Question
Your task is to create software for an occupation list object that uses a linked list instead of an array, then substitute this new class
Your task is to create software for an occupation list object that uses a linked list instead of an array, then substitute this new class for the array-based occupation list class in a copy of the Occupation List assignment you have been working on. You will also need to add a list node class to the project. You should start by making a copy of the first week's IntelliJ Occupation List project, then work with that copy. You do not need to work with the project that involves menus, just the original Occupation List project. If you do things correctly, the new Occupation List class will have properties and methods with names matching those in the array-based Occupation List class, so you should be able to substitute the linked list class into the project in place of the array-based class without changing the main executable class or the Occupation class. You will need to create a ListNode class in addition to the new Occupation List class. Chapter 24 discusses this. Chapter 24 ends with a section discussing how to build a new linked list from a data file containing sets of data for properties in objects of a data class. That is exactly what your software will need to do. Previously, your software had a method to build an array of Occupation objects from a data file. Now it will have a method to build a linked list of Occupation objects from a data file. This section in the chapter is a guide for how to do this. So, you will need to create two classes a class for a list node and a class for the list itself. I strongly suggest you begin with an annotated UML diagram for each class showing the properties and methods needed for the class. The UML diagram for a list node class is in the chapter. The list class itself is simple, but make sure it includes methods similar to those in your existing project to load the list from a data file, print the data, and search the data. Once you have an annotated UML diagram for each of the two new classes, then use the documentation-first approach described starting on page 26 of the Chapter 2 from CSCI 111 (included with this week's material) to outline what each method needs to do, then write the code to do what the outline says to do. This will help you to focus first on the logic of the algorithms, and then focus on coding the logic in Java. If you have any questions about how to do this or run into trouble, then please let me know and I can help you. If your question is about software you are working on, then send a copy of the project with your Canvas message. You should submit a copy of the finished project with the software in the project well-documented. Later we will have a quiz, which will ask you about linked lists and some of the differences between using arrays and linked lists in software such as this.
***MY CODE SO FAR***
***PLEASE HELP I'M NOT SURE WHAT TO DO!!***
***Occupation Class***
package Occupation; import java.util.Collection; import java.util.Objects; public class Occupation { private String COS; private String title; private int employment; private double avgSalary; public Occupation(String COS, String title, int employment, double avgSalary) { this.COS = COS; this.title = title; this.employment = employment; this.avgSalary = avgSalary; } public Occupation(String field, String field1, String field2, String field3, String field4) { } public String getCOS() { return this.COS; } public void setCOS(String COS) { this.COS = COS; } public String getTitle() { return this.title; } public void setTitle(String Title) { this.title = Title; } public int getEmployment() { return this.employment; } public void setEmployment(int employment) { this.employment = employment; } public double getAvgSalary() { return this.avgSalary; } public void setAvgSalary(double avgSalary) { this.avgSalary = avgSalary; } public Occupation COS(String COS) { this.COS = COS; return this; } public Occupation title(String title) { this.title = title; return this; } public Occupation employment(int employment) { this.employment = employment; return this; } public Occupation avgSalary(double avgSalary) { this.avgSalary = avgSalary; return this; } @Override public boolean equals(Object o) { if (o == this) return true; if (!(o instanceof Occupation)) { return false; } Occupation occupation = (Occupation) o; return Objects.equals(COS, occupation.COS) && Objects.equals(title, occupation.title) && employment == occupation.employment && avgSalary == occupation.avgSalary; } @Override public int hashCode() { return Objects.hash(COS, title, employment, avgSalary); } @Override public String toString() { return "{" + " COS='" + getCOS() + "'" + ", title='" + getTitle() + "'" + ", employment='" + getEmployment() + "'" + ", avgSalary='" + getAvgSalary() + "'" + "}"; } public Collection
***OccupationList Class***
package occupation; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.InputStreamReader; import java.util.Scanner; public class OccupationList { private Occupation[] occupations; private int size; //default constructor OccupationList(){ //creates an array of occupations occupations = new Occupation[1000]; size = 0; } //method to read from file public void readFromFile(String filename){ try{ //This allows the scanner to recieve the name of the data file as a string Scanner scanner = new Scanner(new FileInputStream(filename)); //Reads the data until the very end while (scanner.hasNext() && size < 1000) { //Recieves all of the attributes String COS = scanner.nextLine(); String title = scanner.nextLine(); String temp = scanner.nextLine(); int count; try { count = Integer.parseInt(temp.replaceAll(",", "")); } catch (NumberFormatException e) { title += " " + temp; count = Integer.parseInt(scanner.nextLine().replaceAll(",", "")); } double avgSal = Double.parseDouble(scanner.nextLine().replaceAll(",", "")); occupations[size++] = new Occupation(COS, title, count, avgSal); } //closed the file scanner.close(); //This function returns a string if the file you accessed couldn't be found or located } catch(FileNotFoundException e){ //file wasn't found System.out.println("Input file not found"); } } //get method for searching the array by the COS codes in the txt data file public Occupation search(String COS){ for(int i = 0; i < size; i++){ if(occupations[i].getCOS().equals(COS)){ return occupations[i]; } } return null; } //gets number of items in list public int size(){ return size; } //prints all occupations from data public void printAll(){ for(int i = 0; i < size; i++){ System.out.println(occupations[i]); } } //This method returns the total number of people employed public int totalPeopleEmployed(){ int noOfPeopleEmplyed = 0; for(int i = 0;i < size;i++){ noOfPeopleEmplyed+=occupations[i].getEmployment(); } return noOfPeopleEmplyed; } //This method return the average salary for all occupations public double averageSalary(){ double avgOccSalary=0.0; int totAvgSalary=0; try{ for(int i = 0;i < size;i++){ totAvgSalary+=occupations[i].getAvgSalary(); } avgOccSalary=totAvgSalary/size(); }catch (Exception e){ System.out.println("Exception Occured"); } return avgOccSalary; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
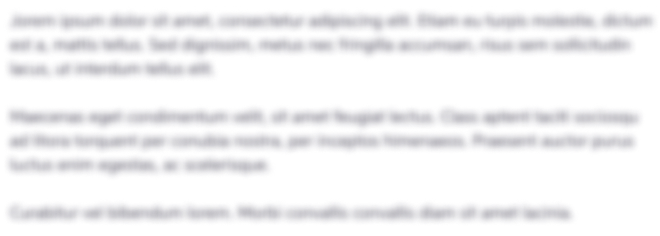
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started