Question
You will create a socket-based server that allows remote users to play the card game. You will implement C++ classes (described below) which encapsulate the
You will create a socket-based server that allows remote users to play the card game. You will implement C++ classes (described below) which encapsulate the POSIX socket system calls needed for the network connection; the .h file for this class is provided. Your application will fork a new process for each incoming connection, so multiple users can play cards with your program simultaneously. You will create a makefile that compiles your code into an executable called cards.
Usage: cards
For example, if I want my card game server to listed to port 11337, I would invoke it with
cards 11337
Valid port numbers are between 11000 and 19999, inclusive. If the port is invalid, your program will print an appropriate error message and end.
The files MySocket.h and MySocket.cpp are provided. The cpp file provides non-functioning stub implementations of the methods of the MySocket and MyConnection classes.
The archive project.zip contains both files: MySocket.h, and MySocket.cpp.
When testing your project, please use 1 + the last 4 digits of your uid as the port number. You can find your uid by typing "id" at the command prompt. Note that this is not your student ID, but the id associated with your account on ale.
MySocket.h
#include
class MyConnection //represents the communication connection between child process and client { private: int connectionFD; public: MyConnection(); //print error, exit(-1); must construct with fd MyConnection(int fd); //MyConnection is just a wrapper for the fd to allow overloading << and >> int read(string&); //return bytes read, -1 on error; will be used in >> int write(const string&); //return bytes written, -1 on error; will be used in << void close(); //close the connection };
class MySocket { private: struct sockaddr_in sock; int socketFD; int portNumber;
public: MySocket(); //print error, exit(-1); must construct with port # MySocket(int portNumber); //creates the server listening socket //must implement =, copy, destructor MySocket& operator=(MySocket&); MySocket(const MySocket&); ~MySocket(); int listen(); //call once to start listening MyConnection accept(); //call repeatedly when connecting to client; returns client stream void close(); };
MyConnection& operator<<(MyConnection&, const string&); MyConnection& operator>>(MyConnection&, string&); #endif
MySocket.cpp
#include "MySocket.h" #includeusing namespace std; MySocket::MySocket(){exit(-1);} MySocket::MySocket(int p):portNumber(p){} MyConnection::MyConnection(){exit(-1);} MyConnection::MyConnection(int fd):connectionFD(fd){} MySocket& MySocket::operator=(MySocket& m){return m;} MySocket::MySocket(const MySocket&){} MySocket::~MySocket(){} int MyConnection::read(string& s){return 0;} int MyConnection::write(const string& s){return 0;} int MySocket::listen(){return 0;} MyConnection MySocket::accept(){return 0;} void MySocket::close(){} void MyConnection::close(){} MyConnection& operator<<(MyConnection& me, const string& s){return me;} MyConnection& operator>>(MyConnection& me, string& s){return me;}
server2.cpp
#include
void doprocessing (MyConnection& c);
int main( int argc, char *argv[] ) { int pid; MySocket m(19999); m.listen(); while (1) { MyConnection c = m.accept(); /* Create child process */ pid = fork(); if (pid < 0) { perror("ERROR on fork"); exit(1); } if (pid == 0) { /* This is the client process */ m.close(); doprocessing(c); exit(0); } else { c.close(); }
} /* end of while */ }
void doprocessing (MyConnection& c) { int n; string inS; while (true) { c >> inS; cout << "Here is the message: " << inS << " "; c << "I got your message: " << inS << " "; } if (inS.length() == 0) exit(0); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
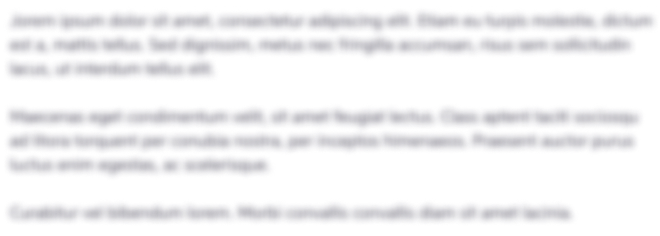
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started