Question
You will write a two-class Java program that implements the Game of 21. This is a fairly simple game where a player plays against a
You will write a two-class Java program that implements the Game of 21. This is a fairly simple game where a player plays against a dealer. The player will receive two and optionally three numbers. Each number is randomly generated in the range 1 to 11 inclusive (in notation: [1,11]). The players score is the sum of these numbers. The dealer will receive two random numbers, also in [1,11]. The player wins if its score is greater than the dealers score and is also less than or equal to 21. A tie goes to the dealer. The player may wager an amount of money before receiving each number. If the player wins, the wager is added to the players total money. If the player loses, the wager is subtracted from the players total money. The game is played as follows: The player starts with a total amount of money, 100.00 in this case. The dealer asks for an initial bet from the player. This is called an ante. The amount of money the player can bet has to be less than or equal to the players total amount of money. Then, the dealer generates a random number for the player. That is added to the players score, which is initially zero. The dealer then generates a random number for itself and adds that to the dealers score. The player may place a bet with the same betting conditions as mentioned above. Then, the dealer generates another number for the player and for itself. Another bet may be placed by the player. The dealer then asks if the player wants one more number. If yes, the dealer generates another random number for the player. Finally, the dealer checks if the player has won. If so, the players total money is the sum of the players initial money and the total bets the player has made. If the player has lost, the players total money is the initial money minus the total amount the player has bet. The Program Structure. The program consists of two Java class definitions: Dealer21 and Driver21. The specification for these class definitions is as follows. Dealer21.java This class simulates the dealer. The dealer manages the state of the game, including the players money, bets, and the score for both player and dealer, and generates random numbers. The dealer also determines the winner of the game. Member variable declarations (name, data type, purpose): dealerScore, integer, the sum of the numbers generated for the dealer. playerScore, integer, the sum of the numbers generated for the player. playerBet, double, the sum of the bets placed by the player. playerMoney, double, the players initial amount of money. rand, Random, generates random numbers for the game. Methods Constructors: There are two constructors. One takes no parameters, and one takes a single parameter- an integer (could also be a long integer), which will serve as the seed for the instance of Random. Both constructors call the init method (see below), which initializes the first four member variables listed above. In addition to calling init, the first constructor creates an instance of Random using its no-parameter constructor, while the second constructor passes the seed value to the Random constructor (refer to the java.util.Random class in the Java API if necessary). Private methods: init: no parameters, no return value. Initializes dealer and player scores to 0, the player bet to 0.0, and the player money to 100.00. generateNum: no parameters, returns an integer, which is a (uniform) random number in the range 1 to 11, inclusive. Public methods: getPlayerBet: no parameters, returns a double, the players aggregate bet. getPlayerMoney: no parameters, returns a double, the players money. getPlayerScore: no parameters, returns an integer, the players aggregate score. getDealerScore: no parameters, returns an integer, the dealers aggregate score. playerWins: no parameters, returns Boolean: true if the player wins, false otherwise. The player wins if the dealer's score is not 21 and the player's score is less than or equal to 21 and the player's score is greater than the dealer's score. placeBet: takes a double parameter- the amount of the players bet, returns boolean. Checks if the amount the player has bet so far plus the bet passed in is less than or equal to the player's total money. If this is true, it adds the amount passed in to the player's current bet and returns true, otherwise, the bet passed in is not added and false is returned. generatePlayerNumber: no parameters, returns an integer, a number in [1,11]. Calls generateNum, adds this number to the player's score, and returns the number generated. generateDealerNumber no parameters, returns an integer, a number in [1,11]. Calls generateNum, adds this number to the dealer's score, and returns the number generated. Driver21.java This class handles console interactions with the player (user). It creates an instance of Dealer21 and uses its public methods (API) to play the game. Member variables: none. Methods: main. An instance of Dealer21 is created in main. Its methods are called to play the game. Actually, you will write two statements to create the Dealer21 instance: one will call the no-parameter constructor, and one will call the constructor that takes a seed value as a parameter. Comment out the first statement. Use the seed version during development and testing. The seed you use will determine the numbers generated during the game. The rest of the code in the main method must produce the same output as in the following examples. Use the strings in the example in your code. Use a single java.util.Scanner instance to take the users input. Use the nextInt and next Scanner class methods. Example 1:
You must reproduce this output exactly. Of course, the numbers will change for each run. None of the numbers that appear in the example above should be hard-coded in the Driver21 code. For example, this is a statement where the number 100 is hard-coded: System.out.println("You have 100.00 money to bet with."); This number should be obtained from the Dealer object. This is the correct way to write the statement: System.out.println("You have "+dealer.getPlayerMoney()+" money to bet with."); All numbers seen in the output above should be obtained from the Dealer object. Another example follows, where the player does not want a third number (answers N):
Notice that the same random numbers were generated in both examples. This is because the same seed, 1234567, was used. You may use any integer for a seed number. What happens if a player bet is higher than the players resources? The player bet is a running sum of all bets the player has made. The next bet a player makes has to be less than or equal to the difference of the player bet and the player total money. If the bet is too high, the placeBet method returns false. The bet will not be accepted. Since we are not using loops in this project, the game moves on. The following example shows what the program does in the case of a bet that was too high.
There are many refinements that could be made to this program. As with all software, new ideas come to mind once you observe the program in action. This is why good coding practices are so important: software is malleable and is constantly changing. Good design and good coding make modification much easier to achieve.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
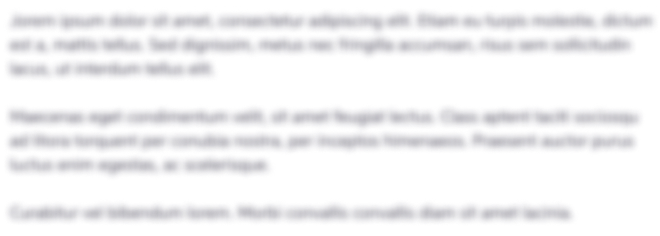
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started