Question
Your objective is to implement the list abstract data type using both an array and a linked list implementation. A template has been provided for
Your objective is to implement the list abstract data type using both an array and a linked list implementation. A template has been provided for you. Rename the template to Main.java. To be clear, both the array and linked list classes must implement all methods in the interface provided by the template. Just like in Python, any abstract methods must have a body by the class inheriting from it. For an interface, all methods are abstract and therefore all must be given a body
PLEASE PLEASE PLEASE Dont use the solution already posted for this question
DONT USE THIS SOLUTION BELOW!!!
START OF SOLUTION
/** The interface for our List (Abstract Data Type) */
interface IList {
/** Adds the given value to the end of the list */
void append(char value);
/** Adds the given value to the beginning of the list */
void prepend(char value);
/** Deletes the container at the given position (a container holds a value) */
void deleteAt(int position);
/** Returns the number of values currently in our list */
int size();
/** Retrieves the value at the given position (0-based) */
char getValueAt(int position);
/** Searches for the FIRST occurence of a given value in our list.
* If found, it returns the position of that value.
* If not found, it returns -1 */
int positionOf(char value);
}
/** Array implementation of our List */
class ListAsArray implements IList {
// initialize array to a size of 30 elements
// this will prevent the need to resize our array
private char[] array = new char[30];
private int size = 0;
@Override
public void append(char value) {
if (size == array.length) {
// resize array
char[] newArray = new char[array.length * 2];
System.arraycopy(array, 0, newArray, 0, array.length);
array = newArray;
}
array[size] = value;
size++;
}
@Override
public void prepend(char value) {
if (size == array.length) {
// resize array
char[] newArray = new char[array.length * 2];
System.arraycopy(array, 0, newArray, 1, array.length);
array = newArray;
} else {
// shift elements over to the right
for (int i = size - 1; i >= 0; i--) {
array[i + 1] = array[i];
}
}
array[0] = value;
size++;
}
@Override
public void deleteAt(int position) {
if (position < 0 || position >= size) {
throw new IndexOutOfBoundsException();
}
// shift elements over to the left
for (int i = position; i < size - 1; i++) {
array[i] = array[i + 1];
}
size--;
}
@Override
public int size() {
return size;
}
@Override
public char getValueAt(int position) {
if (position < 0 || position >= size) {
throw new IndexOutOfBoundsException();
}
return array[position];
}
@Override
public int positionOf(char value) {
for (int i = 0; i < size; i++) {
if (array[i] == value) {
return i;
}
}
return -1;
END OF SOLUTION
Also could you put comments on some of the data types to explain them, thank you so much
Heres the template:
/** The interface for our List (Abstract Data Type) */ interface IList { /** Adds the given value to the end of the list */ void append(char value); /** Adds the given value to the beginning of the list */ void prepend(char value); /** Deletes the container at the given position (a container holds a value) */ void deleteAt(int position); /** Returns the number of values currently in our list */ int size();
/** Retrieves the value at the given position (0-based) */ char getValueAt(int position);
/** Searches for the FIRST occurence of a given value in our list. * If found, it returns the position of that value. * If not found, it returns -1 */ int positionOf(char value); }
/** Array implementation of our List */ class ListAsArray implements IList { // initialize array to a size of 30 elements // this will prevent the need to resize our array }
/** Singly Linked List implementation of our List */ class ListAsLinkedList implements IList { }
/** A singly linked list node for our singly linked list */ class Node { }
/** contains our entry point */ public class Main { /** entry point - DO NOT CHANGE the pre-existing code below */ public static void main(String[] args) { int[] numbers = {105,116,112,115,65,58,47,47,116,105,110,121,88,117,114,108,46,99,111,109,47}; int[] numbers2 = {97,59,111,53,33,111,106,42,50}; int[] numbers3 = {116,104,32,111,116,32,111,71}; /// List as an Array IList array = new ListAsArray(); // add values for(int num : numbers) { array.append((char)num); } for(int num : numbers3) { array.prepend((char)num); } // delete some values int position; position = array.positionOf((char)105); array.deleteAt(position); position = array.positionOf((char)65); array.deleteAt(position); position = array.positionOf((char)88); array.deleteAt(position); // print em position = 0; while (position < array.size()) { System.out.print(array.getValueAt(position)); position++; } /// List as a Linked List IList linkedList = new ListAsLinkedList(); // add values for(int num : numbers2) { linkedList.append((char)num); } linkedList.prepend((char)55); linkedList.prepend((char)121);
// delete some values position = linkedList.positionOf((char)59); linkedList.deleteAt(position); position = linkedList.positionOf((char)33); linkedList.deleteAt(position); position = linkedList.positionOf((char)42); linkedList.deleteAt(position); // print em position = 0; while (position < linkedList.size()) { System.out.print(linkedList.getValueAt(position)); position++; } System.out.println(); // ??? } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
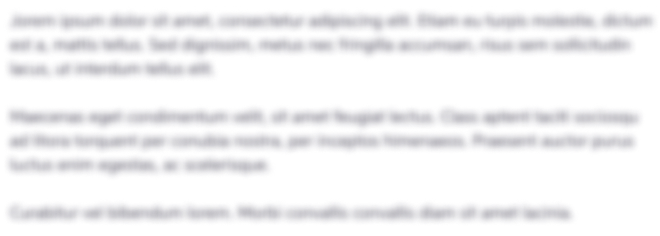
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started