Question
1. Implement the method public void createAdjectiveStory (String storyFilename, String adjectivesFilename, String outputFilename) in the StoryCreator class. The method should use the contents of a
1. Implement the method public void createAdjectiveStory (String storyFilename, String adjectivesFilename, String outputFilename) in the StoryCreator class. The method should use the contents of a file with filename storyFilename as the basis for the story. The story contains some instances of the word 'ADJECTIVE'. These should be replaced with random adjectives from the file with filename adjectivesFilename. You can find examples of two stories and an adjective file in the project folder (evening post story.txt, adjective history.txt, and adjective.txt). You can also choose to create your own files if you wish, but they must follow the same setup rules. After inserting an adjective into the story, write the contents of a file with filename outputFilename. You do not have to worry about missing line breaks or problems with encoding (strange etc). Nor do you worry about adjectives that are not completely grammatically correct, such as: "I once ate a green apple ..." But be careful not to replace parts of words such as "adjective history". 2. Implement the method public void createAdjectiveStory (String storyFilename, String outputFilename) in the StoryCreator class. Here you will also create an adjective story, but this time a user will enter adjectives through the terminal window. The user must enter adjectives until he / she is satisfied and interrupted by writing 'exit'. Then the story is written to file (as in 1). 3. Implement the method public void createAdjectiveStoryFromDictionary (String storyFilename, String dictionaryFilename, String outputFilename) in the class StoryCreator. Norway's Scrabble federation has a glossary (NSF glossary) we can use to insert random adjectives. Find the glossary (NSF glossary.txt) in the project folder and examine how the format of the file is. From the file, you must be able to extract all the adjectives, thus using random scrabble-approved adjectives in your adjective history. The method works otherwise as in (1).
import java.util.HashSet; import java.util.Scanner; import java.io.BufferedReader; import java.io.File; import java.io.FileReader; import java.io.FileNotFoundException; import java.io.FileInputStream; import java.io.InputStreamReader; import java.io.FileWriter; import java.io.IOException;
public class InputReader {
/** * Constructor for objects of class InputReader */ public InputReader() { }
/** * Return all the words in a file - BufferedReader implementation * * @param filename the name of the file * @return an arraylist of all the words in the file */ public ArrayList
try { BufferedReader in = new BufferedReader(new InputStreamReader(new FileInputStream(filename), "8859_1")); String line = in.readLine(); while(line != null) { String [] elements = line.split(" "); for(int i = 0 ; i < elements.length ; i++){ words.add(elements[i]); } line = in.readLine(); } in.close(); } catch(IOException exc) { System.out.println("Error reading words in file: " + exc); } return words; } /** * Return all the words in a file - scanner implementation * * @param filename the name of the file * @return an arraylist of all the words in the file */ public ArrayList
}
import java.util.ArrayList; import java.io.BufferedReader; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException;
public class OutputWriter {
/** * Constructor for objects of class OutputWriter */ public OutputWriter() { }
/** * Writes a list of words to a file. The words are separated by the 'space' character. * * @param output the list of words * @param filename the name of the output file */ public void write(ArrayList
} }
import java.util.Random; public class StoryCreator { private InputReader reader; private OutputWriter writer; private Random random;
public StoryCreator() { reader = new InputReader(); writer = new OutputWriter(); random = new Random(); }
public void createAdjectiveStory(String storyFilename, String adjectivesFilename, String outputFilename) { }
public void createAdjectiveStory(String storyFilename, String outputFilename) {
}
public void createAdjectiveStoryFromDictionary(String storyFilename, String dictionaryFilename, String outputFilename) {
}
}
i am completely stuck sadly.. and wondered if anyone can help, got the reader and writer compiled but cant do the stuff inbetween
Step by Step Solution
There are 3 Steps involved in it
Step: 1
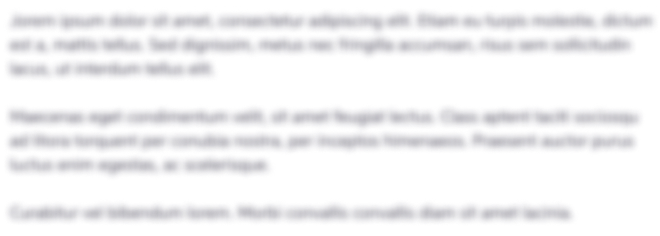
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started