Question
you need to modify the areas in the Queue.h file by adding the necessary code to implement the TODO areas as noted in the comments.
you need to modify the areas in the Queue.h file by adding the necessary code to implement the TODO areas as noted in the comments. The prototype for the functions and everything else in the program must remain unchanged.
Queue.h
#include
using namespace std;
class Queue
{
public:
Queue(int maxSize);
~Queue();
void initialize();
bool empty();
void enqueue(string element);
string dequeue();
string nextElement();
int getSize();
int getMaxSize();
void properties();
void print();
private:
int size; // The current number of items in the queue.
// Again the size is zero based, so
// assume a size of -1 means the queue
// is empty
int maxSize; // The total number of items the queue can
// hold. 0 is the first item, so assume a
// maxSize of -1 means the queue is empty.
string *queue; // Holds a queue of strings
};
/*
* The Queue class implementatoin begins here.
*/
Queue::Queue(int maxSize)
{
this->maxSize = maxSize;
size = -1;
queue = new string[maxSize];
initialize();
}
Queue::~Queue()
{
maxSize = -1;
size = -1;
delete [] queue;
}
void Queue::initialize()
{
// TODO:
// This method deletes all of the items in the
// queue. It should also adjust the size variable
//
// Hint: use a for-loop to set every element in the
// queue to the empty string: "" and set the size to
// -1.
return;
}
bool Queue::empty()
{
// TODO:
// This method checks to see if the queue is empty.
// If it is, (i.e. the size is -1) then return true.
// If the queue is not return false.
return true;
}
void Queue::enqueue(string element)
{
// TODO:
// This method adds the element to the end of the queue.
// You should first check if the value of size+1 does
// not exceed the maximum queue size. If it does do not
// add the element.
return;
}
string Queue::dequeue()
{
// Temporary integer to hold the dequeued element
string element = "";
// TODO:
// This method adds the element to the end of the queue.
// You should first check if the value of size+1 does
// not exceed the maximum queue size. If it does do not
// add the element.
return element;
}
string Queue::nextElement()
{
string element = "[None]";
// TODO:
// This method should only return the element that
// will be dequeued next in the queue . This method
// should not dequeue the element. It should return
// "[None]" if the queue is empty.
return element;
}
int Queue::getSize()
{
return (size+1);
}
int Queue::getMaxSize()
{
return maxSize;
}
void Queue::properties()
{
cout << "Queue properties:" << endl;
cout << " empty = " << empty() << endl;
cout << " max size = " << getMaxSize() << endl;
cout << " current size = " << getSize() << endl;
cout << " next element = " << nextElement() << endl;
cout << endl;
return;
}
void Queue::print()
{
cout << "Queue elements:" << endl;
if (empty())
{
cout << " The queue is empty!" << endl;
cout << endl;
return;
}
while (empty() == false)
{
string element = dequeue();
cout << " " << element << endl;
if (element.size() == 0)
break;
}
cout << endl;
return;
}
Driver.cpp
#include
#include
#include
#include "Queue.h"
using namespace std;
int main(int argc, char **argv)
{
Queue queue(35);
queue.properties();
// Add some nodes to the queue
queue.enqueue("That's");
queue.enqueue("one");
queue.enqueue("big");
queue.dequeue();
queue.enqueue("small");
queue.enqueue("step");
queue.enqueue("for");
queue.enqueue("a");
queue.dequeue();
queue.enqueue("man");
queue.enqueue("one");
queue.enqueue("giant");
queue.enqueue("leap");
queue.enqueue("for");
queue.enqueue("mankind");
queue.dequeue();
queue.properties();
queue.print();
// Initalize the queue
queue.initialize();
queue.properties();
queue.print();
// Add more nodes than the queue can hold
queue.enqueue("We");
queue.enqueue("I");
queue.enqueue("think");
queue.enqueue("we're");
queue.enqueue("going");
queue.dequeue();
queue.enqueue("to");
queue.enqueue("the");
queue.enqueue("moon");
queue.dequeue();
queue.enqueue("because");
queue.enqueue("it's");
queue.enqueue("in");
queue.enqueue("the");
queue.dequeue();
queue.enqueue("nature");
queue.enqueue("of");
queue.enqueue("the");
queue.dequeue();
queue.enqueue("human");
queue.enqueue("being");
queue.enqueue("to");
queue.enqueue("face");
queue.enqueue("challenges");
for (int i=0; i < 5; i++)
{
queue.dequeue();
}
queue.properties();
queue.print();
cout << " ** Press any key to continue ** ";
getchar();
return 0;
}
Output: The output for the program after the functions are implemented should appear as follows:
Queue properties:
empty = 1
max size = 35
current size = 0
next element = [None]
Queue properties:
empty = 0
max size = 35
current size = 10
next element = small
Queue elements:
small
step
for
a
man
one
giant
leap
for
mankind
Queue properties:
empty = 1
max size = 35
current size = 0
next element = [None]
Queue elements:
The queue is empty!
Queue properties:
empty = 0
max size = 35
current size = 11
next element = it's
Queue elements:
it's
in
the
nature
of
the
human
being
to
face
challenges
** Press any key to continue * *
Step by Step Solution
There are 3 Steps involved in it
Step: 1
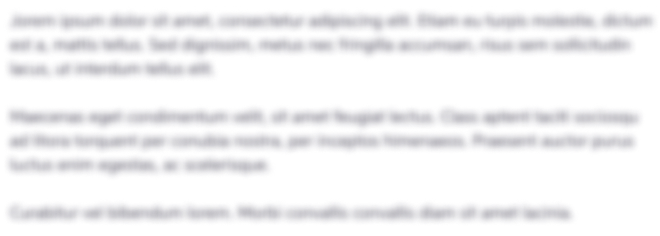
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started