Question
You will be implementing the Singleton Design Pattern to create a Library. Library Class: This is your singleton, you are only able to make one
You will be implementing the Singleton Design Pattern to create a Library.
Library Class:
- This is your singleton, you are only able to make one Library.
- You will create a HashMap to hold the books.
- The HashMap will have String keys (books), and Integer values (numcopies)
When you create a Library, display:
- Creating our Library. Time to begin reading
when you call checkInBook(String bookName, int numToAdd):
- if the book is already in the hashmap, add numToAdd copies,
- otherwise, add book to the hashmap with numToAdd copies
- Then display: ... was added to the library
when you call checkoutBook(String bookName):
- check if the book is in the hashmap, and there is at least one copy
- if so, remove a copy from the library, and display: ...was successfully checked out
- otherwise, display: Sorry ... is not in stock
When you call displayBooks:
- Loop through the HashMap. Displaying:
- Inventory: (followed by each book)
- - ...
- - ...
LibraryDriver:
- The driver will create a Library
- Checkin books to the library
- Checkout books from the library
- Display the library
Driver:
public class LibraryDriver {
public void runLibrary() { Library library = Library.getInstance(); System.out.println(" *********Populating the library**************** "); //Populate initial library library.checkInBook("Anna Karenina", 1); library.checkInBook("Madame Bovary", 2); library.checkInBook("War and Peace", 4); library.checkInBook("The Great Gatsby", 3); library.checkInBook("Lolita", 1); library.checkInBook("Middlemarch", 1); library.checkInBook("The Adventures of Huckleberry Finn", 2); library.checkInBook("The Stories of Anton Chekhov", 1); library.checkInBook("In Search of Lost Time", 3); library.checkInBook("Hamlet", 1); System.out.println(" *********Performing operations on the library**************** "); //checkout books library.checkoutBook("The Great Gatsby"); library.checkoutBook("The Adventures of Huckleberry Finn"); library.checkoutBook("The Adventures of Huckleberry Finn"); library.checkoutBook("Anna Karenina"); library.checkoutBook("Anna Karenina"); library.checkInBook("In Search of Lost Time", 2); library.displayBooks(); }
public static void main(String[] args) { LibraryDriver lDriver = new LibraryDriver(); lDriver.runLibrary(); } }
UML:
Output:
Creating our Library. Time to begin reading.
*********Populating the library****************
Anna Karenina was added to the library Madame Bovary was added to the library War and Peace was added to the library The Great Gatsby was added to the library Lolita was added to the library Middlemarch was added to the library The Adventures of Huckleberry Finn was added to the library The Stories of Anton Chekhov was added to the library In Search of Lost Time was added to the library Hamlet was added to the library
*********Performing operations on the library****************
The Great Gatsby was successfully checked out The Adventures of Huckleberry Finn was successfully checked out The Adventures of Huckleberry Finn was successfully checked out Anna Karenina was successfully checked out Sorry Anna Karenina is not in stock A new copy of In Search of Lost Time was added to the library
Inventory: - Madame Bovary, copies: 2 - Hamlet, copies: 1 - War and Peace, copies: 4 - Lolita, copies: 1 - The Stories of Anton Chekhov, copies: 1 - Anna Karenina, copies: 0 - The Adventures of Huckleberry Finn, copies: 0 - In Search of Lost Time, copies: 5 - The Great Gatsby, copies: 2 - Middlemarch, copies: 1
LibraryDriver Library books: HashMapStep by Step Solution
There are 3 Steps involved in it
Step: 1
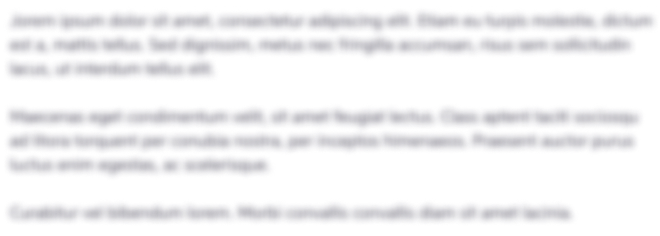
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started