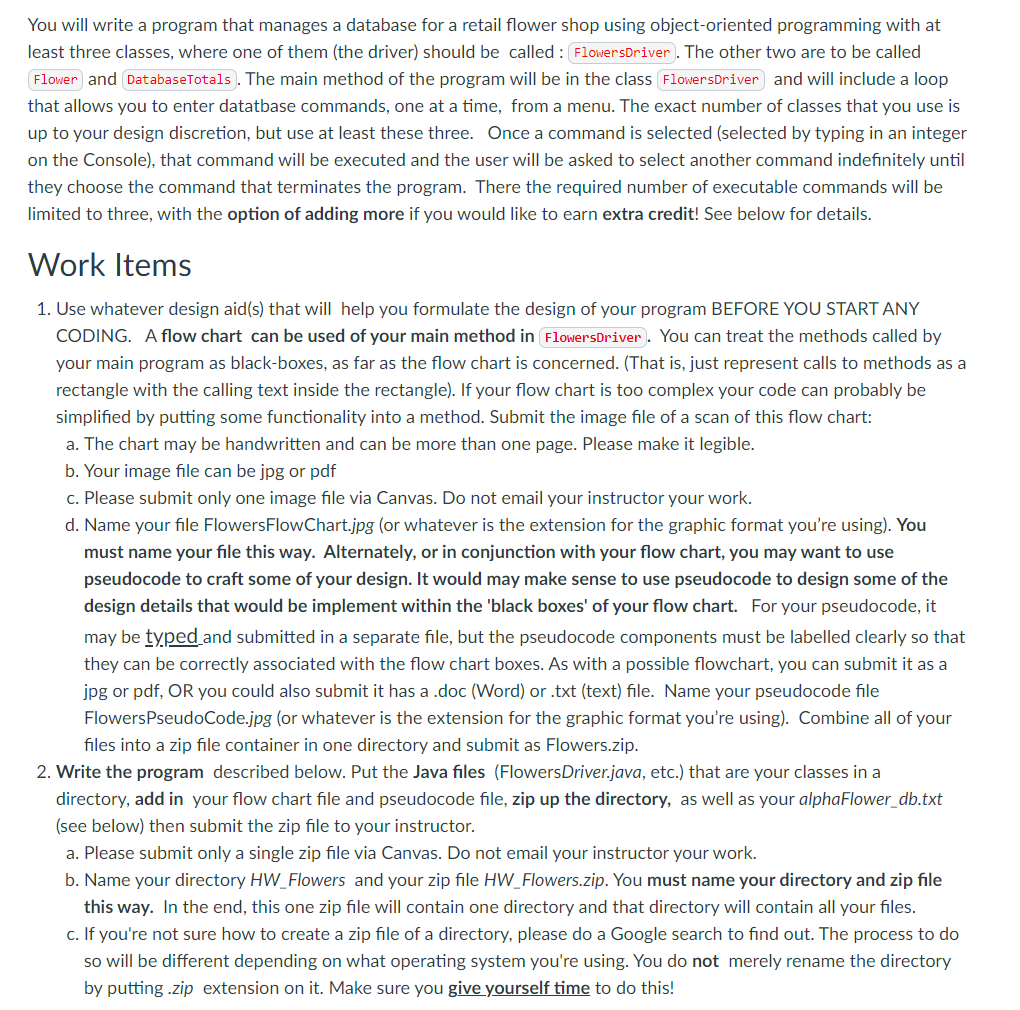
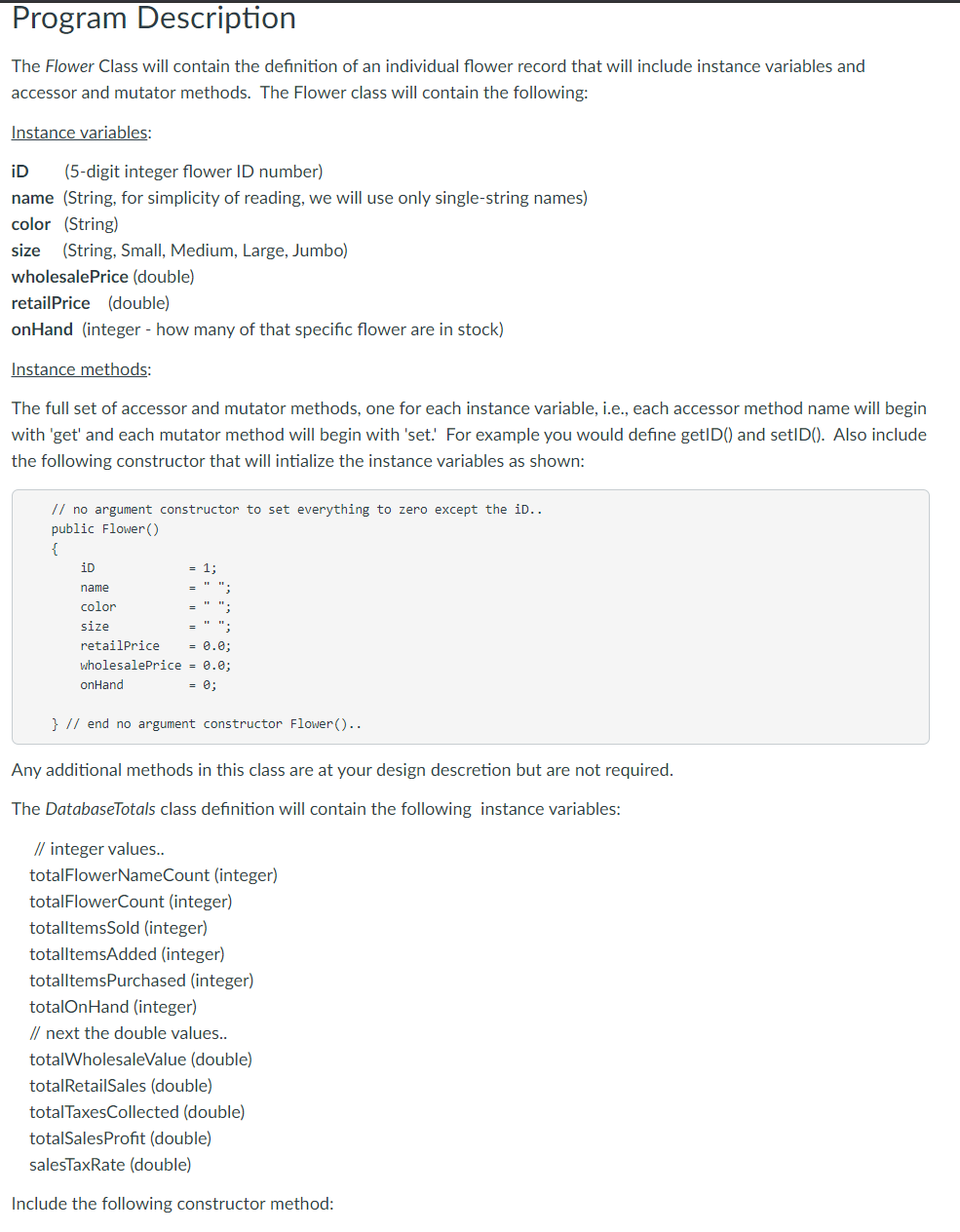
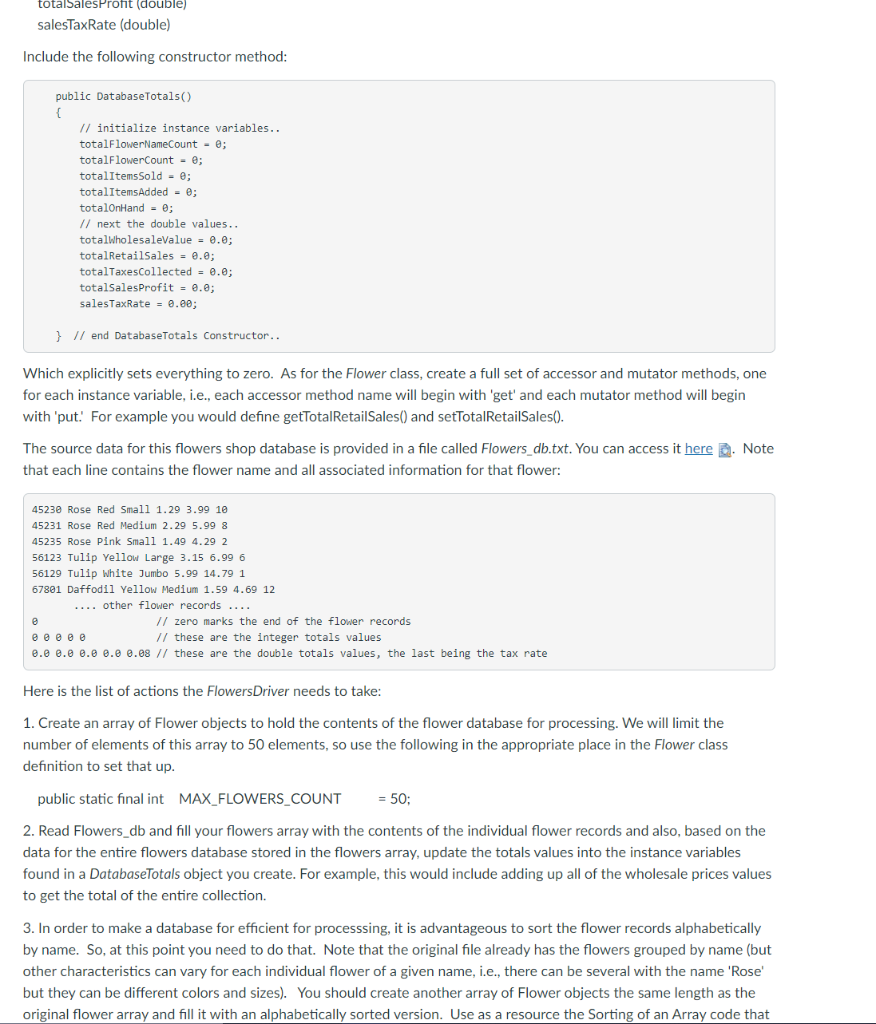
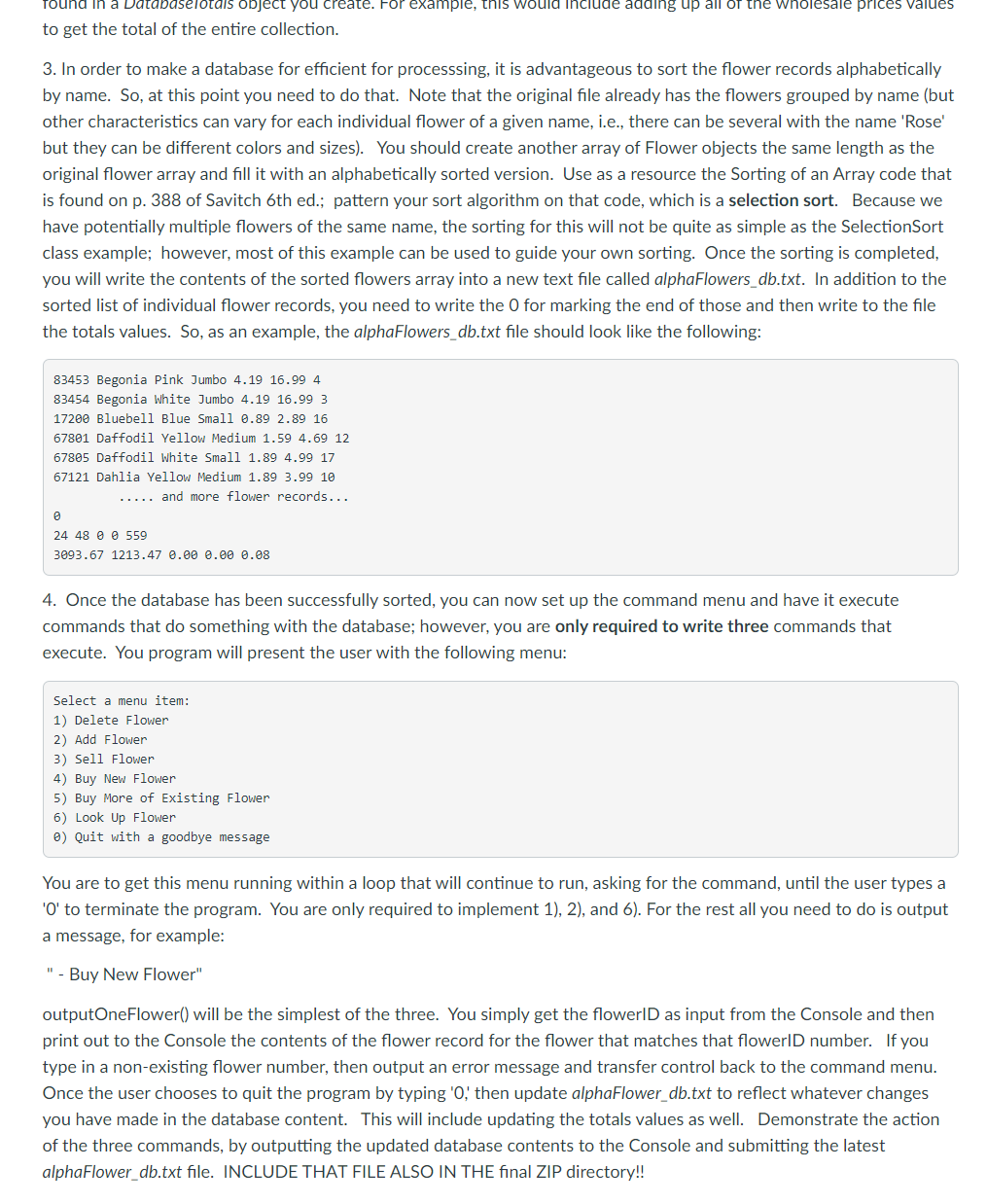
You will write a program that manages a database for a retail flower shop using object-oriented programming with at least three classes, where one of them (the driver) should be called : FlowersDriver. The other two are to be called Flower and DatabaseTotals. The main method of the program will be in the class FlowersDriver and will include a loop that allows you to enter datatbase commands, one at a time, from a menu. The exact number of classes that you use is up to your design discretion, but use at least these three. Once a command is selected (selected by typing in an integer on the Console), that command will be executed and the user will be asked to select another command indefinitely until they choose the command that terminates the program. There the required number of executable commands will be limited to three, with the option of adding more if you would like to earn extra credit! See below for details. Work Items 1. Use whatever design aid(s) that will help you formulate the design of your program BEFORE YOU START ANY CODING. A flow chart can be used of your main method in FlowersDriver. You can treat the methods called by your main program as black-boxes, as far as the flow chart is concerned. (That is, just represent calls to methods as a rectangle with the calling text inside the rectangle). If your flow chart is too complex your code can probably be simplified by putting some functionality into a method. Submit the image file of a scan of this flow chart: a. The chart may be handwritten and can be more than one page. Please make it legible. b. Your image file can be jpg or pdf c. Please submit only one image file via Canvas. Do not email your instructor your work. d. Name your file Flowers