Question
Your task is to implement the following methods within the DynamicArray class. template class DynamicArray { public: DynamicArray() { /* TODO: Implement me */ }
Your task is to implement the following methods within the DynamicArray class. template
Descriptions of each of the public methods are listed below: DynamicArray(): This constructor initializes the DynamicArray with a size of zero and a capacity of 8. DynamicArray(int initialSize): This constructor initializes the DynamicArray with a size of the provided initialSize and a capacity that is double the initial size. Dont worry about setting the values of the first initialSize items allow them to retain their garbage values but do consider them as items within the DynamicArray. DynamicArray(int initialSize, T defaultValue): This constructor will initialize the DynamicArray with a size of the provided initialSize and a capacity that is double the initial size. Assign each of the first initialSize items a value equal to the provided defaultValue. DynamicArray(const DynamicArray
below is the DynamicArray.h
#pragma once
#include
/*
The DynamicArray class serves as a linear container of items. Just like a
Python list, there is a first, a second, a last, and so on. This means that
the list is ordered but not necessarily sorted. The DynamicArray supports
access of items with the square bracket indexing operators [] and has common
push_back/pop_back functionality. The DynamicArray is templated and can
therefore contain any type of data.
The data is stored internally within an array allocated on the heap. As the
array fills, we dynamically allocate a new, bigger array and copy the
contents over to it.
*/
template
class DynamicArray {
private:
//////////////////////////////
// Private Member Variables //
//////////////////////////////
T* data; // The pointer to the array data
int arrSize; // Represents the number of items stored within the DA
int arrCapacity; // The size of the underlying array that holds our data
//TODO: List any additional private member variables you want here
public:
//////////////////
// Constructors //
//////////////////
/*
The default constructor allocates a new array with a capacity of 8 and the
array is to be considered empty.
*/
DynamicArray() {
// TODO: Initialize member variables
// TODO: Allocate memory for array data
data = nullptr; // FIXME: Erase this line once you allocate an address to it via new
}
/*
initialSize - The initial size (not capacity) of the DynamicArray
*/
DynamicArray(int initialSize) {
// TODO: Initialize member variables w.r.t. initialSize
// TODO: Allocate memory for array data
data = nullptr; // FIXME: Erase this line when ready to write this method
}
/*
This constructor takes in an initial size which represents the number of
items the DynamicArray will hold immediately. Note that this does not
represent the constructor, it represents actual items that are contained
within the DynamicArray. Each value in the Dynamic Array would take on the
value of the item passed in as defaultValue. To support growth potential,
we set the capacity to be double the initial size. We therefore allocate
a new array that has a capacity twice that of the initial size.
initialSize - The initial size (not capacity) of the DynamicArray
defaultValue - The value to be assigned to the first initialSize items
*/
DynamicArray(int initialSize, T defaultValue) {
// TODO: Initialize member variables w.r.t. daToCopy
// TODO: Allocate memory for array data
// TODO: Copy defaultValue into array data initialSize times
data = nullptr; // FIXME: Erase this line when ready to write this method
}
/*
This "copy constructor" takes in a reference to another DynamicArray. We
are to copy the contents of that array into this newly created object.
Therefore this constructor allocates a new array with a matching capacity
and copies the contents of the provided DynamicArray into the new local
array. Note that we treat the input array (daToCopy) as a const reference
(&) so that we confirm that the provided array will not be modified and to
gain the optimization benefit of passing by reference rather than creating
an extra, intermediate copy.
daToCopy - The provided array that we are intended to copy
*/
DynamicArray(const DynamicArray
// Initialize member variables w.r.t. daToCopy
arrSize = daToCopy.arrSize;
arrCapacity = daToCopy.arrCapacity;
// Allocate memory for array data
data = new T[arrCapacity];
// Copy contents of daToCopy into local array data
for(int i=0; i < arrSize; i++)
data[i] = daToCopy.data[i];
}
////////////////
// Destructor //
////////////////
/*
This destructor serves to de-allocate the heap-stored array so that the
operating system can reclaim and use it with future calls to "new". The
destructor is called immediately and automaticallywhen the object loses
scope.
