Question
Zombie Attack /* Occasionally the lurching undead will find a way in and you need to hold them off. Here are the minimum requirements for
Zombie Attack
/* Occasionally the lurching undead will find a way in and you need to hold them off.
Here are the minimum requirements for the project: Start with 25 people At least one person needs to survive 10 nights to win the game if everyone dies the simulation is immediately ended. Each person has a gun and 150 rounds of ammo. There is only enough food for 8 people to survive for 10 days. Every night a random number of flesh eaters between 10 and 50 will get in. All zombies must be destroyed every night. A zombie can be killed with a gun or a blunt object. A gun has a 1 in 5 chance to hit. o If gun hits it has a 1 in 3 chance of killing instantly. o A gun must hit twice to kill if the first shot is not an instant kill. o A miss means that the shooter has a 1 in 2 chance of being killed by the zombie. A blunt object has a 1 in 3 chance of hitting. o If a blunt object hits it has a 1 in 10 chance of killing instantly. o A blunt object must hit 3 times to kill if there are no instant kill strikes. o A miss means that the person wielding the blunt object is dead you are in hand to hand combat, if you miss we assume that you are overwhelmed. People will use guns if they have ammo and they will use blunt objects when the ammo is gone.
To earn 100% on the project, do one of the following Balance the game so that a person has a chance of surviving! Provide a mechanism for people to leave to get more resources for longer survival. Make it graphical and interactive
Required programming constructs Scanner println() If/else Class for person, class for apocalypse simulator and a test class. It is important to put data and methods into the appropriate classes. Where do you think the fight method will go? Where do you think that the ammo and food should go? If it only belongs to a person it goes in the person class, if it belongs to the world it goes in the world class. Counter variables Constants Sentinel controlled loops Count controlled loops Methods with parameters Constructor Provide a toString() method I need to see clear descriptive output so that we know what is going on at each step of the program Comments that clearly explain what is going on with the code these are not necessarily good programming comments but I need to see your thought process described in the comments. I need to see well-structured code, instance data at the top of the code, constructors next, and methods after. There is no one solution to this project. You need to come up with the most elegant solution.
General plan:
There should be output summarizing the stats at the beginning of each day, similar to:
========================== You have 10 days left until salvation! There are 25 people left. You have 150 bullets. ===========================
Additional output for combat could be included: You hit a zombie. You killed a zombie. A person has died.
At the end, a summary should be provided, with at least how long that simulation survived, people died, and zombies killed:
Summary ============================ You have survived 4 days. You have killed 100 zombies. 25 people have died. =============================
A ZombieTest file should be included that can run the simulation 100000 times, and have output similar to:
============================== 100000 simulations have run. You have killed 5660066 zombies. 2499976 people have died. 5 simulations were won. 99995 simulations were lost. 3 simulations lost to starvation 0 was the lowest day reach. ===============================
The following 4 are for the GUI. Little or no modification should be needed except for ApocalypseSimulator. All text outputted should go through ApocalypseSimulator. */ // ********** ZombieFrame.java ************ package view;
import javax.swing.*; import java.awt.*; import java.nio.ByteOrder;
public class ZombieFrame extends JFrame { private JPanel inputPanel, outputPanel, titlePanel; private JTextField inputField; private JButton submitButton; private JLabel inputLabel, titleLabel; private JTextArea outputArea; private JScrollPane scroll;
public ZombieFrame(){ setLayout(new BorderLayout()); setDefaultCloseOperation(EXIT_ON_CLOSE); setUpInputs(); setUpOutput(); setUpTitle(); pack(); setVisible(true); }
public void setUpInputs(){ inputPanel=new JPanel(new FlowLayout()); inputLabel=new JLabel("Input Command: "); inputField=new JTextField(25); submitButton=new JButton("submit command");
inputPanel.add(inputLabel); inputPanel.add(inputField); inputPanel.add(submitButton);
add(inputPanel, BorderLayout.SOUTH); }