Flow Chart.jpg (or whatever is the extension for the graphic format you're using). You must name your file this way. Alternately, or in conjunction with your flow chart, you may want to use pseudocode to craft some of your design. It would may make sense to use pseudocode to design some of the design details that would be implement within the 'black boxes' of your flow chart. For your pseudocode, it may be typed and submitted in a separate file, but the pseudocode components must be labelled clearly so that they can be correctly associated with the flow chart boxes. As with a possible flowchart, you can submit it as a jpg or pdf, OR you could also submit it has a .doc (Word) or .txt (text) file. Name your pseudocode file Flowers PseudoCode.jpg (or whatever is the extension for the graphic format you're using). Combine all of your files into a zip file container in one directory and submit as Flowers.zip. 2. Write the program described below. Put the Java files (FlowersDriver.java, etc.) that are your classes in a directory, add in your flow chart file and pseudocode file, zip up the directory, as well as your alphaFlower_db.txt (see below) then submit the zip file to your instructor. a. Please submit only a single zip file via Canvas. Do not email your instructor your work. b. Name your directory HW_Flowers and your zip file HW_Flowers.zip. You must name your directory and zip file this way. In the end, this one zip file will contain one directory and that directory will contain all your files. c. If you're not sure how to create a zip file of a directory, please do a Google search to find out. The process to do so will be different depending on what operating system you're using. You do not merely rename the directory by putting.zip extension on it. Make sure you give yourself time to do this! Program Description The Flower Class will contain the definition of an individual