Question
you will need to add Song, PlayList and MusicPlayer classes . You will be extending the functionality of this program. You will also find songdata.txt
you will need to add Song, PlayList and MusicPlayer classes . You will be extending the functionality of this program. You will also find songdata.txt that should go into your project. (Song, PlayList and MusicPlayer, and songdata are on the bottom)
In MusicPlayer.java:
Task 1 of 2 for MusicPlayer. Add this method :
public static void printPlayList(PlayList pl) { // see the comments in the actual code // for what the output looks like
}
Task 2 of 2 for MusicPlayer . At B: Write the missing lines of code that tokenizes a line of text from the file and creates a new Song instance. Add each song to your PlayList. Test: Print playlist after parsing the file.
In PlayList.java:
Implement these new methods:
public void playPrevSong() { // Task 1 of 7 of PlayList: This method plays the previous song
}
public int size() { // Task 2 of 7 of PlayList: return the size of the playList
ArrayList
}
public Song getSong(int i) { // Task 3 of 7 of PlayList: dont forget to test for errors
}
public Song getCurrentSong() { // Task 4 of 7 of PlayList: Return the current Son
}
public void playRandomSong() { // Task 5 of 7 of PlayList: Generate an appropriate Random number // and set the current song to it
}
public void shuffle() { // Task 6 of 7 of PlayList: Find a way to shuffle an ArrayList // Hint: Use Google or use ArrayList Javadoc // Extra credit if you do it the hard way
}
public void insertSong(Song s, int pos) { // Task 7 of 7 of PlayList: I: Implement this method // test for these conditions:
// pos < 0 add at position 0 // if pos > last index in array add to end // otherwise add at position pos using ArrayList method
}
You do not have to turn in Console output for this problem but be sure to include your modified MusicPlayer and PlayList Java files. I will run your code for this problem to see if the methods produce correct output. So I recommend that you test them before turning them in.
Songdata.txt
2016,Ingrid Michaelson,It Doesn't Have to Make Sense,Light Me Up
2016,Matt Simons,Catch & Release,Catch & Release (Deepend remix)
2016,Daya,Sit Still Look Pretty
2016,Sabrina Carpenter,EVOLution,On Purpose
2016,Listenbee,Until We Go Down
2017,Dua Lipa,Dua Lipa,Blow Your Mind (Mwah)
2015,Carly Rae Jepsen,E-MO-TION,I Really Like You
2017,DNCE,Kissing Strangers
2016,Britney Spears,Glory,Invitation
2016,Lady Gaga,Joanne,A-YO
2016,DNCE,DNCE,Cake by the Ocean
2016,Olly Murs,You Don't Know Love
2016,Petite Meller,The Flute,Baby Love
2016,Demi Lovato,Body Say
2015,Ed Sheeran,X (Wembley Edition),Lay It All On Me
2016,DJ Snake,Encore,Let Me Love You
2016,Graham Candy,Plan A,Glowing in the Dark
2016,David Guetta,Bang My Head (Robin Schulz remix)
Song.java
public class Song {
private String artist;
private String title;
private int year;
public Song(String artist, String title, int year) {
this.artist = artist;
this.setTitle(title);
this.setYear(year);
}
public String toString() {
return this.getTitle() + " by " + this.getArtist() + " (" + this.getYear() + ")";
}
/**
* @return the artist
*/
public String getArtist() {
return this.artist;
}
/**
* @param artist the artist to set
*/
public void setArtist(String artist) {
this.artist = artist;
}
/**
* @return the title
*/
private String getTitle() {
return title;
}
/**
* @param title the title to set
*/
private void setTitle(String title) {
this.title = title;
}
/**
* @return the year
*/
private int getYear() {
return year;
}
/**
* @param year the year to set
*/
private void setYear(int year) {
