Question
You will get practice in this assignment in the following areas in C/C++: Writing a simple C++ program Compiling and linking If Statements and Switch
You will get practice in this assignment in the following areas in C/C++:
- Writing a simple C++ program
- Compiling and linking
- If Statements and Switch Statements
- Simple arithmetic statements
- Standard Input and output
- Variable Declaration
- Simple functions
- Good programming practices
Description:
You are to write a simple C++ program that gives the user the ability to perform the following calculations:
- Square Root (cannot be a negative number)
- Cube
- Natural logarithm(cannot be a negative number)
- Inverse
- Absolute value
Programming Specifications:
Your program will display a menu and give the user the choice of 6 options. To calculate the square root of a number, the cube of a number, the natural logarithm of a number, the inverse of a number, the absolute value of a number, or to exit the program.
- All variables and constants must be declared as floating point (double) .
- The main routine of your program will be primarily the switch statement that controls the menu. This must be put into a loop that continues to execute until the user selects the option to exit.
- Your program can assume that only digits will be entered and not letters or special characters.
- When the user selects the option to calculate the Square Root, Cube, Natural log, Inverse or Absolute value of a number, an appropriately named function without parameters that you will create yourself will be called which will prompt the user to enter a floating point decimal number, calculate the expected value, and output the results to the console window.
- If the user inputs an incorrect menu option, your program will ignore it and redisplay the menu.
- If the user inputs an incorrect value, such as a negative number for the Square Root, you will display a message and return from the function. You are not required to ask them to re-enter the number. You may use exceptions to catch the error if desired but it is not required.
- Enter the menu options as single characters and treat them like characters in the
Required Algorithms
In order to make this program work, you will have to include the C++ math library.
#include
You will also need to use the following functions that are contained in the math library. They are available to you just by including the cmath library. Here are sample calls to the functions. The following code is just an "example" of how to use the functions and does not necessarily represent a solution to the programming lab assignment. However, it is an example of the cmath functions you will need to use when creating your own solution to this programming lab assignment.
double inputnumber;
cout << "Enter a floating point number" ;
cin >> inputnumber;
cout << "The square root of " << inputnumber << " is " << sqrt(inputnumber) << endl;
cout << "The natural log of " << inputnumber << " is " << log(inputnumber) << endl;
cout << inputnumber << " raised to the third power is " << pow(inputnumber,3) << endl;
cout << "The absolute value of " << inputnumber << " is " << fabs(inputnumber) << endl;
cout << "The inverse of " << inputnumber << " is " << 1.0/inputnumber << endl;
Grading Criteria:
- The program compiles. If the program does not compile no further grading can be accomplished. Programs that do not compile will receive a zero.
- (25 Points) The program executes without exception and produces output. The grading of the output cannot be accomplished unless the program executes.
- (25 Points) The program produces the correct output.
- (25 Points) The program specifications are followed.
- (5 Points) your program must have a switch statement to control the menu
- (5 Points) your program must continue to work until exit is selected.
- (5 Points) Incorrect menu options are ignored
- (5 Points) Your program recognizes improper numbers ( negative on square root and natural log)
- (5 Points) Your switch statement calls function that returns the appropriate value.
- (10 Points)The program is documented (commented)
- (5 Points)Use constants when values are not to be changed
- (5 Points)Use proper indentation
- (5 Points)Use good naming standards
Program Shell:
#include
#include
using namespace std;
/***********************************************************************/
// Function Prototypes go here (i.e. function header with semicolon) *
/***********************************************************************/
int main(void)
{
/***********************************************************************/
/* Your switch statement goes here. */
/************************************************************************/
return 0;
}
/*********************************************************************/
/* Your functions that do the square root, absolute value, log, inverse, and cube go here.*/
/**********************************************************************/
Sample Program Run:
M E N U
1 - Calculate Square Root
2 - Calculate Cube
3 - Calculate Natural Logarithm
4 - Calculate Inverse
5 - Calculate Absolute Value
0 - Exit Program
________________________________________
Enter Menu Option = 1
Enter in a non-negative decimal number:
The square root of 27 is 5.19615
M E N U
1 - Calculate Square Root
2 - Calculate Cube
3 - Calculate Natural Logarithm
4 - Calculate Inverse
5 - Calculate Absolute Value
0 - Exit Program
________________________________________
Enter Menu Option = 2
Enter in a decimal number:
The Cube of 3 is 27.0
M E N U
1 - Calculate Square Root
2 - Calculate Cube
3 - Calculate Natural Logarithm
4 - Calculate Inverse
5 - Calculate Absolute Value
0 - Exit Program
________________________________________
Enter Menu Option = 3
Enter in a decimal number: 25.6
The Natural Log of 25.6 is 3.24259
Step by Step Solution
There are 3 Steps involved in it
Step: 1
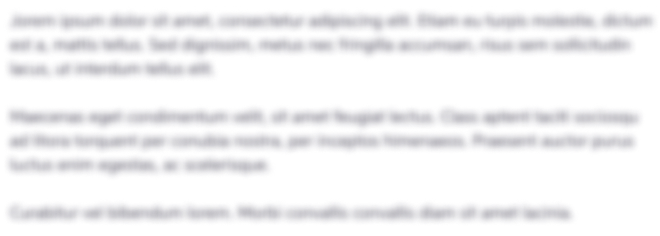
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started