Question
Your task in this programming assignment is to design a python3 class that represents a Complex Number as defined in the field of Mathematics. The
Your task in this programming assignment is to design a python3 class that represents a Complex Number as defined in the field of Mathematics.
The specifications of your Complex number class include:
1. It should be called Complex
2. Its constructor takes up to two arguments for the real and imaginary portions of the complex number. This constructor uses default values of 0 for both the real and imaginary parts if they are not provided.
3. The class contains two instance variables to represent the real and imaginary parts of a complex number
4. The class should contain accessors and mutators for its instance variables. These accessors and mutators should utilize the decorator method as discussed in class.
5. The class also overloads some mathematical/logical operators i.e. defines what these operators mean in the context of Complex numbers. The overloaded operators include: Addition (__add__). Takes another complex number as an argument, and returns the sum of that complex number and itself as a completely different complex number object.
Subtraction (__sub__). Takes another complex number as an argument and returns the difference between itself and that argument as a completely different complex number object.
Multiplication (__mul__). Takes another complex number as an argument, and returns the product of itself and the argument as a completely different complex number object.
Division(__truediv__). Takes another complex number as an argument and returns the quotient of itself and the argument as a completely different complex number object.
Equality(__eq__). Takes another complex number as an argument and returns a boolean representing whether it is equal to that argument or not.
6. The class should also contain a reciprocal function that returns the reciprocal of itself as a completely different complex number object. 7. The class should also contain a conjugate function that returns the conjugate of itself as a completely different complex number object. 8. The class should contain a __str__ function that determines how the complex number will be represented when printed. This is typically in the form a + bi where a is the real part, and b is the imaginary part of the complex number. However, if the imaginary part is negative, then it takes the form of a bi. 9. Feel free to add any other functions that you feel will help to make your class more efficient and/ or neater. You are provided with a test file (ComplexTest.py) that when executed in the same folder as a Complex.py file (that contains your class definition) will test the functionality defined above that your class should have. The test file checks Object creation, the mathematical and logical operators, as well as the conjugate and reciprocal functions. Note that you should not make any changes to the test file and if you have any errors, they can be resolved by making edits to your Complex.py file. To execute the entire program, simply run the ComplexTest.py file. If your class is properly defined in a Complex.py file that is in the same folder, then it should automatically use it to produce the expected output shown below.
1 a = 4 + 5i
b = 2 + 6i
c = 4 - 3i
d = 0 + 0i
e = -3 + 0i
--------------------------------------------------
1/(2 + 6i) = 0.05 - 0.15i
1/(4 - 3i) = 0.16 + 0.12i
4 + 5i == 2 + 6i: False
4 - 3i == 4 - 3i: True
(4 - 3i)* = 4 + 3i
(4 + 5i)* = 4 - 5i
--------------------------------------------------
(4 + 5i) + (2 + 6i) = 6 + 11i
(4 + 5i) + (4 - 3i) = 8 + 2i
(4 + 5i) - (2 + 6i) = 2 - 1i
(4 - 3i) - (4 + 5i) = 0 - 8i
(4 + 5i) * (2 + 6i) = -22 + 34i
(2 + 6i) * (4 - 3i) = 26 + 18i
(4 + 5i) / (2 + 6i) = 0.95 - 0.35i
(4 - 3i) / (2 + 6i) = -0.24999999999999994 - 0.75i
==================================================
A few extra constraints 1. You must include a meaningful header, use good coding style, use meaningful variable names, and comment your source code where appropriate;
2. Your output should be exactly like the sample run shown above;
3. Make your code as efficient as possible i.e. make sure that there arent multiple functions/sections of code doing the same/similar thing
4. You must submit just your class file (Complex.py) as a single .py file.
5. You are also provided with a file that contains information on the mathematical operations that can be applied to complex numbers. If you are unfamiliar with complex numbers or need a refresher, feel free to look over that document
Step by Step Solution
There are 3 Steps involved in it
Step: 1
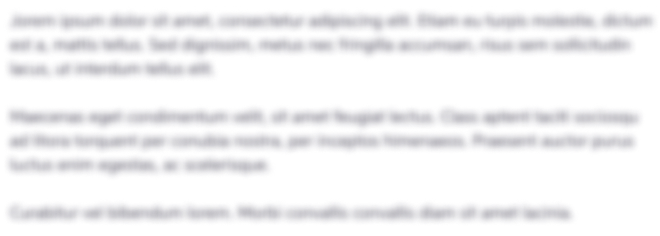
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started