Question
1. use scala Problem 1 The diabetes.csv dataset has historical data on individuals that eventually either developed diabetes or not. Diabetes is a condition where
1. use scala Problem 1 The diabetes.csv dataset has historical data on individuals that eventually either developed diabetes or not. Diabetes is a condition where the body does not produce enough insulin to break down the food that you eat. Without medication, diabetes can lead to damage to cells and vital organs and eventual death. In this problem, we want to predict whether a person is at risk of becoming diabetic based on the individuals data. This information can then be used to begin preventative measures for the individual (example lifestyle change in diet and exercise). The features and label for the dataset are described below Features or independent Variables: Pregnancies: Number of times pregnant Glucose: Plasma glucose concentration, 2 hours in an oral glucose tolerance test In-class lab BloodPressure: Diastolic blood pressure (mm Hg) SkinThickness: Triceps skin fold thickness (mm) Insulin: 2-Hour serum insulin (mu U/ml) BMI: Body mass index (weight in kg/(height in m)^2) DiabetesPedigreeFunction: Diabetes pedigree function, a score based on genetic factor of a person (diabetes has a close relation to family history). Age: Age (years) Labels or Dependent Variable: Outcome: No Diabetes = 0, Diabetes=1 in scala To solve this problem in Scala, we can use machine learning libraries such as Apache Spark MLlib or H2O.ai. Here's an example code using Apache Spark MLlib: scala Copy code // Import necessary libraries import org.apache.spark.ml.classification.{LogisticRegression, LogisticRegressionModel} import org.apache.spark.ml.evaluation.BinaryClassificationEvaluator import org.apache.spark.ml.feature.{VectorAssembler, StandardScaler} import org.apache.spark.ml.linalg.Vectors import org.apache.spark.sql.{DataFrame, SparkSession} // Create a SparkSession val spark = SparkSession.builder().appName("DiabetesPrediction").getOrCreate() // Load the dataset into a DataFrame val data = spark.read.format("csv") .option("header", "true") .option("inferSchema", "true") .load("diabetes.csv") // Create a VectorAssembler to assemble the features val assembler = new VectorAssembler() .setInputCols(Array("Pregnancies", "Glucose", "BloodPressure", "SkinThickness", "Insulin", "BMI", "DiabetesPedigreeFunction", "Age")) .setOutputCol("features") // Apply the VectorAssembler to the data val assembledData = assembler.transform(data) // Create a StandardScaler to standardize the features val scaler = new StandardScaler() .setInputCol("features") .setOutputCol("scaledFeatures") .setWithStd(true) .setWithMean(false) // Apply the StandardScaler to the assembled data val scaledData = scaler.fit(assembledData).transform(assembledData) // Split the data into training and test sets val Array(trainingData, testData) = scaledData.randomSplit(Array(0.7, 0.3), seed = 12345) // Train a Logistic Regression model val lr = new LogisticRegression() .setLabelCol("Outcome") .setFeaturesCol("scaledFeatures") .setMaxIter(100) .setRegParam(0.0) .setElasticNetParam(0.0) val lrModel = lr.fit(trainingData) // Make predictions on the test set val predictions = lrModel.transform(testData) // Evaluate the performance of the model val evaluator = new BinaryClassificationEvaluator() .setLabelCol("Outcome") .setRawPredictionCol("rawPrediction") val areaUnderROC = evaluator.evaluate(predictions) // Print the area under ROC println(s"Area under ROC = $areaUnderROC") // Save the model for future use lrModel.save("diabetesLRModel") In this code, we first load the dataset into a DataFrame and assemble the features into a vector using a VectorAssembler. We then standardize the features using a StandardScaler and split the data into training and test sets. We then train a logistic regression model on the training set and use it to make predictions on the test set. We evaluate the performance of the model using the area under the ROC curve and print it. Finally, we save the model for future use. Note that this is just one way of solving this problem and there may be other approaches that could work better depending on the specifics of the dataset and the problem at hand.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
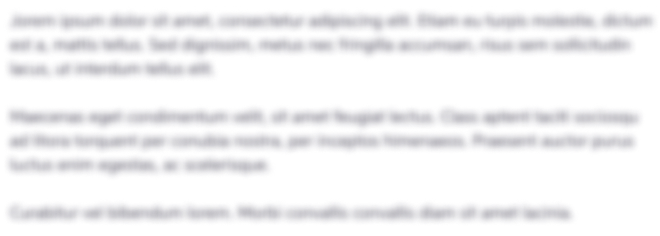
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started