Question
You should add code to the NavigateMaze command to make the robot go from the starting point to the end point. 4 import becker.robots.*; 5
You should add code to the NavigateMaze command to make the robot go from the starting point to the end point.
4 import becker.robots.*; 5 6 class MazeBot extends RobotSE // <-- THIS IS MADE FROM THE RobotSE CLASS. CHECK THE BECKER LIBRARY FOR NEW METHODS 7 { 8 //FIVE Instance Variables will be declared and initialized here 9 //one each for totalMoves, movesWest, movesEast, movesSouth, and MovesNorth 10 11 public MazeBot(City theCity, int str, int ave, Direction dir, int numThings) 12 { 13 super(theCity, str, ave, dir, numThings); 14 } 15 16 // <-- BIG HINT: You might want to create a new method here called movesCounted() that will count 17 // everything it is supposed to by adding to the instance variables before moving, 18 // and then use that instead of move() in the NavigateMaze() method below 19 public void movesCounted() 20 { 21 //THIS METHOD WILL FIRST: 22 23 // Count the total number of moves made 24 // Count the total number of times the robot moved West 25 // Count the total number of times the robot moved East 26 // Count the total number of times the robot moved South 27 // Count the total number of times the robot moved North 28 29 // THEN BE USED TO: 30 31 // move 32 } 33 34 public void printEverything() 35 { 36 // This will print: 37 // The total number of moves made 38 // The total number of times the robot moved West 39 // The total number of times the robot moved East 40 // The total number of times the robot moved South 41 // The total number of times the robot moved North 42 } 43 44 // The isAtEndSpot() method nelow is what's called a 'helper method' 45 // It exists just to make another command (in this case, NavigateMaze) easier to understand. 46 // It does this by replacing some code that otherwise would be in NavigateMaze 47 // with it's name, and doing that work here, instead. 48 // Declaring it "private" means that only the MazeBot is allowed to call upon it. 49 private boolean isAtEndSpot() 50 { 51 return (this.getAvenue() == 9 && this.getStreet() == 10); 52 } 53 54 // THIS IS THE ONE MAIN METHOD WILL DO EVERYTHING (ALTHOUGH IT CAN USE OTHER METHODS 55 // LIKE isAtEndSpot, movesCounted(), printEverything(), ETC) 56 public void NavigateMaze() 57 { 58 // While your robot hasn't yet reached the 'end spot', keep navigating through the Maze and doing its thing 59 while( !this.isAtEndSpot() ) 60 { 61 // HERE IS WHERE ALL THE MAGIC HAPPENS! 62 // The robot will navigate the Maze until it gets to the End Spot. 63 64 // THINGS TO CONSIDER: The robot should put a thing down if it has a thing in the packback; even if it runs out 65 // of things, then robot should still be able to successfully complete the Maze 66 } 67 68 // After completing Maze, print total number of spaces moved and how many times robot moved East, South, West, North. 69 } 70 } 71 72 //################################################################################################### 73 // NO NEED TO TOUCH ANYTHING FROM HERE ON DOWN, EXCEPT TO CHANGE NUMBER OF THINGS IN BACKPACK IN MAIN 74 // The NavigateMaze() method is already set up and called by don the robot down in main 75 //################################################################################################### 76 public class Maze extends Object 77 { 78 private static void MakeMaze(City theCity) 79 { 80 for(int i = 1; i < 11; i++) 81 { 82 // north wall 83 new Wall(theCity, 1, i, Direction.NORTH); 84 85 // Second to north wall 86 if (i <= 9) 87 new Wall(theCity, 1, i, Direction.SOUTH); 88 89 // Third to north wall 90 if (i >= 4) 91 new Wall(theCity, 4, i, Direction.SOUTH); 92 93 // south wall 94 if (i != 9) // (9, 10, SOUTH), is where the 'exit' is 95 new Wall(theCity, 10, i, Direction.SOUTH); 96 97 // west wall 98 if( i != 1) // (1,1, WEST) is where the 'entrance' is 99 new Wall(theCity, i, 1, Direction.WEST); 100 101 // second to west-most wall 102 if (i >= 3 && i < 6) 103 new Wall(theCity, i, 6, Direction.WEST); 104 105 // east wall 106 new Wall(theCity, i, 10, Direction.EAST); 107 } 108 109 // cul-de-sac 110 new Wall(theCity, 3, 10, Direction.WEST); 111 new Wall(theCity, 3, 10, Direction.SOUTH); 112 113 new Wall(theCity, 2, 8, Direction.WEST); 114 new Wall(theCity, 2, 8, Direction.SOUTH); 115 116 new Wall(theCity, 10, 8, Direction.NORTH); 117 new Wall(theCity, 10, 9, Direction.EAST); 118 new Wall(theCity, 10, 9, Direction.NORTH); 119 MakeSpiral(theCity, 8, 9, 3); 120 new Wall(theCity, 8, 10, Direction.SOUTH); 121 122 MakeSpiral(theCity, 10, 5, 4); 123 } 124 125 public static void MakeSpiral(City theCity, int st, int ave, int size) 126 { 127 // We start out building the wall northward 128 // the walls will be built on the east face of the current 129 // intersection 130 Direction facing = Direction.EAST; 131 132 while( size > 0) 133 { 134 int spacesLeft = size; 135 int aveChange = 0; 136 int stChange = 0; 137 switch(facing) 138 { 139 case EAST: 140 stChange = -1; 141 break; 142 case NORTH: 143 aveChange = -1; 144 break; 145 case WEST: 146 stChange = 1; 147 break; 148 case SOUTH: 149 aveChange = 1; 150 break; 151 } 152 153 while(spacesLeft > 0) 154 { 155 new Wall(theCity, st, ave, facing); 156 ave = ave + aveChange; 157 st = st + stChange; 158 spacesLeft--; 159 } 160 // back up one space 161 ave = ave - aveChange; 162 st = st - stChange; 163 164 switch(facing) 165 { 166 case EAST: 167 facing = Direction.NORTH; 168 break; 169 case NORTH: 170 facing = Direction.WEST; 171 size--; 172 break; 173 case WEST: 174 facing = Direction.SOUTH; 175 break; 176 case SOUTH: 177 facing = Direction.EAST; 178 size--; 179 break; 180 } 181 } 182 } 183 184 //########################################################################################### 185 // Main Method 186 //########################################################################################### 187 public static void main(String[] args) 188 { 189 City calgary = new City(12, 12); 190 MazeBot don = new MazeBot(calgary, 1, 1, Direction.EAST, 0); // <-- YOU WILL NEED TO CHANGE THIS FROM ZERO 191 192 Maze.MakeMaze(calgary); 193 194 don.NavigateMaze(); // <-- HERE'S WHERE THE NavigateMaze() method is called. NO NEED TO TOUCH AT ALL 195 196 } 197 }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
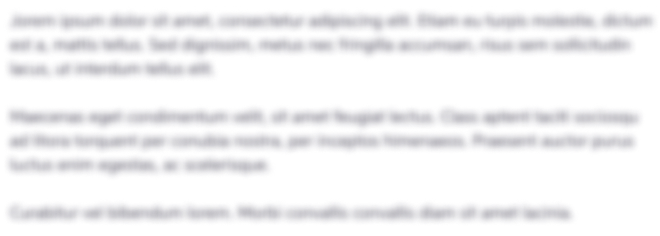
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started