Question
JAVA: How can I fix the error on line 32 or 33 of the Driver.java file? It is in bold below, and the line of
JAVA: How can I fix the error on line 32 or 33 of the Driver.java file? It is in bold below, and the line of code looks like this: String array1[] = oneLine.split(",");
// File named Driver.java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Arrays;
import java.util.Scanner;
public class Driver {
public static void main(String[] args) throws FileNotFoundException {
int row = 20;
int col = 20;
int i = 0;
String[] array = new String[col];
double[][] storeAll = new double[row][col];
USCrimeClass nb = new USCrimeClass();
File myFile = new File(args[0]);
Scanner inFile = new Scanner(myFile);
String oneLine = inFile.nextLine();
array = oneLine.split(",");
//for (String title : array) {
//System.out.println(Arrays.toString(array));
//}
//System.out.println(oneLine);
while (inFile.hasNextLine()) {
oneLine = inFile.nextLine();
String array1[] = oneLine.split(",");
nb.storeArrays(array1, i, storeAll);
i++;
}
System.out.println(Arrays.toString(array));
System.out.println(java.util.Arrays.deepToString(storeAll));
inFile.close();
}
}
// File named USCrimeClass.java
public class USCrimeClass {
int year, population, murder, rape, robbery, assault, property,
burglary, theft, motorTheft;
double violentRate, murderRate, rapeRate, robberyRate, assaultRate,
propertyRate, burglaryRate, theftRate, motorTheftRate;
public USCrimeClass() {
}
public USCrimeClass(int year, int population, int murder, int rape, int robbery, int assault, int property,
int burglary, int theft, int motorTheft, double violentRate, double murderRate, double rapeRate,
double robberyRate, double assaultRate, double propertyRate, double burglaryRate, double theftRate,
double motorTheftRate) {
this.year = year;
this.population = population;
this.murder = murder;
this.rape = rape;
this.robbery = robbery;
this.assault = assault;
this.property = property;
this.burglary = burglary;
this.theft = theft;
this.motorTheft = motorTheft;
this.violentRate = violentRate;
this.murderRate = murderRate;
this.rapeRate = rapeRate;
this.robberyRate = robberyRate;
this.assaultRate = assaultRate;
this.propertyRate = propertyRate;
this.burglaryRate = burglaryRate;
this.theftRate = theftRate;
this.motorTheftRate = motorTheftRate;
}
public double [][] storeArrays(String array1[], int i, double[][] storeAll) {
//String[][] storeAll = new String[21][20];
for(int j = 0; j < 20; j++) {
storeAll[i][j] = Double.parseDouble(array1[j]);
}
return storeAll;
}
// Getters and Setters
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public int getPopulation() {
return population;
}
public void setPopulation(int population) {
this.population = population;
}
public int getMurder() {
return murder;
}
public void setMurder(int murder) {
this.murder = murder;
}
public int getRape() {
return rape;
}
public void setRape(int rape) {
this.rape = rape;
}
