Question
Your task is to write a function read_ponies(filename) that parses a file with the structure described below and returns a tuple of (elevations, path, ponies),
Your task is to write a function read_ponies(filename) that parses a file with the structure described below and returns a tuple of (elevations, path, ponies), where elevations is a 2-dimensional list with the elevation of each location. path is a list of adjacent locations from (0, 0) to (M, N), where M and N are the largest possible x and y coordinate, respectively. ponies is a list of pony tuples (described below). elevations and path are the same as in project 1, but ponies in this question are different! Each pony is represented by a tuple of (id, personality, status). In this pony tuple: id is an int in range (0, num_ponies) personality is either the string "s" or the string "a". Ponies with a personality of "s" are selfish, and ponies with an "a" personality are altruistic. status is a list in the form of [energy, location], where energy is a non-negative integer, and location is a coordinate (that is, tuple in the form of (x, y)). The list of ponies should be ordered by increasing pony id. The structure of the file is as follows: The text 'Elevations:' An arbitrary number of lines, each corresponding to a row of elevations. An arbitrary number of blank lines The text 'Path:' An arbitrary number of lines, each corresponding to a location on a single path. An arbitrary number of blank lines The text 'Ponies:' An arbitrary number of lines, each corresponding to information for a single pony. An arbitrary number of blank lines A sample file is shown below. Elevations: 0, 32, 45 2, 5, 19 7, 6, 25 Path: (0, 0) (0, 1) (1, 1) (1, 2) (2, 2) Ponies: 0, a, [30, (0, 0)] 1, s, [15, (0, 0)] 2, s, [20, (0, 0)] You may assume that the file is well-formatted .txt file, ie that there are no variations from the format described above. You can also assume that ponies in the file are listed in increasing order of pony id and that every pony has a location of (0, 0). However, there might be an arbitrary number of white spaces at the beginning of the line, end of the line, and after each comma , (see file_sample2.txt). Examples >>> result1 = read_ponies("file_sample1.txt") >>> result2 = read_ponies("file_sample2.txt") >>> result1 ([[0, 32, 45], [2, 5, 19], [7, 6, 25]], [(0, 0), (0, 1), (1, 1), (1, 2), (2, 2)], [(0, 'a', [30, (0, 0)]), (1, 's', [15, (0, 0)]), (2, 's', [20, (0, 0)])]) >>> result2 ([[0, 32, 45], [2, 5, 19], [7, 6, 25]], [(0, 0), (0, 1), (1, 1), (1, 2), (2, 2)], [(0, 'a', [30, (0, 0)]), (1, 's', [15, (0, 0)]), (2, 's', [20, (0, 0)])]) Hint You can use .readline() or .readlines() to evaluate the text file line by line . The .strip() method removes white spaces and specific characters from the beginning and end of the string. The .index() method finds the index of the first occurrence of a character. The .split() method splits a string into a list, and you can specify a seperator. The .replace() method replaces a specified phrase with another specified phrase. For example, you can use .replace() to get rid of all unwanted characters (such as "(", ")", "[", "]" etc) by replacing them with empty strings.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
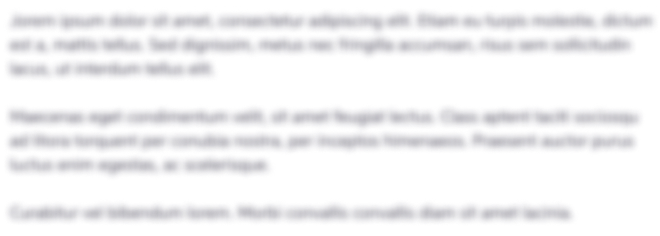
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started