Question
1. Create a new, empty project and add a package called hw1. 2. Create the Cab class in the hw1 package and put in stubs
1. Create a new, empty project and add a package called hw1.
2. Create the Cab class in the hw1 package and put in stubs for all the required methods and the constructor, as shown in the javadoc. Remember that everything listed in the javadoc is declared public. For methods that are required to return a value, just put in a "dummy" return statement that returns zero or false.
3. Download the specchecker, import it into your project as you did in labs 1 and 2, and run it. There will be lots of error messages appearing in the console output, since you haven't actually implemented the methods yet. Always start reading from the top. If you have a missing or extra public method, if the method names or declarations are incorrect, or if something is really wrong like the class having the incorrect name or package, any such errors will appear first in the output and will usually say "Class does not conform to specification." Always fix these first.
4. Now start working incrementally on functionality. The simplest operation to start with is getTotalMiles(), which just tells you how many total miles have been driven (by calls to the drive() method). You'll need to add an instance variable to keep track of this value. It should be initialized to zero in your constructor and updated in drive(). Try it out using a test class as shown in the "sample usage" section.
5. A couple of other easy ones are getMeter() and getTotalCash(). You'll need another instance variable to keep track of the amount of money on the meter, and one to keep track of the total amount of money collected in fares. Both should have initial value zero.
6. The two parameters in the constructor were not needed to implement getTotalMiles(), but they will be needed for the other methods. In order to be used in other methods, those values will have to be saved into instance variables in your constructor (kind of like the protons, neutrons, and electrons in your Atom class from Lab 2).
7. What about getCurrentRate() ? This value is initially zero, but after a call to pickUp() it should return the per-mile rate that was given in the constructor; then after a call to dropOff() it should go back to zero. This suggests that you need another instance variable, to keep track of which value to return for the current rate.
8. Next think about pickUp(). A good way to think about this is to try writing a simple test case to be sure you understand what it is actually supposed to do. For example, Cab c = new Cab(2.0, 3.0); System.out.println(c.getCurrentRate()); // what value should we get? c.pickUp(); System.out.println(c.getCurrentRate()); // what value should we get? System.out.println(c.getMeter()); // what value should we get?
9. Now, when we call drive() after pickUp(), the value on the meter should be going up. This requires adding some additional code to drive(). Cab c = new Cab(2.0, 3.0); c.pickUp(); c.drive(10); System.out.println(c.getMeter()); // what value should we get? (Note that if you already implemented getCurrentRate(), it will make this part easy, since getCurrentRate() will just be zero when the meter value isn't supposed to be changing.)
10. Next you might move on to dropOff(). Again, write some test code first, for example, //Cab c = new Cab(2.0, 3.0); c.pickUp(); c.drive(10); c.dropOff(); System.out.println(c.getCurrentRate()); // what value should we get? System.out.println(c.getMeter()); // what value should we get? System.out.println(c.getTotalCash()); // what value should we get?
JavaDoc:
public class Cab extends java.lang.Object
This class models a cab. Passengers pay to get rides. There is a base fare charged for any ride when it starts, onto which is added a per-mile rate that is charged when the cab drives. The cab has a meter showing how much is to be charged to the passenger, and it also keeps track of the total cash collected and the total miles driven since the cab was constructed. The base fare and the per-mile rate are determined by arguments to the constructor.
The amount to be charged to the passenger is always shown on the meter. The current rate is the amount by which the meter increases per mile driven. It is zero when there is no passenger, but after a passenger has been picked up the current rate is equal to the per-mile rate given in the constructor. Thus when the cab has no passenger and is just driving around, the value on the meter does not go up. Picking up a passenger is indicated by a call to pickUp(), at which time the meter is immediately set to the base fare, and the current rate is set to the actual per-mile rate. As the cab drives, the value on the meter now increases, since the current rate is nonzero.
A ride is ended by a call to dropOff(), at which point
the value on the meter is added to the total cash, and
the meter is reset to zero, and
the current rate is set to zero.
Note that if pickUp() is called, and then pickUp() is called again without the dropOff() method having been called, the previous value on the meter is lost and not added to the total cash. (It is as though a new passenger got in, and the previous passenger got out without paying.)
Here is some sample usage.
import hw1.Cab; public class SampleCabTest { public static void main(String[] args) { // constructs a new cab with a base fare of $2.00 // and a per-mile rate of $3.00 Cab t = new Cab(2, 3); // initially the total miles, total cash, current rate, // and meter should be zero System.out.println(t.getTotalMiles()); System.out.println("Expected 0"); System.out.println(t.getTotalCash()); System.out.println("Expected 0"); System.out.println(t.getCurrentRate()); System.out.println("Expected 0"); System.out.println(t.getMeter()); System.out.println("Expected 0"); System.out.println(); // after driving 5 miles, total mileage should be 5 t.drive(5); System.out.println(t.getTotalMiles()); System.out.println("Expected 5.0"); // after pickUp, the meter should be 2.00 and the current rate should be 3.00 t.pickUp(); // a passenger gets in System.out.println(t.getMeter()); System.out.println("Expected 2.0"); System.out.println(t.getCurrentRate()); System.out.println("Expected 3.0"); System.out.println(); // after driving 10 miles, the meter should be 32.00 t.drive(10); System.out.println(t.getMeter()); System.out.println("Expected 32.0"); // after dropOff, meter and current rate should be 0 t.dropOff(); System.out.println(t.getMeter()); System.out.println("Expected 0"); System.out.println(t.getCurrentRate()); System.out.println("Expected 0"); System.out.println(); // total cash collected should be 32.00 System.out.println(t.getTotalCash()); System.out.println("Expected 32.0"); // total miles should be 15 System.out.println(t.getTotalMiles()); System.out.println("Expected 15.0"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
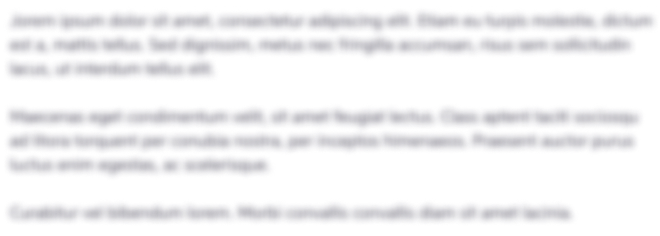
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started