Question
You will demonstrate the ability to create inherited classes. Write a parent class called Employee. 1. Employee must contain the following variables: string m_fname //First
You will demonstrate the ability to create inherited classes. Write a parent class called Employee. 1. Employee must contain the following variables: string m_fname //First name string m_lname //Last name string m_ssn //Social security number double *m_paychecks //A dynamic array containing the total pay int m_numPaychecks //The number of paychecks recorded in m_paychecks 2. It must have a constructor that accepts no arguments, and a constructor that accepts (string fname, string lname, string ssn) 3. It should have getter and setter functions to update the string variables. 4. It should have a pure function that adds a paycheck. This function should resize the dynamic array if the array is full. 5. Declare a pure virtual void function called printPayInformation(). 6. Since this class has dynamic variables, it should appropriately implement an overloaded assignment operator, copy constructor, and destructor. Write a derived class called HourlyEmployee: 1. HourlyEmployee should contain two additional variables: double m_hourlyRate double m_hoursWorked 2. It must have a constructor that accepts no arguments, and a constructor that accepts (string fname, string lname, string ssn, double hourlyRate). Both constructors should explicitly call the parent class constructor and populate any variables passed in. 3. Write a function called finalizeHours(double hoursWorked). This should put the total dollar amount of the paycheck into the dynamic array (you'll need to use that addPaycheck function in the parent class) and it should update the m_hoursWorked function. 4. Override the parent class printPayInformation() function to print the pay history (all the paychecks in the dynamic array) as well as the most recent hours worked and hourly wage. example: Employee Name: Bob Smith Employee SSN: 123-45-6789 Current Hourly Rate: 16.32 Current Hours Worked: 15.1 Paycheck #1: 502.10 Paycheck #2: 400.32 Paycheck #3: 342.89 Paycheck #4: 239.63 Paycheck #5: 246.44 5. Copy constructor, assignment operator, and destructor should be handled appropriately. Write a main function that creates at least 3 hourly employees, assigns them at least 4 paychecks each, and prints their pay details. Submit all .cpp and all .h files compressed into a zip file to Blackboard.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
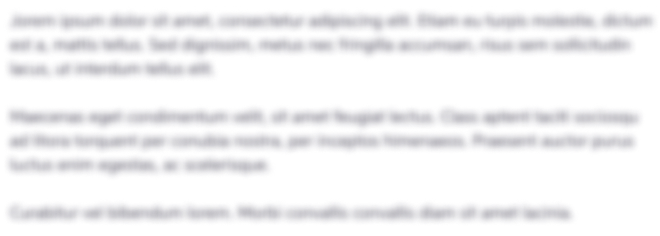
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started