Question
You will be making a digital rolodex using a doubly linked list. Your program will have the following criteria: 1. Each node in the list
You will be making a digital rolodex using a doubly linked list. Your program will have the following criteria: 1. Each node in the list will contain the following information about the person: 1. First Name 2. Last Name 3. Address 4. Phone Number 5. Email Address 6. twitter handle. 2. When the program opens it will read contact information from a text file and create a doubly linked list with the information. 3. The user will be able to: search for and display specific user by any piece of information. Exact matches only is fine. Should be able to navigate through a list if there are multiple results. thus, you probably want to sort first. add a contact to the rolodex delete a user from the rolodex sort the rolodex by any piece of information and navigate through it, displaying one contact at a time. (they will need to be able to exit this navigation mode) quit the program. 4. Upon quitting, all contact information should be written back to the text file. The program should be in C++
#include | |
#include | |
#include | |
using namespace std; | |
struct Address | |
{ | |
int streetNumber; | |
string street; | |
string streetType; | |
string city; | |
string state; | |
int zipcode; | |
}; | |
struct Contact | |
{ | |
string firstName; | |
string lastName; | |
Address address; | |
string phoneNumber; | |
string emailAddress; | |
string twitterHandle; | |
Contact* next; | |
Contact* prev; | |
}; | |
enum sortBy{FIRST=1,LAST,STREET,CITY,STATE,PHONE,EMAIL,TWITTER}; | |
Contact* loadList(string fileName); | |
Contact* remove_(Contact* contact); | |
Contact* insert_(Contact* contact); | |
void saveList(Contact* head, string fileName); | |
Contact* navigateRolodex(Contact* start, Contact* end); | |
void displayContact(Contact* contact); | |
Contact* search(Contact* head); | |
void addContact(Contact* & head); | |
void removeContact(Contact* & head); | |
void sort(Contact* & head); | |
int main() | |
{ | |
string fileName = "contacts.txt"; | |
Contact* rolodex = loadList(fileName); | |
if (rolodex != nullptr) | |
{ | |
int command = 0; | |
while (command != 6) | |
{ | |
cout << "Welcome to Mr. Orme's Rolodex!" << endl; | |
cout << "What would you like to do? " << endl; | |
cout << "\t1. Naviagate through Rolodex" << endl; | |
cout << "\t2. Search for a Contact" << endl; | |
cout << "\t3. Sort Contacts" << endl; | |
cout << "\t4. Add a Contact" << endl; | |
cout << "\t5. Remove a Contact" << endl; | |
cout << "\t6. Quit" << endl; | |
cin >> command; | |
switch (command) | |
{ | |
case 1: | |
navigateRolodex(rolodex, nullptr); | |
break; | |
case 2: | |
search(rolodex); | |
break; | |
case 3: | |
sort(rolodex); | |
break; | |
case 4: | |
addContact(rolodex); | |
break; | |
case 5: | |
removeContact(rolodex); | |
break; | |
case 6: | |
break; | |
default: | |
cout << "Please enter a valid command!" << endl; | |
break; | |
} | |
system("PAUSE"); | |
system("CLS"); | |
} | |
} | |
saveList(rolodex, fileName); | |
} | |
Contact * loadList(string fileName) | |
{ | |
ifstream fin; | |
fin.open(fileName); | |
if (!fin.is_open() && !fin.eof()) | |
{ | |
cout << "ERROR! No rolodex file! Exiting program" << endl; | |
return nullptr; | |
} | |
//create doubly linked list here! | |
fin.close(); | |
return head; | |
} | |
//removes underscores from street name and city name | |
Contact * remove_(Contact * contact) | |
{ | |
