MAD
o Double rooms are numbered evenly from 2 to 20
The price of a studio room 700 MAD
o Studio rooms numbered 33, 44, 55 respectively
An extra 50 MAD is applied for every kid
Important points you need to consider during the implementation:
o The files that you will used in this project are for output only the rest of the operations should be carried in the arrays corresponding to room type (you need to use three arrays)
Except for Hotel earning
o You need to use three structures for every room type:
Single room: status(F if Full E if Empty), check in date(DD-MM- YYYY), name of the client, identity number(CIN), room number, and number of night to spend
Double room: status(F if Full E if Empty),check in date, name of the client1, name of client 2, client 1 identity number(CIN), client 2 identity number, room number, status_kid (1 if accompanied with a kid 0 otherwise), and number of night to spend
Studio room: status(F if Full E if Empty), check in date, name of the client1, name of client 2, client 1 identity number(CIN), client 2 identity number, room number, number of kids and the number of night to spend
o Your program should display the following menu with every option implemented with a user defined function (except for quit). Please note that you must respect the concept of input/output argument and local variables
1. Checkavailabilityofaroomtype
2. Checkin
3. Checkout
4. HotelEarning
5. Quit
- Initially you start your code with setting all rooms status to empty and every room must have its assigned room number
- Check in is used to book a room (if available) for a person. Besides from check in, the hotel manager would like to keep a record of people visited the hotel so for every person checked in you need to add the needed information to a text file called history.txt (input chosen are just examples):
Client Name: Hannah Sanders Partner: None
Room type: Single
Number of kids:
0
Number of nights:
2 ------------------------------------------------
- Check out is used to produce the bill for a particular client in the form of a text file
o The txt file produced will be named Client_Name_CIN (ex Hanaa_Talei_A123456.txt) and must contain the following information :
Check in Date:
Client Name:
Room Type:
Number of kids: Number of nights: Total to pay:
Thanks for your visit!
o Remember to free the room!
- Check availability is used to print available rooms for a particular
room type
- Hotel Earning will have access to history.txt and print on screen
the hotel earning in MAD.
- You may use extra user defined functions if needed
- You need to handle possible errors. Example:
o You try to do check out for a person not in the hotel!
o Check in for a room category while no room is available o .......
the problem statement is complete and doesn't have any lacking information, here is a code for this problem, only it contains a lot of issues when you try to run the menu (go through it all to see) and the file history.txt is not used as asked.
#include
} } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
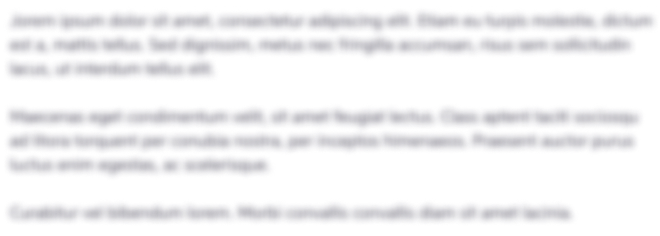
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started