Question
1. How would I change that the year of the book from the list command shows up as (1939) to [1970] menu from using ()
1. How would I change that the year of the book from the "list" command shows up as (1939) to [1970]
menu from using () to using [] for example, (1975) would be [1975]. This format should also apply to new listings using the add command when using list. Also the find command needs to work without breaking.
Python code:
def display_menu(): print("COMMAND MENU") print("list - List all movies") print("add - Add a movie") print("del - Delete a movie") print("find - Find movies by year") print("exit - Exit program") print()
#list movie details
def list(movie_list): if len(movie_list) == 0: print("There are no movies in the list. ") return else: i = 1 for movie in movie_list: row = movie print(str(i) + ". " + row[0] + " (" + str(row[1]) + ") @ " + str(row[2])) i += 1 print()
#to find a movie released in a year
def find(movie_list): year = int(input("Year: ")) #Reading the year i = 0 for movie in movie_list: #Loop through each movie details row = movie if int(row[1]) == year: print(str(row[0]) + " in "+ str(row[1])) i += 1 if i == 0: print("There are no movies released in the year " + str(year)) #display message if no movies are released in that year print()
#movie details to movie_list def add(movie_list): name = input("Name: ") #Reading the movie name year = input("Year: ") #Reading the movie released year price = input("Price: ") #Reading the price movie = [] #name year price movie.append(name) movie.append(year) movie.append(price) movie_list.append(movie) print(movie[0] + " was added. ")
#Function to delete a movie details from movie_list def delete(movie_list): number = int(input("Number: ")) if number < 1 or number > len(movie_list): print("Invalid movie number. ") else: movie = movie_list.pop(number-1) print(movie[0] + " was deleted. ")
#main def main(): movie_list = [["Monty Python and the Holy Grail", 1975, 9.95], ["On the Waterfront", 1954, 5.59], ["Cat on a Hot Tin Roof", 1958, 7.95]] display_menu() while True: command = input("Command: ") if command == "list": list(movie_list) elif command == "add": add(movie_list) elif command == "del": delete(movie_list) elif command == "find": find(movie_list) elif command == "exit": break else: print("Not a valid command. Please try again. ") print("Bye!")
if __name__ == "__main__": main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
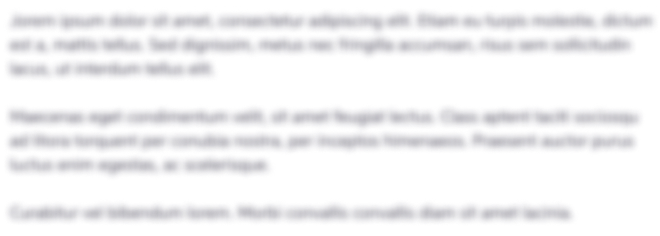
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started