Question
Your task in this homework assignment is to add accessor and mutator functions to a Pizza class. Once you have those functions in place, you
Your task in this homework assignment is to add accessor and mutator functions to a Pizza class.
Once you have those functions in place, you will need to write a price computer function for a Pizza.
## Solution Specifications
Your solution to this problem must meet the following criteria.
1. Write three accessors, one for each private variable in the Pizza class. Name the variables based on the common practice discussed in class.
2. Write three mutators, one for each private variable in the Pizza class. Name the variables based on the common practice discussed in class.
3. After finishing the accesor and mutator the functions, add a `computePrice` functions. The price of a pizza is determined by the following rules:
* Small = $10
* Medium = $14
* Large = $17
* Each topping is $2
Using C++ language:
In main.cpp file:
#include "Pizza.h"
#include
using namespace std;
int main() {
// Variable declarations
Pizza cheesy;
Pizza veggie;
Pizza custom;
cheesy.setToppings(1);
cheesy.setType(HANDTOSSED);
cheesy.outputDescription();
cout << "Price of cheesy: $" << cheesy.computePrice() << endl;
veggie.setSize(LARGE);
veggie.setToppings(5);
veggie.setType(PAN);
veggie.outputDescription();
cout << "Price of veggie : $" << veggie.computePrice() << endl;
veggie.setSize(MEDIUM);
veggie.setToppings(3);
veggie.setType(DEEPDISH);
veggie.outputDescription();
cout << "Price of custom : $" << veggie.computePrice() << endl;
cout << endl;
}
in pizza.cpp file (edit):
#include "Pizza.h"
#include
using namespace std;
//========================
// Pizza
// The constructor sets the default pizza
// to a small, deep dish, with only cheese.
//========================
Pizza::Pizza() {
size = SMALL;
type = DEEPDISH;
toppings = 1;
}
//==================================
// Accessors and Mutators Follow
//==================================
// TODO Add the accessor and mutator functions for each class variable.
// TODO Add a function to compute price of the pizza
//==================================
// outputDescription
// Prints a textual description of the contents of the pizza.
//==================================
void Pizza::outputDescription() {
cout << "This pizza is: ";
switch (size) {
case SMALL:
cout << "Small, ";
break;
case MEDIUM:
cout << "Medium, ";
break;
case LARGE:
cout << "Large, ";
break;
default:
cout << "Unknown size, ";
}
switch (type) {
case DEEPDISH:
cout << "Deep dish, ";
break;
case HANDTOSSED:
cout << "Hand tossed, ";
break;
case PAN:
cout << "Pan, ";
break;
default:
cout << "Uknown type, ";
}
cout << "with " << toppings << " topping(s)" << endl;
}
In pizza.h file (edit):
const int SMALL = 0;
const int MEDIUM = 1;
const int LARGE = 2;
const int DEEPDISH = 0;
const int HANDTOSSED = 1;
const int PAN = 2;
class Pizza {
public:
Pizza();
~Pizza(){};
// TODO Add the accessor and mutator functions for each class variable.
//==================================
// computePrice
// Returns:
// Price of a pizza using the formula:
// Small = $10 + $2 per topping
// Medium = $14 + $2 per topping
// Large = $17 + $2 per topping
//==================================
double computePrice();
void outputDescription();
private:
int size, type, toppings;
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
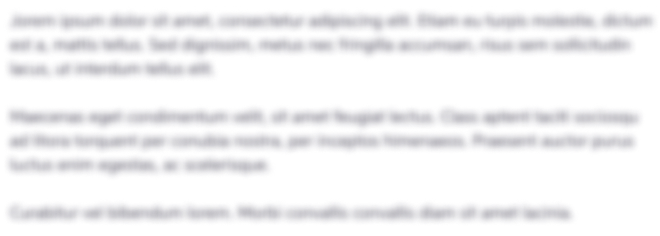
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started