for (int i = 0; i < contact->address.street.size(); i++) | |
{ | |
if (contact->address.street[i] == '_') contact->address.street[i] = ' '; | |
} | |
for (int i = 0; i < contact->address.city.size(); i++) | |
{ | |
if (contact->address.city[i] == '_') contact->address.city[i] = ' '; | |
} | |
return contact; | |
} | |
//replace spaces with underscores. | |
Contact * insert_(Contact * contact) | |
{ | |
for (int i = 0; i < contact->address.street.size(); i++) | |
{ | |
if (contact->address.street[i] == ' ') contact->address.street[i] = '_'; | |
} | |
for (int i = 0; i < contact->address.city.size(); i++) | |
{ | |
if (contact->address.city[i] == ' ') contact->address.city[i] = '_'; | |
} | |
return contact; | |
} | |
void saveList(Contact * head, string fileName) | |
{ | |
ofstream fout; | |
fout.open(fileName); | |
//store file here! | |
fout.close(); | |
} | |
Contact* navigateRolodex(Contact * start, Contact * end) | |
{ | |
//this function is to display an entry in the rolodex and navigate from "start" to "end" | |
return nullptr; //change this! | |
} | |
void displayContact(Contact * contact) | |
{ | |
cout << contact->firstName << " " << contact->lastName << endl | |
<< contact->address.streetNumber << " " << contact->address.street << " " << contact->address.streetType << endl | |
<< contact->address.city << ", " << contact->address.state << " " << contact->address.zipcode << endl | |
<< "Phone: " << contact->phoneNumber << " Email: " << contact->emailAddress << " Twitter: " << contact->twitterHandle << endl; | |
} | |
void displayMenu() | |
{ | |
cout << "\t1. First Name" << endl | |
<< "\t2. Last Name" << endl | |
<< "\t3. Street" << endl | |
<< "\t4. City" << endl | |
<< "\t5. State" << endl | |
<< "\t6. Phone Number" << endl | |
<< "\t7. Email" << endl | |
<< "\t8. Twitter Handle" << endl | |
<< "\t9. CANCEL" << endl; | |
} | |
void addContact(Contact *& head) | |
{ | |
//create new contact | |
cout << "Contact Added!!!" << endl; | |
system("PAUSE"); | |
} | |
void removeContact(Contact *& head) | |
{ | |
cout << "How do you want to search for the contact: " << endl; | |
Contact* toDelete = search(head); | |
if (toDelete != nullptr) | |
{ | |
system("CLS"); | |
cout << "Are you sure you want to delete this contact (y/n):" << endl; | |
displayContact(toDelete); | |
char command = ' '; | |
cin >> command; | |
if (command == 'y' || command == 'Y') | |
{ | |
//delete node and update doublly linked list here! | |
cout << "Contacted Deleted!!!" << endl; | |
} | |
else | |
{ | |
cout << "Nothing deleted. Returning to main menu" << endl; | |
} | |
} | |
system("PAUSE"); | |
} | |
void swap(Contact* & head, Contact* & contact1, Contact* contact2) | |
{ | |
//swap the location of two contacts in the rolodex! | |
//used in sorting! | |
} | |
void sort(Contact *& head, sortBy sortKey) | |
{ | |
//if this > 100 lines, there is a better way to do it! | |
} | |
void sort(Contact* & head) | |
{ | |
//this is called by the main program. Doesn't do the sorting! | |
system("CLS"); | |
cout << "Sort by: " << endl; | |
displayMenu(); | |
int command; | |
cin >> command; | |
if (command != 9) sort(head, (sortBy)command); | |
} | |
Contact* search(Contact * head) | |
{ | |
system("CLS"); | |
//options are same as sortBy enum!!! | |
cout << "Search by: " << endl; | |
displayMenu(); | |
//linear search is the way to go! | |
} |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
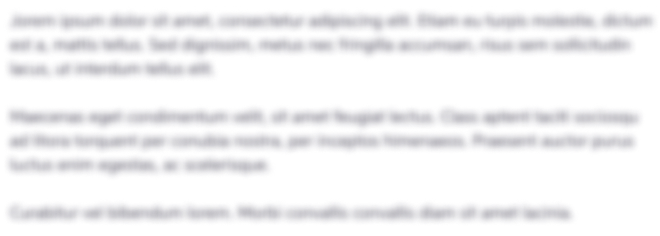
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started