public int getRobbery() {
return robbery;
}
public void setRobbery(int robbery) {
this.robbery = robbery;
}
public int getAssault() {
return assault;
}
public void setAssault(int assault) {
this.assault = assault;
}
public int getProperty() {
return property;
}
public void setProperty(int property) {
this.property = property;
}
public int getBurglary() {
return burglary;
}
public void setBurglary(int burglary) {
this.burglary = burglary;
}
public int getTheft() {
return theft;
}
public void setTheft(int theft) {
this.theft = theft;
}
public int getMotorTheft() {
return motorTheft;
}
public void setMotorTheft(int motorTheft) {
this.motorTheft = motorTheft;
}
public double getViolentRate() {
return violentRate;
}
public void setViolentRate(double violentRate) {
this.violentRate = violentRate;
}
public double getMurderRate() {
return murderRate;
}
public void setMurderRate(double murderRate) {
this.murderRate = murderRate;
}
public double getRapeRate() {
return rapeRate;
}
public void setRapeRate(double rapeRate) {
this.rapeRate = rapeRate;
}
public double getRobberyRate() {
return robberyRate;
}
public void setRobberyRate(double robberyRate) {
this.robberyRate = robberyRate;
}
public double getAssaultRate() {
return assaultRate;
}
public void setAssaultRate(double assaultRate) {
this.assaultRate = assaultRate;
}
public double getPropertyRate() {
return propertyRate;
}
public void setPropertyRate(double propertyRate) {
this.propertyRate = propertyRate;
}
public double getBurglaryRate() {
return burglaryRate;
}
public void setBurglaryRate(double burglaryRate) {
this.burglaryRate = burglaryRate;
}
public double getTheftRate() {
return theftRate;
}
public void setTheftRate(double theftRate) {
this.theftRate = theftRate;
}
public double getMotorTheftRate() {
return motorTheftRate;
}
public void setMotorTheftRate(double motorTheftRate) {
this.motorTheftRate = motorTheftRate;
}
}
// Excel File Crime.csv
Year | Population | Violent crime | Violent crime rate | Murder and nonnegligent manslaughter | Murder and nonnegligent manslaughter rate | Rape | Rape rate | Robbery | Robbery rate | Aggravated assault | Aggravated assault rate | Property crime | Property crime rate | Burglary | Burglary rate | Larceny-theft | Larceny-theft rate | Motor vehicle theft | Motor vehicle theft rate |
1994 | 2.6E+08 | 1857670 | 713.6 | 23326 | 9 | 102216 | 39.3 | 618949 | 237.8 | 1113179 | 427.6 | 12131873 | 4660.2 | 2712774 | 1042.1 | 7879812 | 3026.9 | 1539287 | 591.3 |
1995 | 2.63E+08 | 1798792 | 684.5 | 21606 | 8.2 | 97470 | 37.1 | 580509 | 220.9 | 1099207 | 418.3 | 12063935 | 4590.5 | 2593784 | 987 | 7997710 | 3043.2 | 1472441 | 560.3 |
1996 | 2.65E+08 | 1688540 | 636.6 | 19645 | 7.4 | 96252 | 36.3 | 535594 | 201.9 | 1037049 | 391 | 11805323 | 4451 | 2506400 | 945 | 7904685 | 2980.3 | 1394238 | 525.7 |
1997 | 2.68E+08 | 1636096 | 611 | 18208 | 6.8 | 96153 | 35.9 | 498534 | 186.2 | 1023201 | 382.1 | 11558475 | 4316.3 | 2460526 | 918.8 | 7743760 | 2891.8 | 1354189 | 505.7 |
