Question
You will create a simple class named Module2 that accepts user inputs and processes user commands. Submit your source code file (Module2.java). The Module2 class
You will create a simple class named Module2 that accepts user inputs and processes user commands. Submit your source code file (Module2.java). The Module2 class should contain the following data fields and methods (note that all data and methods are for objects except for Module2's main method.
Data fields:
A Scanner object named reader (your class will need to import the Scanner class)
Two String objects named username and password
Methods:
A Module2 constructor method that initializes username and password to "" (empty Strings)
A method named getUsername that accepts no parameters and returns a String and:
prints a prompt to the console (i.e., "new username: must start with a capital letter, only contains letters, and at least six characters long")
accepts a user-entered String via the console (use Scanner.nextLine())
if the input String is valid at least 6 characters long, starts with a capital letter, and contains only letters the method will print a confirmation message to the console (i.e., "Thank you.") and return the entered String
if the input String is not valid, the method will print a failure message all applicable errors of of: "username must be at least six characters", "username must start with a capital letter", and "username must contain only letters" and prompt the user to enter a new username.
any subsequent usernames will be checked for validity the same way as the first one
A method named getPassword that accepts no parameters and returns a String and:
prints a prompt to the console (i.e., " new password: must have at least eight characters and contain at least one uppercase letter, at least one lowercase letter, and at least one number")
accepts a user-entered String via the console (use Scanner.nextLine())
if the input String is valid at least 8 characters long and contains at least one number, at least one lowercase letter, and at least one uppercase letter prints a confirmation message to the console (i.e., "Thank you."), and returns the entered String
if the input String is not valid, the method will print a failure message all applicable errors of of: "username must contain at least one number", "username must contain at least one lowercase letter", and "username must contain at least one uppercase letter" and prompt the user to end a new username.
any subsequent passwords will be checked for validity the same way as the first one
A main method that, in order:
Creates a new Module2 object using the constructor from a)
Prints the text starting application: initiating username and password creation" to the console
Calls method b) and assigns the returned String to username
Calls method c) and assigns the returned String to password
Prints the message "username and password validated"
Prints the text ending application to the console
Additional Constraints
The program should be implemented as a single file holding the public class Module2. Comments should be provided at the start of the class file (i.e., above the class definition) giving the class authors name and the date the program was finished. No error-handling beyond the validation checks listed in the getUsername and getPassword methods is required.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
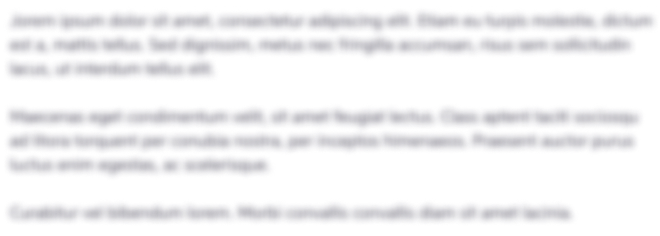
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started