Question
1. sortLargeRandomArray method of SelectSortApp class correctly modified 2. sortLargeInverseArray method of SelectSortApp class correctly modified 3. sortLargeOrderedArray method of SelectSortApp class correctly modified 4.
1. sortLargeRandomArray method of SelectSortApp class correctly modified
2. sortLargeInverseArray method of SelectSortApp class correctly modified 3. sortLargeOrderedArray method of SelectSortApp class correctly modified
4. SelectSortApp class correctly modified
Please do not copy paste some online resource
show the complete code
// selectSort.java // demonstrates selection sort // to run this program: C>java SelectSortApp //////////////////////////////////////////////////////////////// class ArraySel { private long[] a; // ref to array a private int nElems; // number of data items //-------------------------------------------------------------- public ArraySel(int max) // constructor { a = new long[max]; // create the array nElems = 0; // no items yet } //-------------------------------------------------------------- public void insert(long value) // put element into array { a[nElems] = value; // insert it nElems++; // increment size } //-------------------------------------------------------------- public void display() // displays array contents { for (int j = 0; j < nElems; j++) // for each element, System.out.print(a[j] + " "); // display it System.out.println(""); } //-------------------------------------------------------------- public long selectionSort() { long numSwaps = 0; int out, in, min; for (out = 0; out < nElems - 1; out++) // outer loop { min = out; // minimum for (in = out + 1; in < nElems; in++) // inner loop if (a[in] < a[min]) // if min greater, min = in; // we have a new min swap(out, min); // swap them numSwaps++; } // end for(out) return numSwaps; } // end selectionSort() //-------------------------------------------------------------- private void swap(int one, int two) { long temp = a[one]; a[one] = a[two]; a[two] = temp; } //-------------------------------------------------------------- } // end class ArraySel //////////////////////////////////////////////////////////////// class SelectSortApp { protected ArraySel arr; private void insertRandomData(int maxSize) { for (int j = 0; j < maxSize; j++) // fill array with { // random numbers long n = (long) (java.lang.Math.random() * (maxSize - 1)); arr.insert(n); } } protected long sortLargeRandomArray() { int numElements = 100000; arr = new ArraySel(numElements); // use insertRandomData method to create ArraySel instance with 100,000 long startTime = System.currentTimeMillis(); long numSwaps = arr.selectionSort(); long endTime = System.currentTimeMillis(); double runtime = (endTime - startTime) / 1000.0; System.out.println("Sorting 100,000 random elements completed in " + runtime + " second(s) and took " + numSwaps + " number of swaps"); return numSwaps; } protected long sortLargeInverseArray() { int numElements = 100000; arr = new ArraySel(numElements); // create a loop that populates the array with 100,000 numbers in *decreasing* order, // the opposite (inverse) direction that the sort will put then long startTime = System.currentTimeMillis(); long numSwaps = arr.selectionSort(); long endTime = System.currentTimeMillis(); double runtime = (endTime - startTime) / 1000.0; System.out.println("Sorting 100,000 elements in inverse order completed in " + runtime + " second(s) and took " + numSwaps + " number of swaps"); return numSwaps; } protected long sortLargeOrderedArray() { int numElements = 100000; arr = new ArraySel(numElements); // create a loop that populates the array with 100,000 numbers in *increasing* order, // the direction that the sort will sort them. long startTime = System.currentTimeMillis(); long numSwaps = arr.selectionSort(); long endTime = System.currentTimeMillis(); double runtime = (endTime - startTime) / 1000.0; System.out.println("Sorting 100,000 elements in correct order completed in " + runtime + " second(s) and took " + numSwaps + " number of swaps"); return numSwaps; } public static void main(String[] args) { SelectSortApp app = new SelectSortApp(); // This should require the most number of swaps, and take the most amount of time // NOTE: Because the JVM is so good at compiler optimizations, this might actually take // the least amount of time! Add -Djava.compiler=NONE to your runtime to see // how long this *really* could take! app.sortLargeInverseArray(); // This should be in the middle of the number of swaps, but given the compiler optimizations // will probably take the most amount of time app.sortLargeRandomArray(); // This should require no swaps, and will take about the same amount of time as the inverse array, // or maybe even longer! app.sortLargeOrderedArray(); } // end main() } // end class SelectSortApp ////////////////////////////////////////////////////////////////
Step by Step Solution
There are 3 Steps involved in it
Step: 1
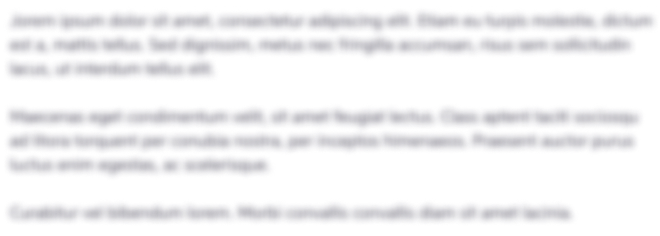
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started