Question
Your task for Project 1 is to create a language that will allow a user to manage, store, and query user data for a corporation.
Your task for Project 1 is to create a language that will allow a user to manage, store, and query user data for a corporation. You will be creating a system similar to SQL. This project will focus on creating the basic language parsing mechanism to recognize valid (and invalid) phrases in the language. Below you will find a complete sampling of the valid statements in the language. Note that some of the statements can be nested together. You should only print the following:
You must implement functionality for both the execute command as well as the exit command.
If it is a valid statement is should print VALID select Statement (where select is whatever the type is)
If it is not a valid statement it should print INVALID select Syntax (where select is whatever the type is)
If it doesnt have a type (complete garbage) then print INVALID SYNTAX
CLARIFICATIONS:
TABLE_NAME must be alphabetic only (no reserved words and no special symbols)
COLUMN_NAME must be alphabetic only (no reserved words and no special symbols)
Commands can be multi-lined as well as multiple statements on a single line
(Underline denotes optional items)
Functionality:
create table TABLE_NAME (
COLUMN_NAME TYPE,
COLUMN_NAME TYPE,
COLUMN_NAME TYPE,
....
);
Note: TYPE is the type of the data stored in the field of the table. The valid types are: integer, float, boolean, char, char(n) where n is an integer (fixed length string).
drop table TABLE_NAME;
alter table TABLE_NAME add COLUMN_NAME TYPE;
alter table TABLE_NAME drop COLUMN_NAME;
alter table TABLE_NAME alter COLUMN_NAME TYPE;
select distinct COLUMN_NAME_LIST from TABLE_EXPRESSION where BOOLEAN_EXPRESSION;
NOTE: COLUMN_NAME_LIST can be the following:
COLUMN_NAME, COLUMN_NAME, ...
* denotes ALL columns. EX. select * from Student;
NOTE: TABLE_EXPRESSION can be the following:
TABLE_NAME
A nested SELECT_STATEMENT in parenthesis.
TABLE_EXPRESSION natural join TABLE_EXPRESSION
NOTE: BOOLEAN_EXPRESSION can contain the following:
COLUMN_NAME COMPARATOR IDENTIFIER
IDENTIFIER is a VALUE
COMPARATOR is one of the following: =, <, >, >=, <=.
BOOLEAN_EXPRESSION LOGIC_OPERATOR BOOLEAN_EXPRESSION
LOGIC_OPERATOR is either AND or OR.
AND has higher precedence (i.e. it gets evaluated first)
not BOOLEAN_EXPRESSION
insert into TABLE_NAME (COLUMN_NAME_LIST) values (VALUES_LIST);
NOTE: COLUMN_NAME_LIST length must match VALUES_LIST length, but Type doesnt need to be checked for this project.
update TABLE_NAME set COLUMN_NAME = VALUE where BOOLEAN_EXPRESSION;
delete from TABLE_NAME where BOOLEAN_EXPRESSION;
execute from FILE_NAME;
exit;
STARTER CODE:
package identification;
import java.io.*;
import java.util.Scanner;
public class Main
{
public static void main( String args[] )
{
Scanner input = new Scanner(System.in);
Command comm = null;
String information = "";
while(!(comm instanceof ExitCommand))
{
System.out.print(">");
information = input.nextLine();
if(information.contains(";"))
{
String command = information.substring(0,information.indexOf(";"));
comm = Parser.parse(command);
comm.execute();
information = information.substring(information.indexOf(";")+1)
}
}
}
}
CREATETABLE CLASS
package identification;
import java.sql.*;
public class CreateTable implements Command
{
private String tableName;
private String[] columnNameType;
public CreateTable(String input)
{
//parse the string to see if it is valid.
}
public void execute()
{
System.out.println("Valid Create Syntax.");
}
}
COMMAND CLASS
package identification;
public interface Command {
public void execute();
}
EXIT COMMAND
package identification;
public class ExitCommand implements Command {
public void execute()
{
System.out.println("System exiting.");
}
}
CLASS PARSER
package identification;
public class Parser
{
public static Command parse(String userInput)
{
Command result;
if(userInput.equals("exit"))
result = new ExitCommand();
else if(userInput.startsWith("create table"))
result = new CreateTable(userInput);
return result;
}
}
CAN YOU PLEASE FINISH THE CODE FOR THE CLASSES; DELETE, INSERT,UPDATE,DROP TABLE AND SELECT. AND FINISH UP ON THE STARTER CODE, IF THERE IS ANYTHING MISSING.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
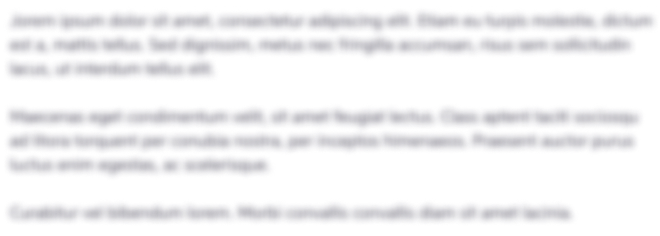
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started