flower record that will include instance variables and accessor and mutator methods. The Flower class will contain the following: Instance variables: iD (5-digit integer flower ID number) name (String, for simplicity of reading, we will use only single-string names) color (String) size (String, Small, Medium, Large, Jumbo) wholesale Price (double) retailPrice (double) onHand (integer - how many of that specific flower are in stock) Instance methods: The full set of accessor and mutator methods, one for each instance variable, i.e., each accessor method name will begin with 'get' and each mutator method will begin with 'set! For example you would define getID() and set|D(). Also include the following constructor that will intialize the instance variables as shown: // no argument constructor to set everything to zero except the iD.. public Flower) { iD 1: name color size retailPrice = 0.0; wholesalePrice = 0.0; on Hand = 0; } // end no argument constructor Flower().. Any additional methods in this class are at your design descretion but are not required. The Database Totals class definition will contain the following instance variables: // integer values.. totalFlower NameCount (integer) totalFlowerCount (integer) totalltemsSold (integer) totalltemsAdded (integer) totalltemsPurchased (integer) totalOnHand (integer) // next the double values.. totalWholesaleValue (double) totalRetailSales (double) totalTaxes Collected (double) totalSalesProfit (double) salesTaxRate (double) Include the following constructor method: totalSalesPront (double) salesTaxRate (double) Include the following constructor method: public DatabaseTotals() { // initialize instance variables.. totalFlowerNameCount - @; totalFlowerCount - @; totalItemsSold - e; totalItems Added 0; totalonHand = e: // next the double values.. " totalWholesalevalue = 0.0; totalRetailSales = 0.8; totalTaxesCollected = 