public void setUpOutput(){ outputPanel=new JPanel(new FlowLayout()); outputArea=new JTextArea(25,50); scroll=new JScrollPane(outputArea); outputPanel.add(scroll); add(outputPanel, BorderLayout.CENTER); } public void setUpTitle(){ titlePanel=new JPanel(new FlowLayout()); titleLabel=new JLabel("ZOMBIE ATTACK"); titlePanel.add(titleLabel); add(titlePanel,BorderLayout.NORTH); }
public JTextField getInputField() { return inputField; }
public void setInputField(JTextField inputField) { this.inputField = inputField; }
public JButton getSubmitButton() { return submitButton; }
public void setSubmitButton(JButton submitButton) { this.submitButton = submitButton; }
public JTextArea getOutputArea() { return outputArea; }
public void setOutputArea(JTextArea outputArea) { this.outputArea = outputArea; } }
// ************** ZombieControl.java ****************
package control;
import model.ApocalypseSimulator; import view.ZombieFrame;
import java.awt.event.ActionEvent; import java.awt.event.ActionListener;
public class ZombieControl implements ActionListener { private ZombieFrame view; private ApocalypseSimulator model;
public ZombieControl(ZombieFrame view, ApocalypseSimulator model) { this.view = view; this.model = model; view.getSubmitButton().addActionListener(this); }
@Override public void actionPerformed(ActionEvent e) { model.inputText(view.getInputField().getText()); view.getOutputArea().append(model.outputText("from control"));
} }
// ********** ApocalypseSimulator.java ****************
package model;
public class ApocalypseSimulator { // all text outputted should be through this class! private String text;
public ApocalypseSimulator(){}
public void inputText(String s){ text=s;
}
public String outputText(String s){ return s+" "+text; } }
// ********** RunGUI *****************
package tests;
import control.ZombieControl; import model.ApocalypseSimulator; import view.ZombieFrame;
public class RunGUI { public static void main(String[] args) { ZombieFrame view=new ZombieFrame(); ApocalypseSimulator model=new ApocalypseSimulator(); ZombieControl control=new ZombieControl(view, model); }
}
// ************************************ // ************************************
The following 4 need to be completed:
// ************************************ // ************************************
//**************Apocalypse.java ****************
public class Apocalypse { //instance data here. What do you need to keep track of? //number of days left?
// ie: Person p=new Person(); // may contain parameters
// include all of the stuff that is going to simulate the passing of // the day until everyone has died, or people survived for 10 days.
// Constructor. How do we want to construct? // What will we initialize through the constructor? // Number of days remaining? Number of zombies that will attack at night? Amount of ammo? // Any user interface for setting starting values
// //runSimulation() will go here ie: public boolean runSimulation(){ // return true of there are survivors. May return any type.
// examples of creating random chance: // numZombies=Utilities.generateRandomInRange(Utilities.MIN_ZOMBIE,UtilitiesMAX_ZOMBIE); //randomly generate 10 to 50 zombies // hitWithGun=Utilities.generateRandomInRange(1,5); // 1 in 5 change to hit with a gun // instantKillGun=Utilities.generateRandomInRange(1,3); // 1 in 3 of instant kill with a gun // instantKillBlunt=Utilities.generateRandomInRange(1,10); // 1 in 10 of instant kill without a gun // Do not use magic numbers! Put constants in Utilities.
}
// ********** Utilities.java *************
c class Utilities { //constants to be used in place of magic numbers public static int START_NUM_DAYS=10, START_NUM_FOOD=80; public static int MIN_ZOMBIES=10, MAX_ZOMBIE=50; public enum Status{RUN, STOP}
//static methods that will be used in Apocalypse public static int generateRandomInRange(int min, int max){ return min + (int)(Math.random() * ((max - min) + 1)); } }
// ************ Person.java *****************
c class Utilities { //constants to be used in place of magic numbers public static int START_NUM_DAYS=10, START_NUM_FOOD=80; public static int MIN_ZOMBIES=10, MAX_ZOMBIE=50; public enum Status{RUN, STOP}
//static methods that will be used in Apocalypse public static int generateRandomInRange(int min, int max){ return min + (int)(Math.random() * ((max - min) + 1)); } }
//********** Main.java *******************
public class Main {
public static void main(String[] args) { /*the only thing in the main is an Apocalypse object * and a call to runSimulation(); * there should be no program essential logic in the main. * ie:
* Apocalypse apoc=new Apocalypse(); // maybe with parameters (FOOD, DAYS) * apoc.runSimulation(); // may contain parameters */ } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
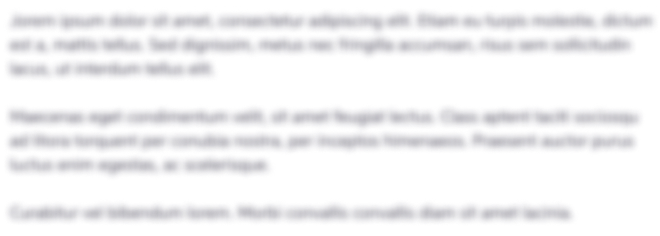
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started