Question
1) All bank accounts should have an accountId, name, and balance. 2) A Checking account should have an additional attribute for overdraftProtection which should be
1) All bank accounts should have an accountId, name, and balance.
2) A Checking account should have an additional attribute for overdraftProtection which should be type Boolean. This attribute determines whether a bank account has the overdraft protection feature enabled or not.
3) All bank accounts should prevent a deposit from happening if the amount being deposited is greater than or equal to $10,000.
4) When withdrawing from a checking account. The following constraints should be applied: i. If overdraft protection is enabled, and the withdrawal would result in a negative balance, do not allow the withdrawal to happen. PURPOSE PROBLEM REQUIREMENTS ii. If overdraft protection is not enabled, and the withdrawal would result in a negative balance, allow the withdrawal to happen and deduct an extra $50 fee.
5) When withdrawing from a savings account, do not allow the withdrawal to happen if it would result in a negative balance.
6) When adding a new bank account to the system, prompt the user to enter the specific type of bank account along with the necessary data.
7) When displaying all the accounts to the console, include the type of account in the output.
STARTING CODE:
public class Account { private int accountID; private String name; private double balance; public Account(int accountID, String name, double balance) { this.accountID = accountID; this.name = name; this.balance = balance; } public Account(int accountID, String name) { this.accountID = accountID; this.name = name; this.balance = 0.0; } public void withdraw(double amt) { this.balance -= amt; } public void deposit(double amt) { this.balance += amt; } public int getAccountID() { return accountID; } public void setAccountID(int accountID) { this.accountID = accountID; } public String getName() { return name; } public void setName(String name) { this.name = name; } public double getBalance() { return balance; } public void setBalance(double balance) { this.balance = balance; } @Override public String toString() { return accountID + "," + this.name + "," + String.format("%.2f", this.balance); } }
import java.io.File; import java.io.FileNotFoundException; import java.io.PrintWriter; import java.util.ArrayList; import java.util.Scanner; public class AccountManager { private static ArrayListaccountList = new ArrayList (); private static Scanner sc = new Scanner(System.in); public static void readAccounts() throws FileNotFoundException { File f = new File("accounts.txt"); Scanner sc = new Scanner(f); while (sc.hasNext()) { String[] values = sc.nextLine().split(","); accountList.add(new Account(Integer.parseInt(values[0]), values[1], Double.parseDouble(values[2]))); } } private static Account findAccount() { System.out.print("ID: "); int id = sc.nextInt(); sc.nextLine(); for (Account a : accountList) { if (a.getAccountID() == id) { return a; } } System.out.println(" ERROR: ID " + id + " does not exist."); return null; } private static int generateId() { int maxId = -99999; for (Account a : accountList) { if (a.getAccountID() > maxId) { maxId = a.getAccountID(); } } return maxId + 1; } public static void displayAllAccounts() { System.out.println("ID NAME BALANCE"); System.out.println("-----------------------------------------"); for (Account a : accountList) { System.out.printf("%-7d %-20s $%,.2f ", a.getAccountID(), a.getName(), a.getBalance()); } } public static void addAccount() { System.out.print("Name: "); String name = sc.nextLine(); accountList.add(new Account(generateId(), name)); System.out.println(" Successfully added a new account for " + name); } public static void removeAccount() { Account a = findAccount(); if (a != null) { accountList.remove(a); System.out.println(" Successfully removed account for " + a.getName()); } } public static void withdraw() { Account a = findAccount(); if (a != null) { System.out.print("Amount: "); double amt = sc.nextDouble(); sc.nextLine(); a.withdraw(amt); System.out.println(" Withdraw successful. New balance for " + a.getName() + " is $" + String.format("%,.2f", a.getBalance()) + " "); } } public static void deposit() { Account a = findAccount(); if (a != null) { System.out.print("Amount: "); double amt = sc.nextDouble(); sc.nextLine(); a.deposit(amt); System.out.println(" Deposit successful. New balance for " + a.getName() + " is $" + String.format("%,.2f", a.getBalance()) + " "); } } public static void writeAccounts() throws FileNotFoundException { File f = new File("accounts.txt"); PrintWriter prw = new PrintWriter(f); for (Account a : accountList) { prw.println(a); } prw.close(); } }
import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner; public class Main { public static void main(String [] args) throws FileNotFoundException { AccountManager.readAccounts(); // read all the employees from the file executeMenu(); // execute the menu AccountManager.writeAccounts(); // write all the employees back to the file } /** * repeatedly display the menu options to the user and prompt them to select one of the options. * * based on the option they select, the respective method from the EmployeeManager gets called. */ public static void executeMenu() { Scanner sc = new Scanner(System.in); String option = ""; while(!option.equalsIgnoreCase("f")) { System.out.println("A) Display all bank accounts"); System.out.println("B) Add new bank account"); System.out.println("C) Remove bank account"); System.out.println("D) Withdraw"); System.out.println("E) Deposit"); System.out.println("F) Exit"); System.out.print(" Enter an option: "); option = sc.next().toLowerCase(); System.out.println(); switch(option) { case "a": AccountManager.displayAllAccounts(); break; case "b": AccountManager.addAccount(); break; case "c": AccountManager.removeAccount(); break; case "d": AccountManager.withdraw(); break; case "e": AccountManager.deposit(); break; case "f": System.out.println("Exiting..."); break; default: System.out.println("ERROR: Please enter a number 1-4"); } System.out.println(" ----------------------------------------- "); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
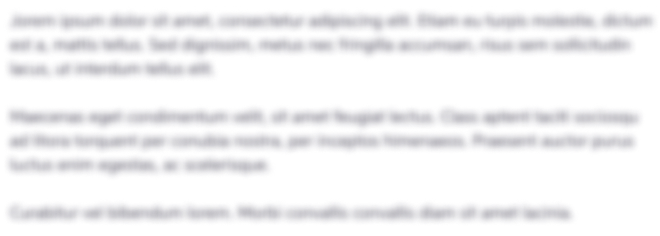
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started