Question
1. A code for merge sort is provided in this document. We are going to experimentally check the runtime for these algorithms for varying input
1. A code for merge sort is provided in this document. We are going to experimentally check the runtime for these algorithms for varying input sizes.
Input size n (size of the list):
We are going to experiment with the following list sizes
1. 10,000
2. 100,000
3. 1000,000
For each of these sizes we are going to have two types of input:
a. Sorted list (ascending order) of elements from range (1:n)
b. Sorted list (descending order) of elements from range (n:1)
#PYTHON CODE
def mergeSort(mylist):
if len(mylist)>1:
mid = len(mylist)//2
lefthalf = mylist[:mid]
righthalf = mylist[mid:]
mergeSort(lefthalf)
mergeSort(righthalf)
# Merging the lists occurs here
i=j=k=0
while i < len(lefthalf) and j < len(righthalf):
if lefthalf[i] <= righthalf[j]:
mylist[k]=lefthalf[i]
i=i+1
else:
mylist[k]=righthalf[j]
j=j+1
k=k+1
while i < len(lefthalf):
mylist[k]=lefthalf[i]
i=i+1
k=k+1
while j < len(righthalf):
mylist[k]=righthalf[j]
j=j+1
k=k+1
return mylist
Step by Step Solution
There are 3 Steps involved in it
Step: 1
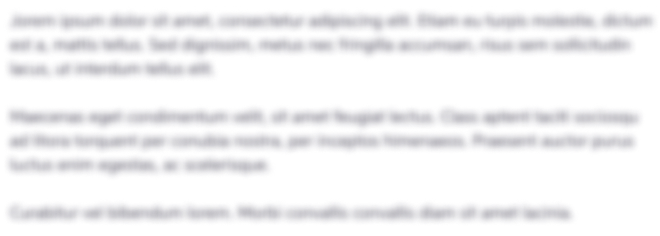
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started