Question
Your project must meet these specific requirements: Use at least 2 tables in a multi-table SQLite database. Take an input from the user (use the
Your project must meet these specific requirements:
Use at least 2 tables in a multi-table SQLite database.
Take an input from the user (use the Scanner class) and use the input to build and run a query (use PreparedStatement) against the database. The input must be validated/sanitized appropriately, preventing SQL injection.
Print output that includes data read from the database. Output should include information from at least 2 tables and be in a user-friendly form.
The presentation and data layers are separate: there is no user interface input/output code in the data layer and no database code in the presentation layer. Thus, you will need at least 2 classes in the project (one for data, one for presentation), but you are welcome to have more than 2.
All classes and all methods other than 1-line getters and setters are documented with javadoc comments.
There is a main method that starts the program.
I have tried various fixes but I have gone back to the basic code. All I have left is to use user input to read from sql..lets say CA for california, So user inputs CA and then only customers from CA come up with their information and email showing.
This is what I have so far:
MAIN CLASS
package edu.pcc.cis233j.jdbctutorial; import java.util.Scanner; import java.util.List; /** * Print info on customers in the FiredUp database */ public class Main { public static void main(String[] args) {
System.out.println("Enter State or Province name:"); Scanner sc = new Scanner(System.in);
String stateName = sc.nextLine(); System.out.println("FiredUp Customers:"); FiredUpDB firedUp = new FiredUpDB(); Listcustomers = firedUp.readCustomers(); for (Customer cust : customers) { System.out.println("ID: " + cust.getId() + ", Name: " + cust.getName() + ", City: " + cust.getCity() + ", State: " + cust.getState() + ", Email Address(es): " + cust.getEmailAddresses()); } } }
FIREDUPDB CLASS
package edu.pcc.cis233j.jdbctutorial; import java.sql.*; import java.util.ArrayList; import java.util.List; /** * Data access class for the FiredUp database. * Currently reads only customer information. * */ public class FiredUpDB { private static final String FIREDUP_DB = "jdbc:sqlite:FiredUp.db"; //private static final String FIREDUP_URL = "jdbc:jtds:sqlserver://cisdbss.pcc.edu/FiredUp"; //private static final String USERNAME = "275student"; //private static final String PASSWORD = "275student"; private static final String CUSTOMER_SQL = "SELECT CustomerID, Name, City, StateProvince FROM CUSTOMER where StateProvince = stateName"; //private static final String CUSTOMER_SQL = "SELECT CustomerID, Name, City, StateProvince FROM CUSTOMER"; private static final String EMAIL_SQL = "SELECT EmailAddress FROM EMAIL WHERE FK_CustomerID = ?"; /** * Read all customers from the FiredUp database and return them as a list of Customer objects * @return a list of customers from the FiredUp database */ public ListreadCustomers() { ArrayList customers = readCustomerBasics(); readEmailAddresses(customers); return customers; } /** * Read from the FiredUp database customers from the given state * @param state the state of interest (US state or Canadian province) * @return a list of customers from the given state */ // Create method readCustomersFromState private ArrayList readCustomerBasics() { ArrayList customers = new ArrayList<>(); try ( Connection conn = getConnection(); PreparedStatement stmt = conn.prepareStatement(CUSTOMER_SQL); ResultSet rs = stmt.executeQuery() ) { while (rs.next()) { customers.add(new Customer(rs.getInt("CustomerID"), rs.getString("Name"), rs.getString("City"), rs.getString("StateProvince"))); } } catch (SQLException e) { e.printStackTrace(); } return customers; } /** * @return a connection to the FiredUp database * @throws SQLException if unable to connect */ private Connection getConnection() throws SQLException { return DriverManager.getConnection(FIREDUP_DB); //return DriverManager.getConnection(FIREDUP_URL, USERNAME, PASSWORD); } /** * Read customers from the database, including their basic properties from the CUSTOMER table, * but not including customer data from related tables such as email addresses or phone numbers * @return a list of Customer objects */ /* private ArrayList readCustomerBasics() { ArrayList customers = new ArrayList<>(); try ( Connection conn = getConnection(); PreparedStatement stmt = conn.prepareStatement(CUSTOMER_SQL); ResultSet rs = stmt.executeQuery() ) { while (rs.next()) { customers.add(new Customer(rs.getInt("CustomerID"), rs.getString("Name"), rs.getString("City"), rs.getString("StateProvince"))); } } catch (SQLException e) { e.printStackTrace(); } return customers; }*/ /** * Read email addresses from the database for each customer in the given list, * adding the email addresses found to the corresponding Customer object * @param customers list of customers whose email addresses should be read */ private void readEmailAddresses(ArrayList customers) { try ( Connection conn = getConnection(); PreparedStatement stmt = conn.prepareStatement(EMAIL_SQL) ) { for (Customer cust : customers) { stmt.setInt(1, cust.getId()); ResultSet rs = stmt.executeQuery(); while (rs.next()) { cust.addEmailAddress(rs.getString("EmailAddress")); } } } catch (SQLException e) { e.printStackTrace(); } } }
CUSTOMER CLASS
package edu.pcc.cis233j.jdbctutorial; import java.util.ArrayList; /** * A customer in the FiredUp database (not all properties are included) */ public class Customer { private int id; private String name; private String city; private String state; private ArrayListemailAddresses; public Customer(int id, String name, String city, String state) { this.id = id; this.name = name; this.city = city; this.state = state; emailAddresses = new ArrayList<>(); } public int getId() { return id; } public String getName() { return name; } public String getCity() { return city; } public String getState() { return state; } /** * Add an email address to this customer's email addresses * @param email an email address belonging to this customer */ public void addEmailAddress(String email) { emailAddresses.add(email); } /** * @return the list of email addresses belonging to this customer */ public ArrayList getEmailAddresses() { return emailAddresses; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
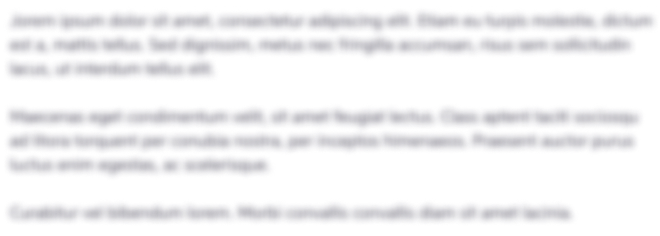
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started