0.8; totalSalesProfit = 0.0; salesTax Rate = e.ee; } // end DatabaseTotals Constructor.. Which explicitly sets everything to zero. As for the Flower class, create a full set of accessor and mutator methods, one for each instance variable, i.e., each accessor method name will begin with 'get' and each mutator method will begin with 'put' For example you would define getTotalRetail Sales() and setTotalRetail Sales(). The source data for this flowers shop database is provided in a file called Flowers_db.txt. You can access it here. Note that each line contains the flower name and all associated information for that flower: 45230 Rose Red Small 1.29 3.99 10 45231 Rose Red Medium 2.29 5.99 8 45235 Rose Pink Small 1.49 4.29 2 56123 Tulip Yellow Large 3.15 6.99 6 56129 Tulip white Jumbo 5.99 14.79 1 67801 Daffodil Yellow Medium 1.59 4.69 12 ... other flower recor // zero marks the end of the flower records eeeee // these are the integer totals values 0.0 0.0 0.0 0.0 0.08 // these are the double totals values, the last being the tax rate records Here is the list of actions the Flowers Driver needs to take: 1. Create an array of Flower objects to hold the contents of the flower database for processing. We will limit the number of elements of this array to 50 elements, so use the following in the appropriate place in the Flower class definition to set that up. public static final intMAX_FLOWERS_COUNT = 50; 2. Read Flowers_db and fill your flowers array with the contents of the individual flower records and also, based on the data for the entire flowers database stored in the flowers array, update the total values into the instance variables found in a DatabaseTotals object you create. For this would include addi up all of the wholesale prices values to get the total of the entire collection. 