1998 | 2.7E+08 | 1533887 | 567.6 | 16974 | 6.3 | 93144 | 34.5 | 447186 | 165.5 | 976583 | 361.4 | 10951827 | 4052.5 | 2332735 | 863.2 | 7376311 | 2729.5 | 1242781 | 459.9 |
1999 | 2.73E+08 | 1426044 | 523 | 15522 | 5.7 | 89411 | 32.8 | 409371 | 150.1 | 911740 | 334.3 | 10208334 | 3743.6 | 2100739 | 770.4 | 6955520 | 2550.7 | 1152075 | 422.5 |
2000 | 2.81E+08 | 1425486 | 506.5 | 15586 | 5.5 | 90178 | 32 | 408016 | 145 | 911706 | 324 | 10182584 | 3618.3 | 2050992 | 728.8 | 6971590 | 2477.3 | 1160002 | 412.2 |
2001 | 2.85E+08 | 1439480 | 504.5 | 16037 | 5.6 | 90863 | 31.8 | 423557 | 148.5 | 909023 | 318.6 | 10437189 | 3658.1 | 2116531 | 741.8 | 7092267 | 2485.7 | 1228391 | 430.5 |
2002 | 2.88E+08 | 1423677 | 494.4 | 16229 | 5.6 | 95235 | 33.1 | 420806 | 146.1 | 891407 | 309.5 | 10455277 | 3630.6 | 2151252 | 747 | 7057379 | 2450.7 | 1246646 | 432.9 |
2003 | 2.91E+08 | 1383676 | 475.8 | 16528 | 5.7 | 93883 | 32.3 | 414235 | 142.5 | 859030 | 295.4 | 10442862 | 3591.2 | 2154834 | 741 | 7026802 | 2416.5 | 1261226 | 433.7 |
2004 | 2.94E+08 | 1360088 | 463.2 | 16148 | 5.5 | 95089 | 32.4 | 401470 | 136.7 | 847381 | 288.6 | 10319386 | 3514.1 | 2144446 | 730.3 | 6937089 | 2362.3 | 1237851 | 421.5 |
2005 | 2.97E+08 | 1390745 | 469 | 16740 | 5.6 | 94347 | 31.8 | 417438 | 140.8 | 862220 | 290.8 | 10174754 | 3431.5 | 2155448 | 726.9 | 6783447 | 2287.8 | 1235859 | 416.8 |
2006 | 2.99E+08 | 1435123 | 479.3 | 17309 | 5.8 | 94472 | 31.6 | 449246 | 150 | 874096 | 292 | 10019601 | 3346.6 | 2194993 | 733.1 | 6626363 | 2213.2 | 1198245 | 400.2 |
2007 | 3.02E+08 | 1422970 | 471.8 | 17128 | 5.7 | 92160 | 30.6 | 447324 | 148.3 | 866358 | 287.2 | 9882212 | 3276.4 | 2190198 | 726.1 | 6591542 | 2185.4 | 1100472 | 364.9 |
2008 | 3.04E+08 | 1394461 | 458.6 | 16465 | 5.4 | 90750 | 29.8 | 443563 | 145.9 | 843683 | 277.5 | 9774152 | 3214.6 | 2228887 | 733 | 6586206 | 2166.1 | 959059 | 315.4 |
2009 | 3.07E+08 | 1325896 | 431.9 | 15399 | 5 | 89241 | 29.1 | 408742 | 133.1 | 812514 | 264.7 | 9337060 | 3041.3 | 2203313 | 717.7 | 6338095 | 2064.5 | 795652 | 259.2 |
2010 | 3.09E+08 | 1251248 | 404.5 | 14722 | 4.8 | 85593 | 27.7 | 369089 | 119.3 | 781844 | 252.8 | 9112625 | 2945.9 | 2168459 | 701 | 6204601 | 2005.8 | 739565 | 239.1 |
2011 | 3.12E+08 | 1206005 | 387.1 | 14661 | 4.7 | 84175 | 27 | 354746 | 113.9 | 752423 | 241.5 | 9052743 | 2905.4 | 2185140 | 701.3 | 6151095 | 1974.1 | 716508 | 230 |
2012 | 3.14E+08 | 1217057 | 387.8 | 14856 | 4.7 | 85141 | 27.1 | 355051 | 113.1 | 762009 | 242.8 | 9001992 | 2868 | 2109932 | 672.2 | 6168874 | 1965.4 | 723186 | 230.4 |
2013 | 3.16E+08 | 1163146 | 367.9 | 14196 | 4.5 | 79770 | 25.2 | 345031 | 109.1 | 724149 | 229.1 | 8632512 | 2730.7 | 1928465 | 610 | 6004453 | 1899.4 | 699594 | 221.3 |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
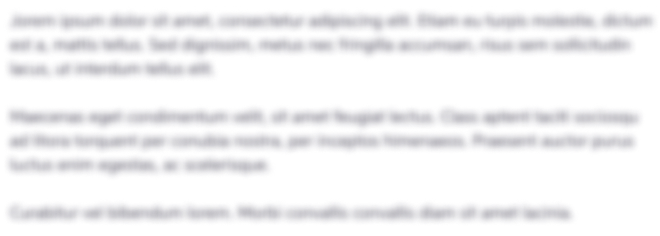
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started