Question
Your program must be capable of utilizing a command line argument to specify the input log file and output file with the filtered data and
Your program must be capable of utilizing a command line argument to specify the input log file and output file with the filtered data and analysis results.
./project1 inputFile outputFile
Your program must ensure the user has correctly provided the required command-line arguments and display a usage statement if the provided arguments are incorrect.
Requirements Summary
Create a program that will produce a smoothed curve of timestamped distance data, with annotations to denote significant changes in the angle of detection, or distance to detected object. The resulting timestamped data (with annotations) should be placed in the outputFile.
Data description and motivation
The data in the inputFile are simplified information from a front-mounted LIDAR sensor on board an autonomous vehicle. All data presented in test files are collected from the CAT Vehicle Testbed at the University of Arizona.
Data are collected at a frequency of 75 Hz, and each datapoint consists of 181 samples of distance information that scan an arc of pi radians, with time information that indicates the computer time at which the computer recorded the data from the sensor. Each pulse of the laser returns a distance (in meters) of any reflection it encounters; if no reflection is detected, then the maximum range of the sensor is returned---81m.
A separate software component has already analyzed this hemispherical scan, and found the smallest distance value for each sample, and its index in the vector of length 181. Through the index number, the angle (in radians) of the nearest sample is returned.
Through the use of this distance, angle, and timing information, the distance between the CAT Vehicle and its nearest obstacle can be inferred; differentiating this data provides velocity and acceleration information---but unfortunately the noise in the data prevents the direct application of a differential operator. Thus, the goal of this project is to smooth the data, so that it can be more suitable for estimating relative velocity.
Input file format
The input log consists of a timestamp, followed by distance and then angle information.
TTTTT.TTT,DD.DD,A.AAA
Each line is separated by a newline. If a line does not match this format, it should be ignored and the program should continue processing the file.
The following is a simple example of the inputFile format:
1464815342.110273122787, 17.889999389648, 0.095994234085 1464815342.123246669769, 17.920000076294, 0.087267547846 1464815342.137223720551, 17.889999389648, 0.095994234085 1464815342.150209188461, 17.920000076294, 0.087267547846 1464815342.163207292557, 17.909999847412, 0.095994234085 1464815342.177282094955, 17.920000076294, 0.087267547846 1464815342.189191341400, 17.909999847412, 0.095994234085 1464815342.204182624817, 17.909999847412, 0.087267547846 1464815342.217026233673, 17.899999618530, 0.095994234085 1464815342.230037212372, 17.920000076294, 0.087267547846 1464815342.243027210236, 17.909999847412, 0.095994234085
The input data times may have minor jitter, i.e., on average the frequency is 75 Hz, but is not guaranteed to always be 1/75 seconds apart. You should ensure that your program will not crash at runtime, for example due to issues such as opening an empty file, or reading in an invalid file format.
The maximum distance is 81.0m, and the default angle if no obstacle is found is -pi/2 rad (i.e., less than -1.570).
Data structures
The following data structure and enumerated type should be used for storing and filtering data.
// This enumerated type provides entries for each of the // potential labels attached to a sample typedef enum FilterStatus { UNDEFINED=-1, VALID, FILTERED, ANGLE_RESET, DISTANCE_RESET } FilterStatus; // This struct is used to print out the filtered data // (and can also be used to store data from the read files) // N.B. a previous version of this struct used float rather than // double values: if you had trouble with truncating data points, // change ALL of your variables that used to be float to be double typedef struct obstacleDataSample_struct { double timestamp; double distance; double angle; FilterStatus status; } obstacleDataSample;
You may define other structures and types as you see fit.
Filter algorithm
The only data value which is being filtered is the distance; the filter is an average of all distance points across the width of the filter, which is defined at compile time as 11 data points. The filtered value is the center of this filtering window. For example, consider the subset of values below:
0,1.03,0.05840 0.01333,1.01,0.05840 0.02667,1.1,0.05840 0.04,1.04,0.05840 0.05333,1,0.05840 0.06667,0.979,0.05840 0.08,0.958,0.05840 0.09333,0.937,0.05840 0.10667,0.916,0.05840 0.12,0.895,0.05840 0.13333,0.874,0.05840 0.14667,0.824,0.05840
For the above example, the average distance 0.957545 m would be valid for time 0.08. An example result (in the appropriate file format) if these were the only datapoints in the file would be:
0.000000,1.030000,0.058400,UNDEFINED 0.013330,1.010000,0.058400,VALID 0.026670,1.100000,0.058400,VALID 0.040000,1.040000,0.058400,VALID 0.053330,1.000000,0.058400,VALID 0.066670,0.979000,0.058400,VALID 0.080000,0.957545,0.058400,FILTERED 0.093330,0.937000,0.058400,VALID 0.106670,0.916000,0.058400,VALID 0.120000,0.895000,0.058400,VALID 0.133330,0.874000,0.058400,VALID 0.146670,0.824000,0.058400,VALID
The filter should be run only when the full filter window of valid points is available; otherwise, the existing distance is used. Angle data are not filtered, but are used to determine validity.
A sample is determined to be valid when compared to the previous sample: the relative distance must be strictly less than the specified tolerance of 0.1 m, and the relative angle must be strictly less than 15 degrees. Consider using the M_PI constant, defined in the cmath library to perform this calculation. The below should help in understanding how to classify each point, in order to determine whether to filter.
- The first element is always UNDEFINED
- If the sample has the default angle, the sample is labeled UNDEFINED
- If the sample can be used in filtering, but does not represented filtered data, it is labeled VALID
- If the angle between two points exceeds the angle maximum tolerance, the sample is labeled ANGLE RESET.
- If it is within the angular tolerance but the distance between two points exceeds the distance maximum tolerance, then the second point is given DISTANCE RESET.
- If a sample is not within either distance or angle tolerance of a previous sample, and is not at the maximum distance or default angle, it is given ANGLE RESET.
Output file format
The output file should be in the same format as the input file, of lines of text each of which is followed by a new line. There should be one addional entry per line that indicates the status of each data point. These are represented as data that may be used in filtering (VALID), filtered valid data with (FILTERED), marks a new position due to a significant change in angle (ANGLE RESET), a significant step in distance (DISTANCE RESET), or that the sample is undefined (UNDEFINED), as text outputs.
TTTTT.TTTTTT,DD.DDDDDD,A.AAAAAA,STATUS
All numerical values should be printed with setprecision(6) of a C++ stream.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
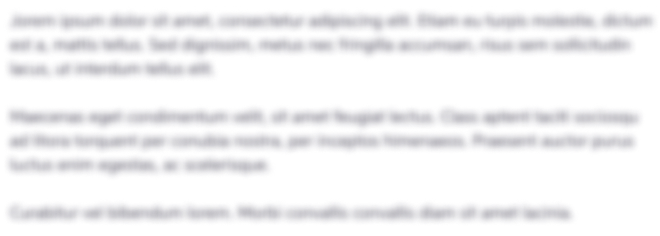
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started