3. In order to make a database for efficient for processsing, it is advantageous to sort the flower records alphabetically by name. So, at this point you need to do that. Note that the original file already has the flowers grouped by name (but other characteristics can vary for each individual flower of a given name, i.e., there can be several with the name 'Rose but they can be different colors and sizes). You should create another array of Flower objects the same length as the original flower array and fill it with an alphabetically sorted version. Use as a resource the Sorting of an Array code that found in a Databaselotais object you create. For example, this would include adding up all of the wholesale prices values to get the total of the entire collection. 3. In order to make a database for efficient for processsing, it is advantageous to sort the flower records alphabetically by name. So, at this point you need to do that. Note that the original file already has the flowers grouped by name (but other characteristics can vary for each individual flower of a given name, i.e., there can be several with the name 'Rose' but they can be different colors and sizes). You should create another array of Flower objects the same length as the original flower array and fill it with an alphabetically sorted version. Use as a resource the Sorting of an Array code that is found on p. 388 of Savitch 6th ed.; pattern your sort algorithm on that code, which is a selection sort. Because we have potentially multiple flowers of the same name, the sorting for this will not be quite as simple as the SelectionSort class example; however, most of this example can be used to guide your own sorting. Once the sorting is completed, you will write the contents of the sorted flowers array into a new text file called alphaFlowers_db.txt. In addition to the sorted list of individual flower records, you need to write the 0 for marking the end of those and then write to the file the