Question
Your first job is to write a Phone class, where we will be using the Comparable interface. Before you start writing the compareTo method, be
Your first job is to write a Phone class, where we will be using the Comparable interface. Before you start writing the compareTo method, be sure to have the class with:
- 3 instance variables (at least)
- Getters and setters for each
- 2 additional methods of your choice
Comparable
This leads to another question, how do I compare objects in such a way that I will know which one should go first and which should go second? In comes the Comparable interface.
First, implement the comparable interface like so:
public class Phone implements Comparable
Weve used interfaces before so everything up to Comparable should look a little familiar. What is this
The interface Comparable authorizes an int-returning method compareTo, which takes a Phone as its parameter. Define and implement the compareTo method.
This method should return one of three possible values:
- -1 (or any negative int) if this object is "less than" or "comes before" the parameter object
- 0 if this object has the same value as the parameter object
- +1 (or any positive int) if this object is "greater than" or "comes after" the parameter object
Part 3 (5 points)
- You should first confirm that other is not null, and if not, throw an IllegalArgumentException.
(If we had not used the type parameter
- Then, check whether this and the parameter are literally the same object in memory, in which case we should return 0.
- Then, go through each of the instance variables in an order that you decide. For each one compare the instance variable values of this and other; if they are different, return -1 or 1 as appropriate. If they are the same, move on to the next instance variable. If all of the instance variables are the same, we should return 0. Note that you'll be relying somewhat on String's compareTo method here!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
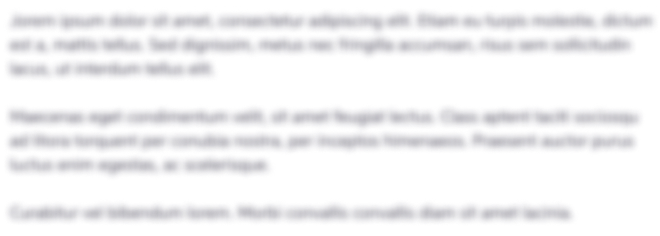
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started