*/
~DynamicArray() {
// Free up (delete) the heap-allocated data
if (data != nullptr)
delete[] data;
}
//////////////////////////
// Overloaded Operators //
//////////////////////////
/*
This is the "assignment" operator. It allows us to assign the contents of
one DynamicArray to another. For example, consider the following code:
DynamicArray
da1.push_back(1);
da1.push_back(2);
DynamicArray
da2.push_back(3);
da1 = da2; // This invokes the assignment operator to replace the
// original contents of da1 with a copy of the contents
// of da2
daToAssign - The DynamicArray that we want to copy onto the current DA
*/
DynamicArray
// Free up (delete) the previous heap-allocated data
delete[] data;
// Initialize member variables w.r.t. daToAssign
arrSize = daToAssign.arrSize;
arrCapacity = daToAssign.arrCapacity;
// Allocate memory for array data
data = new T[arrCapacity];
// Copy contents of daToAssign into local array data
for(int i=0; i < arrSize; i++)
data[i] = daToAssign.data[i];
// Return the current object itself. "this" is a keyword that acts as a
// pointer to the current object. By dereferencing (*) "this", we get the
// actual value of the object itself.
return *this;
}
/*
This is the "subscript" operator that allows us to easily index into and
return the items within the inner heap-allocated array. You pass in the
index and receive the value from the internal array. This works like so:
DynamicArray
da.push_back(10);
da.push_back(20);
da.push_back(30);
int middleVal = da[1];
We return a reference (return type: "T&") to the item so that we can also
change that value through assignment. Consider the following:
DynamicArray
da.push_back(10);
da.push_back(50);
da.push_back(30);
da[1] = 20;
index - An integer representing the position of the item to return
*/
T& operator[] (int index) {
return data[index];
}
/////////////////////////////////
// Additional Member Functions //
/////////////////////////////////
/*
The push_back method takes an item and adds it to the collection. This
will add it to the internal array, expanding to a larger array if there
is not enough room.
item - The item the user wishes to add to the collection.
*/
void push_back(T item) {
// TODO: Grow the array if it is full
// TODO: Add item to the "end" of the data array
}
/*
The pop_back method is invoked to inform us that the user no longer wants
the last item in the container any longer. Consider this removing the last
item in the DynamicArray.
*/
void pop_back() {
// TODO: Remove item from the "end" of the data array
}
/*
This size method returns the size of the DynamicArray. That is, the number
of items that are stored within the DynamicArray container.
*/
int size() {
// TODO: Return the size (number of items store in) the array
return 0; // <-- FIXME: Change this to reflect actual size
}
/*
The capacity method returns an integer representing the current size of
the internal heap-allocated array. This does not represent the number of
items stored within the DynamicArray container, it represents the total
number of items that can be stored within it before it is necessary to
grow the internal array again.
*/
int capacity() {
// TODO: Return the current maximum capacity of the array
return -1; // <-- FIXME: Change this to reflect actual capacity
}
/*
The clear method empties the container. If the user calls .size() after
this, then it would return zero.
*/
void clear() {
// TODO: Make the array "empty" so that it holds zero items
}
/*
The reverse method reverses the order of the contents of the DynamicArray.
Consider the physical representation below:
Before a call to reverse
Inner Array Data: [1, 2, 3, 4, 5, ?, ?, ?] <-- ? represents junk
arrSize: 5
arrCapacity: 8
After a call to reverse
Inner Array Data: [5, 4, 3, 2, 1, ?, ?, ?] <-- ? represents junk
arrSize: 5
arrCapacity: 8
*/
void reverse() {
// TODO: Reverse the contents of the array
}
private:
//////////////////////////////
// Private Member Functions //
//////////////////////////////
//TODO: Provide any private methods (helper functions) you want here.
// Note that these are NOT necessary but you can add things here
// if you think something would be useful to your implementation.
};
the ex.1.cpp
#include
#include "DynamicArray.h"
using namespace std;
int main() {
cout << "[Hello DynamicArray!]" << endl;
DynamicArray
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
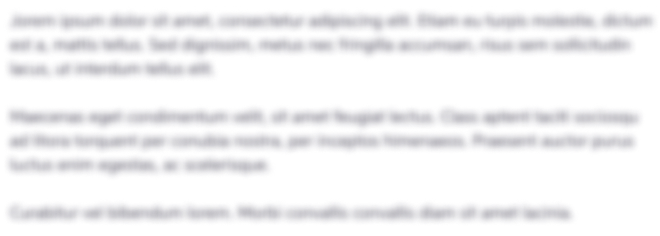
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started