totals values. So, as an example, the alphaFlowers_db.txt file should look like the following: 83453 Begonia Pink Jumbo 4.19 16.99 4 83454 Begonia White Jumbo 4.19 16.99 3 17200 Bluebell Blue Small 0.89 2.89 16 67801 Daffodil Yellow Medium 1.59 4.69 12 67805 Daffodil White Small 1.89 4.99 17 67121 Dahlia Yellow Medium 1.89 3.99 10 ..... and more flower records... 24 48 0 559 3093.67 1213.47 0.00 0.00 0.08 4. Once the database has been successfully sorted, you can now set up the command menu and have it execute commands that do something with the database; however, you are only required to write three commands that execute. You program will present the user with the following menu: Select a menu item: 1) Delete Flower 2) Add Flower 3) Sell Flower 4) Buy New Flower 5) Buy More of Existing Flower 6) Look Up Flower *) Quit with a goodbye message You are to get this menu running within a loop that will continue to run, asking for the command, until the user types a 'O' to terminate the program. You are only required to implement 1), 2), and 6). For the rest all you need to do is output a message, for example: " - Buy New Flower" outputOneFlower() will be the simplest of the three. You simply get the flowerID as input from the Console and then print out to the Console the contents of the flower record for the flower that matches that flower1D number. If you type in a non-existing flower number, then output an error message and transfer control back to the command menu. Once the user chooses to quit the program by typing '0, then update alphaFlower_db.txt to reflect whatever changes you have made in the database content. This will include updating the totals values as well. Demonstrate the action of the three commands, by outputting the updated database contents to the Console and submitting the latest alphaFlower_db.txt file. INCLUDE THAT FILE ALSO IN THE final ZIP directory