this.year = year;
}
}
PlayList.Java
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.Random;
public class PlayList {
private String name;
private ArrayList
private int currSong = 0;
private Random ranGen = new Random();
public PlayList(String name) {
this.name = name;
}
public String getName() {
return this.name;
}
public String toString() {
return "Playing: " + playList.get(currSong);
}
public void addSong(Song songToAdd) {
playList.add(songToAdd);
}
public void playNextSong() {
if (this.currSong + 1 < playList.size()) {
this.currSong++;
}
else {
this.currSong = 0;
}
}
public void playPrevSong() {
// C: Implement this method
}
public int size() {
// D: Implement this method
// return the size of the playList array
}
public Song getSong(int i) {
// E: Implement this method
// test for failures
}
public Song getCurrentSong() {
// F: Implement this method
}
public void playRandomSong() {
// G: Implement this method
}
public void shuffle() {
// H: Implement this method
// Do this anyway you want but first google shuffling an ArrayList
}
public void insertSong(Song s, int pos) {
// I: Implement this method
// test for these conditions:
// pos < 0 add at position 0
// if pos > last index in array add to end
// otherwise add at position pos using ArrayList method
}
}
MusicPlayer.java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class MusicPlayer {
public static void main(String[] args) {
// Make a PlayList
PlayList myPlayList = new PlayList("Student's Play List");
File songFile = new File("songdata.txt");
Scanner fileReader;
try {
fileReader = new Scanner(songFile);
int songCounter = 0;
while (fileReader.hasNextLine()) {
String aLine = fileReader.nextLine();
String[] tokens = aLine.split(",");
// Lines look this: 2016,David Guetta,single,Bang My Head (Robin Schulz remix)
// B: Your code goes here. Create a Song from tokens and add it to mPlayList
// Here!
}
}
catch (FileNotFoundException e) {
e.printStackTrace();
}
// Tests of the PlayList
// list the songs in the playList
printPlayList(myPlayList);
// Print the currently playing song
System.out.println("Currently Playing: " + myPlayList.getCurrentSong());
// go to the next song
myPlayList.playNextSong();
System.out.println("Currently Playing: " + myPlayList.getCurrentSong());
myPlayList.playNextSong();
System.out.println("Currently Playing: " + myPlayList.getCurrentSong());
// Song oldyMoldy = new Song("The Beatles", "Norwegian Wood", 1965);
// myPlayList.insertSong(oldyMoldy, 10);
// printPlayList(myPlayList);
// myPlayList.shuffle();
// printPlayList(myPlayList);
}
public static void printPlayList(PlayList pl) {
// A: Finish this printPlayList method
// It should produce this output:
/*--------------oz's Playlist--------------------
It Doesn't Have to Make Sense by Ingrid Michaelson (2016)
Catch & Release by Matt Simons (2016)
Sit Still Look Pretty by Daya (2016)
EVOLution by Sabrina Carpenter (2016)
Until We Go Down by Listenbee (2016)
Dua Lipa by Dua Lipa (2017)
E-MO-TION by Carly Rae Jepsen (2015)
Kissing Strangers by DNCE (2017)
Glory by Britney Spears (2016)
Joanne by Lady Gaga (2016)
DNCE by DNCE (2016)
You Don't Know Love by Olly Murs (2016)
The Flute by Petite Meller (2016)
Body Say by Demi Lovato (2016)
X (Wembley Edition) by Ed Sheeran (2015)
Encore by DJ Snake (2016)
Plan A by Graham Candy (2016)
Bang My Head (Robin Schulz remix) by David Guetta (2016)
------------------------------------------------------- */
// Your Code Goes Here
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
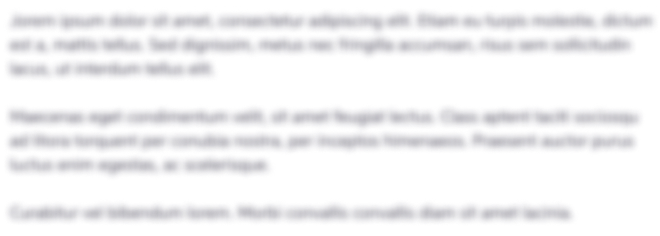
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started