Question
You're going to make an array-backed class called OscillatingResizablelist that you can add things to...but there are, of course, some wrinkles: the class will be
I've provided the initialization of the backing array because it's goofy. 13 */ 14 public OscillatingResizableList() { 15 16 listContents = (T[]) new Object[DEFAULT_CAPACITY); 17 } 18 19 /** 29 * Returns a list of the contents of the backing array listContents. 21 22
For the record, documentation in public methods should RARELY - if EVER - talk about the * internal guts of the class. :) 23 24 * 25 * @return a list of the backing array contents 26 27 public List I've provided the initialization of the backing array because it's goofy. 13 */ 14 public OscillatingResizableList() { 15 16 listContents = (T[]) new Object[DEFAULT_CAPACITY); 17 } 18 19 /** 29 * Returns a list of the contents of the backing array listContents. 21 22 For the record, documentation in public methods should RARELY - if EVER - talk about the * internal guts of the class. :) 23 24 * 25 * @return a list of the backing array contents 26 27 public List
Step by Step Solution
There are 3 Steps involved in it
Step: 1
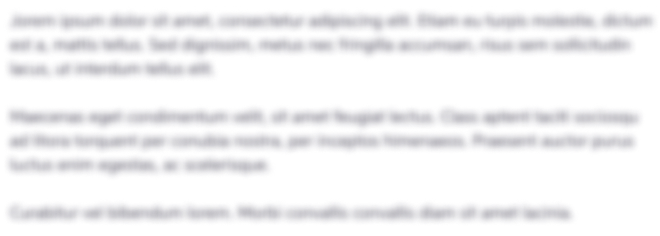
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started