Question
You will modify the WordList class (see attached files) so that is uses an Array to store words instead of an ArrayList. Note: See below
You will modify the WordList class (see attached files) so that is uses an Array to store words instead of an ArrayList.
Note: See below the code and cut and pasted into BlueJ
Modify as follows:
- Remove the import statement
- Change the type for the field words so that it is an array of String objects
- Add a size field to keep track of the number of words in the list
- Modify the constructor to initialize words to an array of size 10 and initialize the size field.
- Modify the getSize method to return the size field
- Modify the add method to do the following:
- If the array is full, get a new array that is double the size of the current one and copy the contents of the old array to the new one. You can do this yourself or you can look for the appropriate version of the copyOf method in the Arrays class
- Add the new word to the end of the list
- Adjust the size field.
- Modify the remove method to do the following:
- If the index is invalid, print an appropriate error message and return null
- If the index is valid, save the word in a local variable to be returned at the end.
- "Remove" the word by sliding down the elements at positions index + 1 through end of the list
- Adjust the size field
- Return saved word that was removed
- Modify the printWords method as needed
import java.util.ArrayList;
public class WordList
{
private ArrayList
/**
* Constructor for objects of class WordList
*/
public WordList()
{
words = new ArrayList<>();
}
/**
* Method to return the current size of the list
*
* @return the number of words in the list
*/
public int getSize()
{
return words.size();
}
/**
* Method to add a new word to the end of the list
*
* @param newWord the word to be added
*/
public void add(String newWord)
{
words.add(newWord);
}
/**
* Method to remove a word at the specified index
*
* @param index the position of the word to be removed
* @return the word that was removed
*/
public String remove(int index)
{
return words.remove(index);
}
public void printWords()
{
for(String word : words)
{
System.out.println(word);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
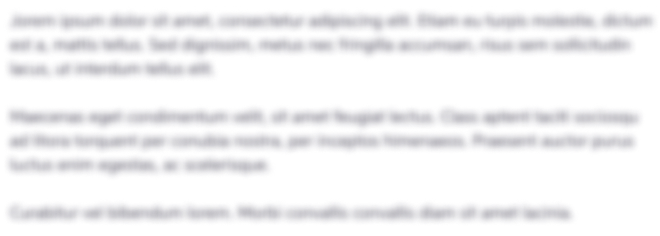
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started