Question
1. Overview: Write stubs for any new methods required in the redefined Bibliography class and the methods in the new BiblioFileIO class (view). Then rewrite
1. Overview: Write stubs for any new methods required in the redefined Bibliography class and the methods in the new BiblioFileIO class (view). Then rewrite JUnit test cases for the redefined Bibliography class and write a new JUnit test class to test the BiblioFileIOclass. As before, your JUnittest classes should include tests for the public methods of these classes.
2. Details: Remove any test methods from the earlier JUnit tests that are no longer relevant (e.g., because the methods tested no longer exist or because their functionality has changed substantially if there are any).
3. Add "modification hints" for those methods in the Bibliography class that will need to be changed, but do not change these methods yet. Enter these "hints" in the method comment block that precedes the methods. Write JUnit tests for these methods that reflects the the methods as they will be once you modify them in Stage 2. These tests should be as complete as you can make them.
****************************************************************************************************************************************************
Bibliography: This class represents the collection of publications used by your program. It will need an instance variable to represent the collection this will be built around an ArrayList of Publication objects. This program will function as a Set. It will require the standard methods for a collection (add(), get(), remove(), and size()) as well as methods for handling the standard set operations (intersection(), difference(), union(), and subset()). You may also want to pass through other useful methods from the ArrayList class (such as contains()). This class will require the following public methods:
public boolean add( Publication pub ) This method adds or inserts a Publication alphabetically into the Bibliography. This method will return true if the Publication can be added successfully. A Publication can be added successfully if its canAdd() method returns true. If the Publication cannot be added (if its canAdd() method returns false), then the method should return false.
public boolean delete( int whichOne ) This method removes from the array the Publication found at the position indicated by whichOne. If the value of whichOne is invalid, then the method should return false. If the value is valid, then the method should return true.
public Bibliography difference( Bibliography other ) This method will return a new Bibliography that contains the difference between the current Bibliography and the other Bibliography.
public Publication get( int whichOne ) This method will return the Publication object found at the position indicated by whichOne. If the value of whichOne is invalid, then the method should return null.
public Bibliography intersection( Bibliography other ) This method will return a new Bibliography that contains the intersection of the current Bibliography and the other Bibliography.
public int size() This method returns the size of the collection (the number of Publication objects currently stored).
public boolean subset( Bibliography other ) This method will return true if every item in the other Bibliography is also in the current Bibliography.
public Bibliography union( Bibliography other ) This method will return a new Bibliography that contains the union of the current Bibliography and the other Bibliography.
****************************************************************************************************************************************************
public class Bibliography { public static final int INIT_SIZE = 16; private int last; // pointer to the last slot used in the array private Publication[] bibliography; /** * Default constructor - instantiate array and initialize. */ public Bibliography() { last = -1; bibliography = new Publication[ INIT_SIZE ]; } /** * Add a publication to the collection. If successful, then increment * "last". If array is full, then expand array (private expand method). * * @param pub the Publication object to add * @return true if the addition was successful (Publication.canAdd() returns * true. */ public boolean add( Publication pub ) { boolean success = false; if ( pub.canAdd() ) { // expand if need be if ( size() == capacity() ) { expand(); } // increment last & add to array last++; bibliography[ last ] = pub; success = true; } return success; } /** * Return the capacity (size) of the array. * * @return the capacity of the array */ public int capacity() { return bibliography.length; } /** * Delete the last Publication added to the collection. Return true if * successful, false otherwise (true if something to delete). If successful, * then decrement "last". * * @return true if deletion was successful, false otherwise. */ public boolean deleteLast() { boolean success = false; if ( size() > 0 ) { bibliography[ last ] = null; last--; success = true; } return success; } /** * Retrieve the Publication object at the specified position. If position is * out of bounds or if position is not populated, return null. * * @param whichOne - the position from which to get * @return the */ public Publication get( int whichOne ) { Publication pubToReturn = null; if ( whichOne >= 0 && whichOne < size() ) { pubToReturn = bibliography[ whichOne ]; } return pubToReturn; } /** * Return the size of the collection (how many items does it hold). This * will be equal to the value "last". * * @return the value of "last". */ public int size() { return last + 1; } /** * Double the size of the array. */ private void expand() { Publication[] newBib = new Publication[ capacity() * 2 ]; for ( int i = 0; i < size(); i++ ) { newBib[ i ] = bibliography[ i ]; } bibliography = newBib; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
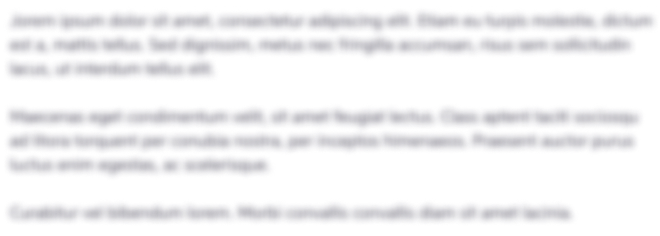
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started