Question
You will need to create and ArrayList class that implements a dynamically resizable array using templates. You should implement the following methods: //Default Constructor --
You will need to create and ArrayList class that implements a dynamically resizable array using templates. You should implement the following methods:
//Default Constructor -- create a starting array with 10 slots/elements //Constructor (int size) -- create a starting array with given size //Copy constructor -- deep copy //Assignment operator -- deep copy //Destructor -- frees dynamically allocated memory
//set(int position, T value) sets the value at position //get(int position) gets the value at position //append(T value) appends the value to the end of the array -- extend the array if necessary //insert(int position, T value) inserts value at position -- moves everything else down one -- extend the array if necessary
--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
#ifndef ArrayList_hpp #define ArrayList_hpp #include
#define DEFAULT_SIZE 10
template
public: ArrayList(); //array list ArrayList(const ArrayList& other); ArrayList& operator=(const ArrayList& other); ~ArrayList(); void append(T value); //"append" adds value to the end of the array //grows the array as needed. void print(std::ostream& out) private: void copyList(T* old, int size); T* arr; int size; int capacity;
};
template
template
template
template
template
void ArrayList
#endif /* ArrayList_hpp */
--------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Here is what I have so far. My int main() is in my .cpp file, but I could use help writing out a couple of test cases.
As far as the code I have now, I am not confident in my code, please correct me and explain if something is wrong.
Also, I am still trying to undersatnd the concept of pointers and how they work. I have not fully grasped this concept, I would really appreciate comments in regards to the pointers and how they are working with this piece of code especially when it comes to knowing where and when to use the astrix(*) symbol and the (&) symbol.
I would appreciate the extra explanation/comments in the code to understand the funtionality of how the pointers are working.
I will leave a thumbs and comment, thank you for your time!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
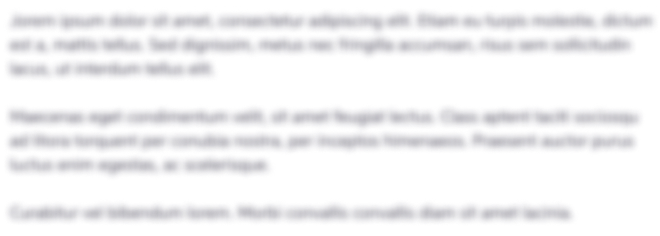
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started